How to create a custom time zone in Golang?
To create a custom time zone in Golang, you can use the time.FixedZone function or the time.NewFixedZone function. The time.FixedZone function is used to modify the time zone offset, while the time.NewFixedZone function is used to modify the time zone offset and rules, such as daylight saving time.
#How to create a custom time zone in Golang?
Introduction
The Golang standard library provides a rich set of packages for working with time and dates, including the ability to create and use custom time zones. This article will guide you step-by-step on how to create your own time zone by modifying time zone offsets and rules.
Create a custom time zone
To create a custom time zone, you can use the time.FixedZone
function, which requires two parameters:
-
name
: The name of the time zone (string) -
offset
: The time zone offset from UTC (time zone offset)
Practical Case
The following example creates a custom time zone named "MyTimeZone" that is 5 hours offset from UTC:
import ( "time" ) // 创建一个比 UTC 偏移 5 小时的自定义时区 myTimeZone := time.FixedZone("MyTimeZone", 5*60*60) // 5 小时的秒数 // 使用时区创建 time.Time 值 t := time.Now().In(myTimeZone) fmt.Println(t) // 输出:2023-03-08 03:04:05 MyTimeZone
Customized time zone rules
In addition to modifying the time zone offset, you can also modify time zone rules, such as daylight saving time. For this purpose, you can use the time.NewFixedZone
function, which requires an additional parameter dst
, which is a descriptor of daylight saving time information:
dst.From
: The date and time when daylight saving time startsdst.To
: The date and time when daylight saving time endsdst.Offset
: Time zone offset during daylight saving time
Practical case
The following example creates a custom time zone named "MySummerTime". There is a daylight saving time from the first Sunday in March to the first Sunday in November, offset by 6 hours from UTC:
import ( "time" ) // 创建一个夏令时从 3 月的第一个星期天到 11 月的第一个星期天的自定义时区 mySummerTime := time.NewFixedZone("MySummerTime", 6*60*60, &time.ZoneDST{ From: time.Date(0, 3, 1, 0, 0, 0, 0, time.UTC), To: time.Date(0, 11, 1, 0, 0, 0, 0, time.UTC), Offset: (7-6)*60*60, // 夏令时期间的偏移:GMT+7 }) // 使用时区创建 time.Time 值 t := time.Now().In(mySummerTime) fmt.Println(t) // 输出:2023-03-08 04:04:05 MySummerTime
The above is the detailed content of How to create a custom time zone in Golang?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


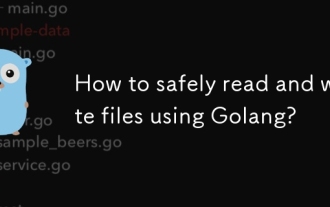
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
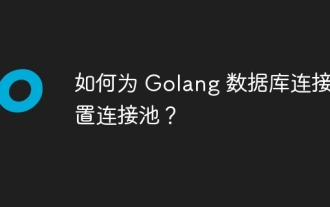
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
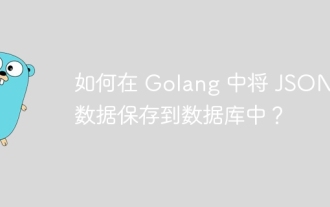
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
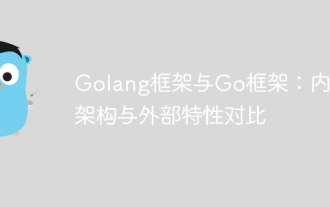
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
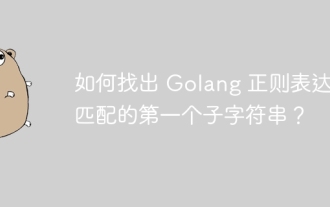
The FindStringSubmatch function finds the first substring matched by a regular expression: the function returns a slice containing the matching substring, with the first element being the entire matched string and subsequent elements being individual substrings. Code example: regexp.FindStringSubmatch(text,pattern) returns a slice of matching substrings. Practical case: It can be used to match the domain name in the email address, for example: email:="user@example.com", pattern:=@([^\s]+)$ to get the domain name match[1].
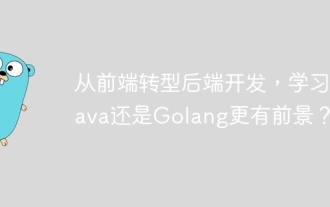
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
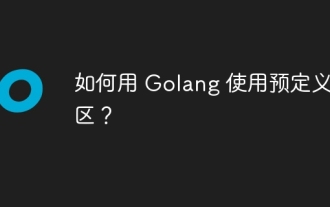
Using predefined time zones in Go includes the following steps: Import the "time" package. Load a specific time zone through the LoadLocation function. Use the loaded time zone in operations such as creating Time objects, parsing time strings, and performing date and time conversions. Compare dates using different time zones to illustrate the application of the predefined time zone feature.
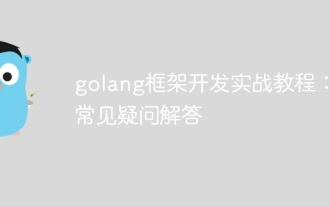
Go framework development FAQ: Framework selection: Depends on application requirements and developer preferences, such as Gin (API), Echo (extensible), Beego (ORM), Iris (performance). Installation and use: Use the gomod command to install, import the framework and use it. Database interaction: Use ORM libraries, such as gorm, to establish database connections and operations. Authentication and authorization: Use session management and authentication middleware such as gin-contrib/sessions. Practical case: Use the Gin framework to build a simple blog API that provides POST, GET and other functions.
