How to unit test custom types in Golang?
It is crucial to unit test custom types in Golang. Methods include: 1. Using the testing package: creating a Test function and reporting errors using t.Error(); 2. Using Mocking frameworks (such as gomock and mockery) : Create a mock type for testing; 3. Use an auxiliary function: Create an auxiliary function to test the type and use it in unit testing.
How to unit test custom types in Golang
Unit testing custom types is crucial because It ensures that the type behaves as expected. There are several ways to unit test custom types in Golang.
1. Use the standard testing package
testing
package provides tools for writing and running unit tests. For custom types, you can use the Test
function to define test cases and the t.Error()
function to report errors:
package mypackage import "testing" type MyType struct { value int } func TestSum(t *testing.T) { myType := MyType{1} if myType.Sum(2) != 3 { t.Error("Expected 3, got", myType.Sum(2)) } }
2. Use Mocking Framework
The Mocking framework allows you to create custom types of mocks to test your code more easily. Popular Mocking frameworks include gomock
and mockery
:
Using gomock
:
package mypackage import ( "testing" "github.com/golang/mock/gomock" ) type MyInterface interface { DoSomething(value int) } func TestMyFunction(t *testing.T) { ctrl := gomock.NewController(t) defer ctrl.Finish() mockMyInterface := gomock.NewMockFrom(ctrl, "MyInterface") mockMyInterface.EXPECT().DoSomething(1).Return(nil) myFunction(mockMyInterface) }
3. Using Auxiliary Function
Create a helper function to test your custom types, which can help you keep your code clean. You can then use this helper function in your unit tests:
package mypackage import ( "testing" ) type MyType struct { value int } func TestSum(t *testing.T) { myType := MyType{1} if assertEqual(myType.Sum(2), 3, t) { t.Error("Expected 3, got", myType.Sum(2)) } } func assertEqual(actual, expected int, t *testing.T) bool { if actual != expected { t.Errorf("Expected %d, got %d", expected, actual) } return actual == expected }
The above is the detailed content of How to unit test custom types in Golang?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
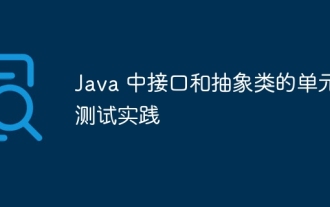
Steps for unit testing interfaces and abstract classes in Java: Create a test class for the interface. Create a mock class to implement the interface methods. Use the Mockito library to mock interface methods and write test methods. Abstract class creates a test class. Create a subclass of an abstract class. Write test methods to test the correctness of abstract classes.

PHP unit testing tool analysis: PHPUnit: suitable for large projects, provides comprehensive functionality and is easy to install, but may be verbose and slow. PHPUnitWrapper: suitable for small projects, easy to use, optimized for Lumen/Laravel, but has limited functionality, does not provide code coverage analysis, and has limited community support.
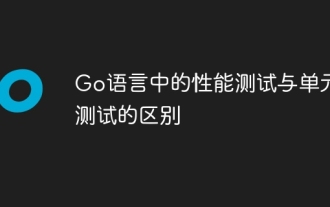
Performance tests evaluate an application's performance under different loads, while unit tests verify the correctness of a single unit of code. Performance testing focuses on measuring response time and throughput, while unit testing focuses on function output and code coverage. Performance tests simulate real-world environments with high load and concurrency, while unit tests run under low load and serial conditions. The goal of performance testing is to identify performance bottlenecks and optimize the application, while the goal of unit testing is to ensure code correctness and robustness.
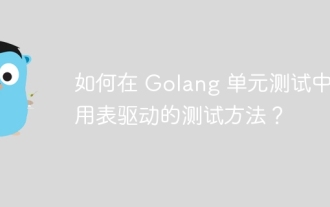
Table-driven testing simplifies test case writing in Go unit testing by defining inputs and expected outputs through tables. The syntax includes: 1. Define a slice containing the test case structure; 2. Loop through the slice and compare the results with the expected output. In the actual case, a table-driven test was performed on the function of converting string to uppercase, and gotest was used to run the test and the passing result was printed.
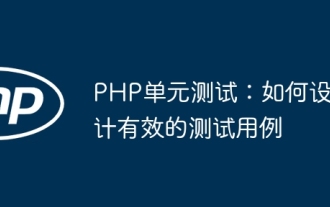
It is crucial to design effective unit test cases, adhering to the following principles: atomic, concise, repeatable and unambiguous. The steps include: determining the code to be tested, identifying test scenarios, creating assertions, and writing test methods. The practical case demonstrates the creation of test cases for the max() function, emphasizing the importance of specific test scenarios and assertions. By following these principles and steps, you can improve code quality and stability.
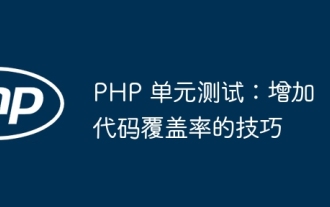
How to improve code coverage in PHP unit testing: Use PHPUnit's --coverage-html option to generate a coverage report. Use the setAccessible method to override private methods and properties. Use assertions to override Boolean conditions. Gain additional code coverage insights with code review tools.
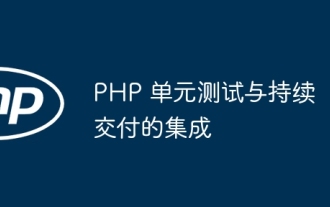
Summary: By integrating the PHPUnit unit testing framework and CI/CD pipeline, you can improve PHP code quality and accelerate software delivery. PHPUnit allows the creation of test cases to verify component functionality, and CI/CD tools such as GitLabCI and GitHubActions can automatically run these tests. Example: Validate the authentication controller with test cases to ensure the login functionality works as expected.
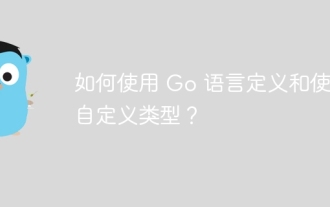
In Go, custom types can be defined using the type keyword (struct) and contain named fields. They can be accessed through field access operators and can have methods attached to manipulate instance state. In practical applications, custom types are used to organize complex data and simplify operations. For example, the student management system uses the custom type Student to store student information and provide methods for calculating average grades and attendance rates.
