


Adaptation of data access layer design and microservice architecture in Java framework
In order to implement the data access layer in the microservice architecture, you can follow the DDD principle and separate domain objects from data access logic. By adopting a service-oriented architecture, DAL can provide API services through standard protocols such as REST or gRPC, enabling reusability and observability. Taking Spring Data JPA as an example, you can create a service-oriented DAL and use JPA-compatible methods (such as findAll() and save()) to operate on data, thereby improving the scalability and flexibility of your application.
Data access layer design in Java framework and adaptation of microservice architecture
Introduction
Microservices architecture is becoming a popular approach to building modern applications. It provides greater scalability and flexibility by decomposing applications into smaller, independently deployable units based on independent services. The Data Access Layer (DAL) is critical to any application and is responsible for the application's interaction with the database. In a microservices architecture, it is crucial to design a DAL that can adapt to the needs of the microservices.
Design principles
Designing a DAL suitable for microservice architecture should follow the following principles:
- Loose coupling:DAL should be decoupled from business logic to promote ease of maintenance and reusability.
- Service Oriented: The DAL should provide service oriented APIs to facilitate interaction with other microservices.
- Scalability: DAL should be designed to scale easily as the application grows.
- Resilience: The DAL should be able to handle failures and outages and provide a failure recovery mechanism.
DDD and DAL
Domain-driven design (DDD) is a design pattern that guides the design of applications based on a domain model. DDD advocates separating domain objects from data access logic. By adopting DDD, we can design a DAL with the following advantages:
- Low coupling: Domain objects are independent of the database, improving code reusability.
- Rich semantics: DAL operations use the same language as the domain model, improving readability and maintainability.
- Portability: The domain model is portable to other platforms independent of the DAL.
Service-oriented DAL
In a microservice architecture, the DAL should provide a service-oriented API. The API allows other microservices to interact with the DAL through standard protocols such as REST or gRPC. Service-oriented DAL provides the following benefits:
- Reusability: Other microservices can leverage the services of the DAL without reinventing the wheel.
- Orchestration: Microservices can use technologies such as API gateways or service meshes to orchestrate access to DAL services.
- Observability: Centralized logging and monitoring helps monitor and troubleshoot DAL requests.
Practical Case: Using Spring Data JPA
Spring Data JPA is a popular Java framework that provides a simple abstraction. We can use Spring Data JPA to create service-oriented DAL:
@RestController @RequestMapping("/api/customers") public class CustomerController { @Autowired private CustomerRepository customerRepository; @GetMapping public List<Customer> getAllCustomers() { return customerRepository.findAll(); } @PostMapping public Customer createCustomer(@RequestBody Customer customer) { return customerRepository.save(customer); } // 其他操作... }
CustomerRepository
The interface inherits from JpaRepository
and provides ready-made methods compatible with JPA, such as findAll()
and save()
.
Conclusion
By adopting DDD principles and service-oriented architecture, we can design A data access layer to meet the needs of microservices architecture. By using a framework like Spring Data JPA, we can easily create service-oriented DALs and increase the scalability, flexibility, and reusability of our applications.
The above is the detailed content of Adaptation of data access layer design and microservice architecture in Java framework. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


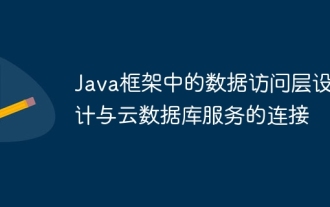
The data access layer in the Java framework is responsible for the interaction between the application and the database. To ensure reliability, DAO should follow the principles of single responsibility, loose coupling and testability. The performance and availability of Java applications can be enhanced by leveraging cloud database services such as Google Cloud SQL or Amazon RDS. Connecting to a cloud database service involves using a dedicated JDBC connector and socket factory to securely interact with the managed database. Practical cases show how to use JDBC or ORM framework to implement common CRUD operations in Java framework.
