


How do the architectural features of the golang framework affect application performance?
The underlying architecture of the Go framework significantly affects application performance. These features include: Concurrency: Goroutines allow multiple requests to be processed simultaneously, improving throughput. Memory management: The garbage collection mechanism automatically releases unused memory to reduce consumption. Response time: Built-in types limit the number of goroutines processing requests to prevent resource overuse.
How the Go framework architecture affects application performance
The Go framework provides a powerful foundation for building high-performance web applications. The underlying architectural features of these frameworks have a significant impact on the overall performance of the application.
Concurrency:
The Go framework is based on a concurrency model that enables applications to handle multiple requests simultaneously. Goroutine lightweight threads allow multiple tasks to run in parallel, increasing throughput and reducing latency.
Practical case:
Using Gee, a lightweight Go Web framework, a simple HTTP server was built:
package main import ( "fmt" "net/http" ) func main() { http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) { fmt.Fprintf(w, "Hello, world!") }) http.ListenAndServe(":8080", nil) }
This server Requests from multiple clients can be processed simultaneously because a goroutine is created for each request.
Memory management:
The Go framework uses a garbage collection mechanism to manage memory. This eliminates the need for manual memory management, increases developer productivity, and reduces performance issues related to memory leaks.
Practical case:
Using Echo, a high-performance Go Web framework, to create a simple REST API:
package main import ( "fmt" "net/http" "github.com/labstack/echo/v4" ) func main() { e := echo.New() e.GET("/", func(c echo.Context) error { return c.String(http.StatusOK, "Hello, world!") }) e.Logger.Fatal(e.Start(":8080")) }
Due to the garbage collection mechanism , the framework will automatically release unused memory, reducing memory consumption and improving overall performance.
Response time:
The Go framework emphasizes response time and reduces latency by optimizing the HTTP processing pipeline. Built-in types like web.MaxHandler limit the number of goroutines that can handle a single request, preventing resource overcommitment.
Practical case:
Create a fast blogging application using Gin, a Go framework for building modern Web APIs:
package main import ( "fmt" "net/http" "github.com/gin-gonic/gin" ) func main() { r := gin.Default() r.GET("/", func(c *gin.Context) { c.String(http.StatusOK, "Welcome to my blog!") }) r.POST("/article", func(c *gin.Context) { // 处理创建文章的请求 }) r.Run(":8080") }
Thanks to Gin's response time optimization, blogging applications can handle large numbers of concurrent requests and always provide fast responses.
The above is the detailed content of How do the architectural features of the golang framework affect application performance?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


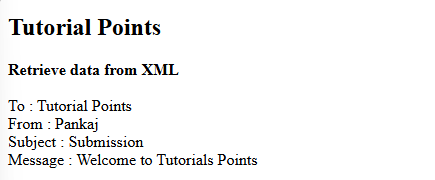
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
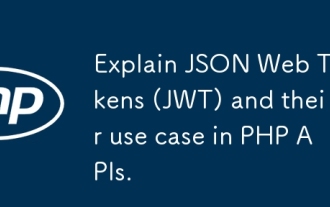
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
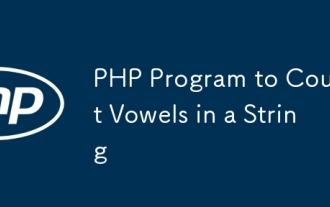
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
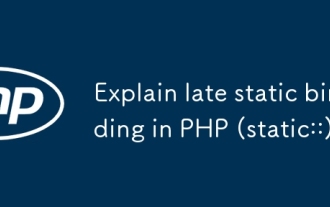
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
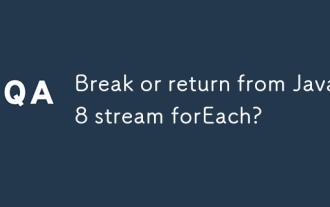
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
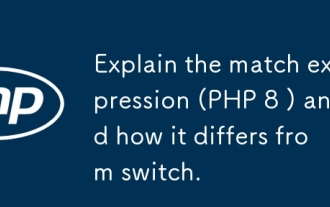
In PHP8, match expressions are a new control structure that returns different results based on the value of the expression. 1) It is similar to a switch statement, but returns a value instead of an execution statement block. 2) The match expression is strictly compared (===), which improves security. 3) It avoids possible break omissions in switch statements and enhances the simplicity and readability of the code.
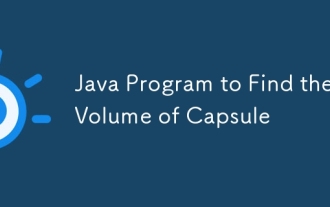
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
