How to select specific column from database in Golang?
Using the xorm library in Golang, you can easily query specific columns from the database: import the xorm library and initialize the database connection. Construct a Session for interacting with the database. Use the Cols method to specify the columns to select. Call the Find method to execute the query and obtain the results.
#How to select specific columns from database in Golang?
In Golang, you can easily query specific columns from the database using the xorm
library. xorm
is a Go ORM framework that allows you to interact with databases in an intuitive way.
Steps:
- Import the
xorm
library and initialize a database connection. - Construct a
Session
for interacting with the database. - Use the
Cols
method to specify the columns to select. - Call the
Find
method to execute the query and obtain the results.
Code example:
package main import ( "fmt" "github.com/go-xorm/xorm" ) type User struct { Id int `xorm:"pk autoincr"` Name string `xorm:"varchar(50)"` Email string `xorm:"varchar(50)"` Password string `xorm:"varchar(255)"` } func main() { // 1. 初始化数据库连接 engine, err := xorm.NewEngine("mysql", "user:password@/db_name") if err != nil { fmt.Println(err) return } defer engine.Close() // 2. 构建一个 Session session := engine.NewSession() // 3. 指定要选择的列 session.Cols("Id", "Name") // 4. 执行查询并获取结果 users := []User{} if err = session.Find(&users); err != nil { fmt.Println(err) return } // 5. 遍历结果并打印 for _, user := range users { fmt.Println(user.Id, user.Name) } }
Output:
1 John 2 Mary 3 Bob
This example demonstrates how to use xorm
Select specific columns from the database, namely Id
and Name
.
The above is the detailed content of How to select specific column from database in Golang?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
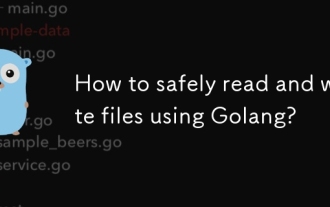
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
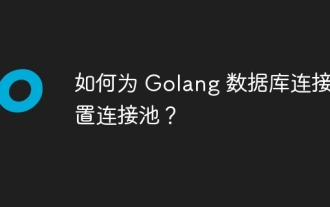
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
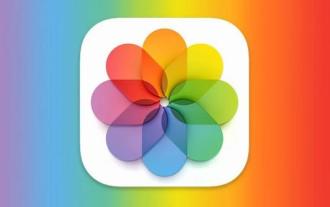
Apple's latest releases of iOS18, iPadOS18 and macOS Sequoia systems have added an important feature to the Photos application, designed to help users easily recover photos and videos lost or damaged due to various reasons. The new feature introduces an album called "Recovered" in the Tools section of the Photos app that will automatically appear when a user has pictures or videos on their device that are not part of their photo library. The emergence of the "Recovered" album provides a solution for photos and videos lost due to database corruption, the camera application not saving to the photo library correctly, or a third-party application managing the photo library. Users only need a few simple steps
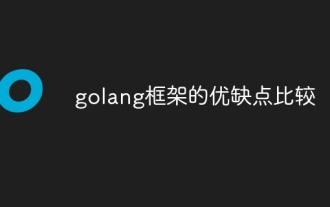
The Go framework stands out due to its high performance and concurrency advantages, but it also has some disadvantages, such as being relatively new, having a small developer ecosystem, and lacking some features. Additionally, rapid changes and learning curves can vary from framework to framework. The Gin framework is a popular choice for building RESTful APIs due to its efficient routing, built-in JSON support, and powerful error handling.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
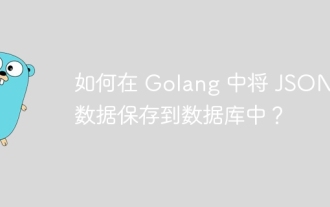
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
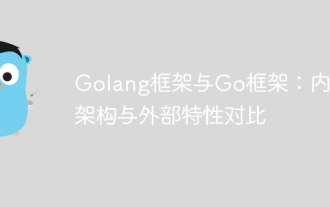
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
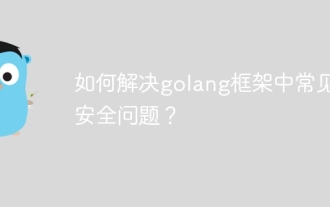
How to address common security issues in the Go framework With the widespread adoption of the Go framework in web development, ensuring its security is crucial. The following is a practical guide to solving common security problems, with sample code: 1. SQL Injection Use prepared statements or parameterized queries to prevent SQL injection attacks. For example: constquery="SELECT*FROMusersWHEREusername=?"stmt,err:=db.Prepare(query)iferr!=nil{//Handleerror}err=stmt.QueryR