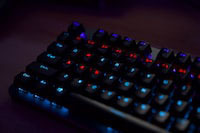
PHP microservices architecture has become a popular way to build complex applications and achieve high scalability and availability. However, adopting microservices also brings unique challenges and opportunities. This article will delve into these aspects of PHP microservices architecture to help developers make informed decisions when exploring uncharted territory. Challenging distributed system complexity: Microservices architecture decomposes applications into loosely coupled services, which increases the inherent complexity of distributed systems. For example, communication between services, failure handling, and network latency all become factors to consider. Service governance: Managing a large number of microservices requires a mechanism to discover, register, route and manage these services. This involves building and maintaining a service governance framework, which can be resource-intensive. Troubleshooting: in microservices
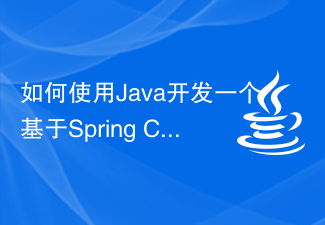
How to use Java to develop a microservice architecture based on Spring Cloud Alibaba. Microservice architecture has become one of the mainstream architectures of modern software development. It splits a complex system into multiple small, independent services, and each service can be independent Deploy, scale and manage. SpringCloudAlibaba is an open source project based on SpringCloud, providing developers with a set of tools and components to quickly build a microservice architecture. This article will introduce how
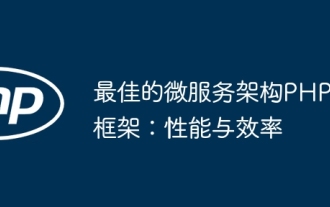
Best PHP Microservices Framework: Symfony: Flexibility, performance and scalability, providing a suite of components for building microservices. Laravel: focuses on efficiency and testability, provides a clean API interface, and supports stateless services. Slim: minimalist, fast, provides a simple routing system and optional midbody builder, suitable for building high-performance APIs.
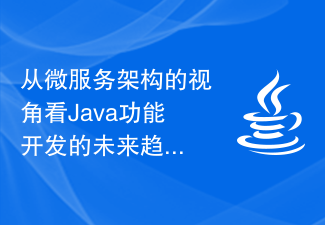
Looking at the future trends of Java function development from the perspective of microservice architecture Summary: In recent years, with the rapid development of cloud computing and big data technology, microservice architecture has become the first choice for most enterprise software development. This article will explore the future trends of Java function development from the perspective of microservice architecture, and analyze its advantages and challenges with specific code examples. Introduction With the continuous expansion of software scale and rapid changes in business, monolithic applications have gradually exposed the problem of being unable to meet modern development needs. The concept of microservice architecture is proposed to meet this challenge.
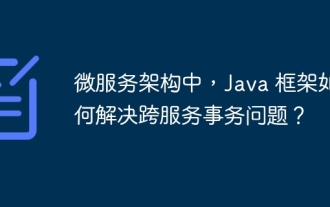
The Java framework provides distributed transaction management functions to solve cross-service transaction problems in microservice architecture, including: AtomikosTransactionsPlatform: coordinates transactions from different data sources and supports XA protocol. SpringCloudSleuth: Provides inter-service tracing capabilities and can be integrated with distributed transaction management frameworks to achieve traceability. SagaPattern: Decompose transactions into local transactions and ensure eventual consistency through the coordinator service.
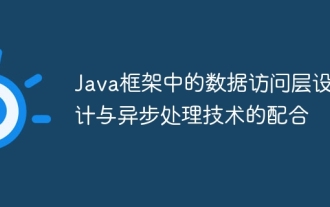
Combined with data access layer (DAO) design and asynchronous processing technology, application performance can be effectively improved in the Java framework. DAO is responsible for handling interactions with the database and follows the single responsibility principle; asynchronous processing technologies such as thread pools, CompletableFuture and ReactorPattern can avoid blocking the main thread. Combining the two, such as finding the user asynchronously via a CompletableFuture, allows the application to perform other tasks simultaneously, thus improving response times. Practical cases show the specific steps of using SpringBoot, JPA and CompletableFuture to implement an asynchronous data access layer for developers to refer to to improve application performance.
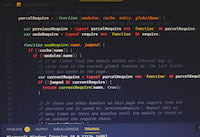
Overview of JavaActiveMQ JavaActiveMQ is an open source messaging middleware that can help enterprises easily build microservice architecture. It has the characteristics of high performance, high reliability and high scalability, and supports multiple message protocols, such as JMS, AMQP and MQtT. Features of JavaActiveMQ High performance: JavaActiveMQ is a high-performance message middleware that can process millions of messages per second. High reliability: JavaActiveMQ is a high-reliability message middleware, which can ensure reliable transmission of messages. High scalability: JavaActiveMQ is a highly scalable message middleware that can be easily expanded according to business needs.
