Revealing the pros and cons of java framework
Java frameworks provide advantages that simplify development, including rapid development, maintainability, scalability, and security. But they also have drawbacks, such as limited flexibility, performance overhead, coupling, and learning curves. For example, the Spring framework simplifies user data management, making code cleaner and easier to maintain.
Pros and Cons of Java Framework
Java framework is a set of reusable components and libraries designed to simplify Java applications Program development. They provide core functionality such as data access, network connectivity, and authentication, allowing developers to focus on creating the business logic of the application.
Advantages:
- Rapid development: The framework provides out-of-the-box functionality, thereby reducing development time.
- Maintainability: The framework follows specific design patterns to make the application easier to maintain.
- Extensibility: The framework provides pluggable components that allow the functionality of the application to be easily extended.
- Security: Most frameworks have built-in security features such as authentication and authorization.
- Community Support: Popular frameworks have large communities that provide support, documentation, and examples.
Disadvantages:
- Limited Flexibility: The framework imposes specific architectural and design decisions that may Limit flexibility.
- Performance Overhead: Some frameworks may introduce performance overhead, especially when the application does not require all of its functionality.
- Coupling: Using a framework couples the application to that framework, making it difficult to switch frameworks in the future.
- Learning Curve: Understanding and using frameworks requires a learning curve, especially for complex frameworks.
Practical case:
Consider the following Java application that needs to manage user data:
public class App { public static void main(String[] args) { // 初始化数据库连接 Database db = new Database(); // 创建用户 User user = new User(); user.setUsername("admin"); user.setPassword("password"); db.createUser(user); // 获取用户列表 List<User> users = db.getUsers(); // 打印用户列表 for (User u : users) { System.out.println(u.getUsername()); } } }
Using the Spring framework, this code can be simplified :
public class App { @Autowired private UserService userService; public static void main(String[] args) { // 初始化 Spring 容器 SpringApplication.run(App.class, args); // 创建用户 User user = new User(); user.setUsername("admin"); user.setPassword("password"); userService.createUser(user); // 获取用户列表 List<User> users = userService.getUsers(); // 打印用户列表 for (User u : users) { System.out.println(u.getUsername()); } } }
In this example, the Spring framework handles the complexity of database connections and user management, making the code cleaner and easier to maintain.
The above is the detailed content of Revealing the pros and cons of java framework. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


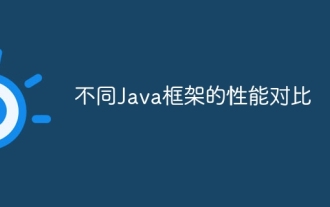
Performance comparison of different Java frameworks: REST API request processing: Vert.x is the best, with a request rate of 2 times SpringBoot and 3 times Dropwizard. Database query: SpringBoot's HibernateORM is better than Vert.x and Dropwizard's ORM. Caching operations: Vert.x's Hazelcast client is superior to SpringBoot and Dropwizard's caching mechanisms. Suitable framework: Choose according to application requirements. Vert.x is suitable for high-performance web services, SpringBoot is suitable for data-intensive applications, and Dropwizard is suitable for microservice architecture.
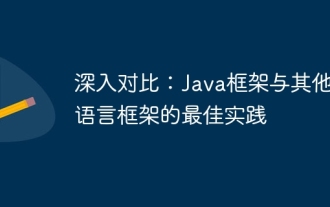
Java frameworks are suitable for projects where cross-platform, stability and scalability are crucial. For Java projects, Spring Framework is used for dependency injection and aspect-oriented programming, and best practices include using SpringBean and SpringBeanFactory. Hibernate is used for object-relational mapping, and best practice is to use HQL for complex queries. JakartaEE is used for enterprise application development, and the best practice is to use EJB for distributed business logic.
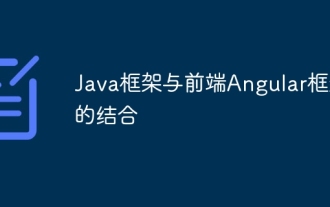
Answer: Java backend framework and Angular frontend framework can be integrated to provide a powerful combination for building modern web applications. Steps: Create Java backend project, select SpringWeb and SpringDataJPA dependencies. Define model and repository interfaces. Create a REST controller and provide endpoints. Create an Angular project. Add SpringBootJava dependency. Configure CORS. Integrate Angular in Angular components.
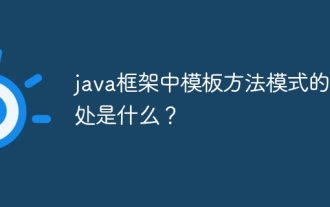
The Template Method pattern defines an algorithm framework with specific steps implemented by subclasses. Its advantages include extensibility, code reuse, and consistency. In a practical case, the beverage production framework uses this pattern to create customizable beverage production algorithms, including coffee and tea classes, which can customize brewing and flavoring steps while maintaining consistency.
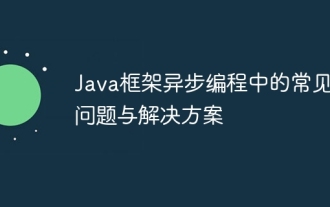
3 common problems and solutions in asynchronous programming in Java frameworks: Callback Hell: Use Promise or CompletableFuture to manage callbacks in a more intuitive style. Resource contention: Use synchronization primitives (such as locks) to protect shared resources, and consider using thread-safe collections (such as ConcurrentHashMap). Unhandled exceptions: Explicitly handle exceptions in tasks and use an exception handling framework (such as CompletableFuture.exceptionally()) to handle exceptions.
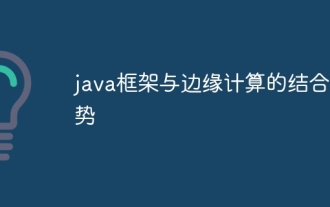
Java frameworks are combined with edge computing to enable innovative applications. They create new opportunities for the Internet of Things, smart cities and other fields by reducing latency, improving data security, and optimizing costs. The main integration steps include selecting an edge computing platform, deploying Java applications, managing edge devices, and cloud integration. Benefits of this combination include reduced latency, data localization, cost optimization, scalability and resiliency.
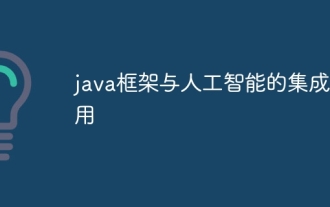
Java frameworks integrated with AI enable applications to take advantage of AI technologies, including automating tasks, delivering personalized experiences, and supporting decision-making. By directly calling or using third-party libraries, the Java framework can be seamlessly integrated with frameworks such as H2O.ai and Weka to achieve functions such as data analysis, predictive modeling, and neural network training, and be used for practical applications such as personalized product recommendations.
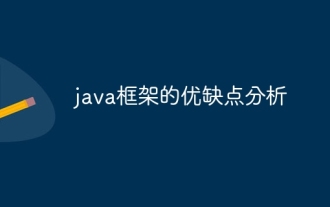
The Java framework provides predefined components with the following advantages and disadvantages: Advantages: code reusability, modularity, testability, security, and versatility. Disadvantages: Learning curve, performance overhead, limitations, complexity, and vendor lock-in.
