PHP Unit Testing: How to Design Effective Test Cases
It is crucial to design effective unit test cases, following the following principles: atomicity, simplicity, repeatability and clarity. The steps include: determining the code to be tested, identifying test scenarios, creating assertions, and writing test methods. The practical case demonstrates the creation of test cases for the max() function, emphasizing the importance of specific test scenarios and assertions. By following these principles and steps, you can improve code quality and stability.
PHP Unit Testing: Designing Effective Test Cases
Unit testing is a vital software development practice that passes Verify the correctness of code snippets to improve code quality. Designing effective test cases is critical to ensuring the reliability and timeliness of testing.
Principles of efficient test case design
- Atomicity: Test cases should be independent of other test cases.
- Conciseness: Test cases should be short and easy to understand.
- Repeatable: Test cases should produce the same results every time they are executed.
- Clarity: Test cases should clearly state the expected behavior of the test.
Steps in designing test cases
- #Determine the code segment to be tested:Clear the code function you want to test or method.
- Identify test scenarios: Consider all possible data inputs and outputs to cover various scenarios.
- Create assertions: Write assertions to verify expected code behavior.
- Writing test methods: Encapsulate test scenarios and assertions in a test method.
Practical case
Consider a simple function that calculates the maximum value:
function max($a, $b) { if ($a > $b) { return $a; } else { return $b; } }
Test case:
class MaxTest extends PHPUnit_Framework_TestCase { public function testMax() { // 测试场景 1:a > b $a = 5; $b = 3; $expected = 5; $actual = max($a, $b); $this->assertEquals($expected, $actual); // 测试场景 2:a < b $a = 3; $b = 5; $expected = 5; $actual = max($a, $b); $this->assertEquals($expected, $actual); // 测试场景 3:a = b $a = 5; $b = 5; $expected = 5; $actual = max($a, $b); $this->assertEquals($expected, $actual); } }
In this example, we have created three test methods for different test scenarios. Each test method contains an assertion that verifies the expected behavior of the function.
Conclusion
By following good design principles and using practical examples, you can create effective and reliable unit tests. This will improve the quality of your code base and provide greater stability to your application.
The above is the detailed content of PHP Unit Testing: How to Design Effective Test Cases. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
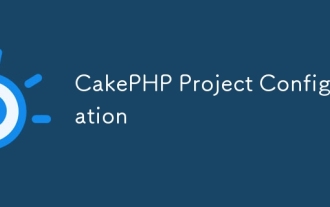
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
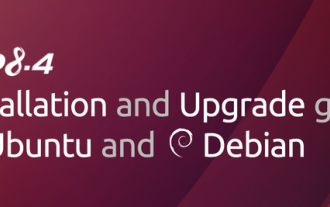
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
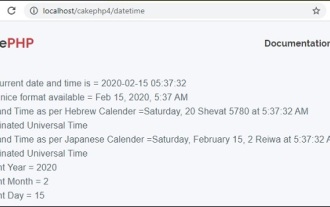
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
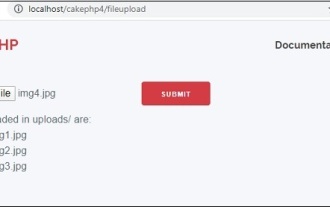
To work on file upload we are going to use the form helper. Here, is an example for file upload.
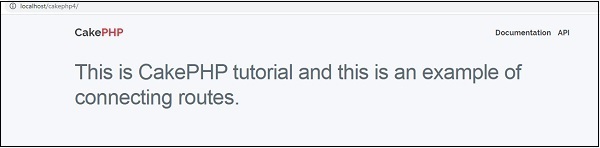
In this chapter, we are going to learn the following topics related to routing ?
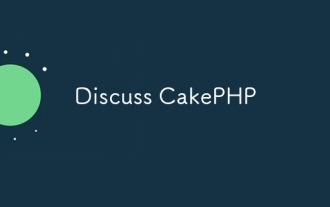
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
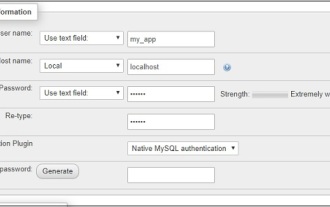
Working with database in CakePHP is very easy. We will understand the CRUD (Create, Read, Update, Delete) operations in this chapter.
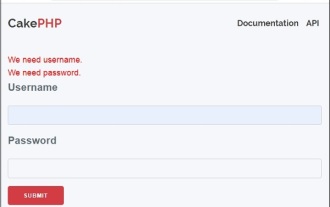
Validator can be created by adding the following two lines in the controller.
