


C++ graphics rendering: mastering multi-threading and asynchronous technology
The performance of C graphics rendering can be significantly improved using multi-threading and asynchronous techniques: Multi-threading allows rendering tasks to be distributed to multiple threads, thereby utilizing multiple CPU cores. Asynchronous programming allows other tasks to continue while assets are loading, eliminating the delay of waiting for I/O operations. The practical example shows how to use multi-threading and asynchronous I/O to speed up scene rendering, dividing the rendering task into three parallel tasks: geometry processing, lighting calculation and texture loading.
#C Graphics Rendering: Proficient in multi-threading and asynchronous techniques
Graphics rendering involves generating a pixel matrix of an image or animation. In modern games and physically based rendering, generating these images in real time is an expensive task. By using multi-threading and asynchronous technologies, we can process rendering tasks in parallel, significantly improving performance.
Multi-threading
Multi-threading enables us to create multiple threads that run simultaneously. This way, different rendering tasks can be assigned to different threads, such as geometry processing, lighting calculations, and texture mapping. By dividing tasks, we can take full advantage of multiple CPU cores, thus speeding up the overall rendering process.
Asynchronous
Asynchronous programming technology allows us to start a task and then execute other code at the same time. This is useful for rendering tasks, as they often involve heavy I/O operations, such as loading texture and geometry data. By using asynchronous I/O, we can continue processing other tasks while the application loads assets, eliminating the delay of waiting for the I/O operation to complete.
Practical Case
Let's look at a C code example that uses multi-threading and asynchronous I/O to speed up scene rendering:
#include <thread> #include <future> #include <iostream> class Scene { public: void render() { std::packaged_task<void()> geometryTask([this] { renderGeometry(); }); std::packaged_task<void()> lightingTask([this] { computeLighting(); }); std::packaged_task<void()> textureTask([this] { loadTextures(); }); std::thread geometryThread(std::move(geometryTask)); std::thread lightingThread(std::move(lightingTask)); std::thread textureThread(std::move(textureTask)); geometryTask.get_future().wait(); lightingTask.get_future().wait(); textureTask.get_future().wait(); // 组合渲染结果 } void renderGeometry() { // 几何处理代码 } void computeLighting() { // 光照计算代码 } void loadTextures() { // 纹理加载代码 } }; int main() { Scene scene; scene.render(); return 0; }
In this In the example, the rendering of the scene is divided into three concurrent tasks: geometry processing, lighting calculation and texture loading. These tasks run in parallel, maximizing the use of your computer's processing power.
Conclusion
By leveraging multi-threading and asynchronous techniques, we can significantly improve the performance of C graphics rendering. By dividing rendering tasks and using asynchronous I/O, we can take advantage of the multi-core architecture of modern computers, resulting in a smooth, responsive interactive experience.
The above is the detailed content of C++ graphics rendering: mastering multi-threading and asynchronous technology. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


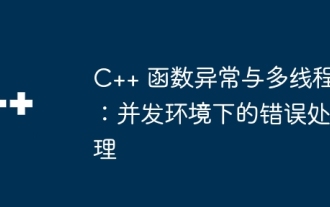
Function exception handling in C++ is particularly important for multi-threaded environments to ensure thread safety and data integrity. The try-catch statement allows you to catch and handle specific types of exceptions when they occur to prevent program crashes or data corruption.
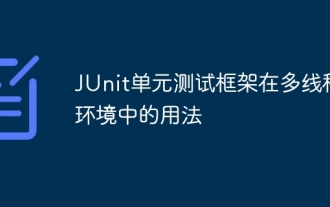
There are two common approaches when using JUnit in a multi-threaded environment: single-threaded testing and multi-threaded testing. Single-threaded tests run on the main thread to avoid concurrency issues, while multi-threaded tests run on worker threads and require a synchronized testing approach to ensure shared resources are not disturbed. Common use cases include testing multi-thread-safe methods, such as using ConcurrentHashMap to store key-value pairs, and concurrent threads to operate on the key-value pairs and verify their correctness, reflecting the application of JUnit in a multi-threaded environment.
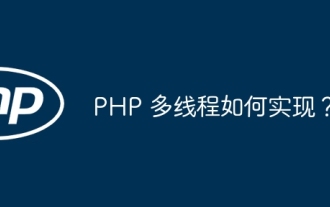
PHP multithreading refers to running multiple tasks simultaneously in one process, which is achieved by creating independently running threads. You can use the Pthreads extension in PHP to simulate multi-threading behavior. After installation, you can use the Thread class to create and start threads. For example, when processing a large amount of data, the data can be divided into multiple blocks and a corresponding number of threads can be created for simultaneous processing to improve efficiency.
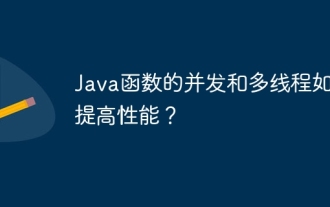
Concurrency and multithreading techniques using Java functions can improve application performance, including the following steps: Understand concurrency and multithreading concepts. Leverage Java's concurrency and multi-threading libraries such as ExecutorService and Callable. Practice cases such as multi-threaded matrix multiplication to greatly shorten execution time. Enjoy the advantages of increased application response speed and optimized processing efficiency brought by concurrency and multi-threading.
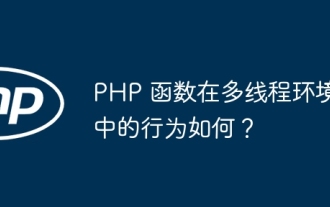
In a multi-threaded environment, the behavior of PHP functions depends on their type: Normal functions: thread-safe, can be executed concurrently. Functions that modify global variables: unsafe, need to use synchronization mechanism. File operation function: unsafe, need to use synchronization mechanism to coordinate access. Database operation function: Unsafe, database system mechanism needs to be used to prevent conflicts.
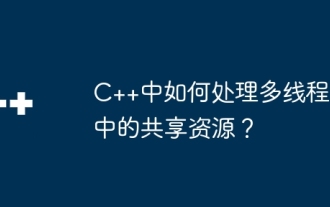
Mutexes are used in C++ to handle multi-threaded shared resources: create mutexes through std::mutex. Use mtx.lock() to obtain a mutex and provide exclusive access to shared resources. Use mtx.unlock() to release the mutex.
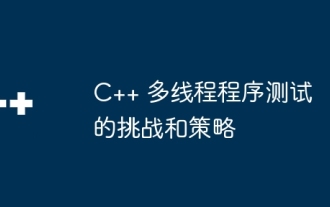
Multi-threaded program testing faces challenges such as non-repeatability, concurrency errors, deadlocks, and lack of visibility. Strategies include: Unit testing: Write unit tests for each thread to verify thread behavior. Multi-threaded simulation: Use a simulation framework to test your program with control over thread scheduling. Data race detection: Use tools to find potential data races, such as valgrind. Debugging: Use a debugger (such as gdb) to examine the runtime program status and find the source of the data race.
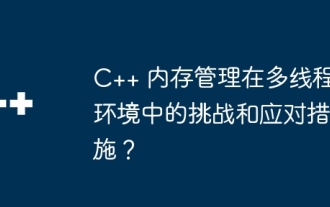
In a multi-threaded environment, C++ memory management faces the following challenges: data races, deadlocks, and memory leaks. Countermeasures include: 1. Use synchronization mechanisms, such as mutexes and atomic variables; 2. Use lock-free data structures; 3. Use smart pointers; 4. (Optional) implement garbage collection.
