How to use C++ for natural language processing and text analysis?
Natural language processing with C++ involves installing the Boost.Regex, ICU and pugixml libraries. The article details the creation of a stemmer, which reduces words to their root words, and a bag-of-words model, which represents text as word frequency vectors. Demonstrates the use of word segmentation, stemming, and bag-of-word models to analyze text and output the segmented words, word stems, and word frequencies.
Using C++ for natural language processing and text analysis
Natural language processing (NLP) is a field that uses computers to process, analyze and generate human language. The discipline of the task. This article explains how to use the C++ programming language for NLP and text analysis.
Install the necessary libraries
You need to install the following libraries:
- Boost.Regex
- ICU for C++
- pugixml
The command to install these libraries on Ubuntu is as follows:
sudo apt install libboost-regex-dev libicu-dev libpugixml-dev
Create a stemmer
A stemmer is used to reduce words to their root words.
#include <boost/algorithm/string/replace.hpp> #include <iostream> #include <map> std::map<std::string, std::string> stemmer_map = { {"ing", ""}, {"ed", ""}, {"es", ""}, {"s", ""} }; std::string stem(const std::string& word) { std::string stemmed_word = word; for (auto& rule : stemmer_map) { boost::replace_all(stemmed_word, rule.first, rule.second); } return stemmed_word; }
Create a bag-of-words model
The bag-of-words model is a model that represents text as a word frequency vector.
#include <map> #include <string> #include <vector> std::map<std::string, int> create_bag_of_words(const std::vector<std::string>& tokens) { std::map<std::string, int> bag_of_words; for (const auto& token : tokens) { std::string stemmed_token = stem(token); bag_of_words[stemmed_token]++; } return bag_of_words; }
Practical case
The following is a demonstration of text analysis using the above code:
#include <iostream> #include <vector> std::vector<std::string> tokenize(const std::string& text) { // 将文本按空格和句点分词 std::vector<std::string> tokens; std::istringstream iss(text); std::string token; while (iss >> token) { tokens.push_back(token); } return tokens; } int main() { std::string text = "Natural language processing is a subfield of linguistics, computer science, information engineering, and artificial intelligence concerned with the interactions between computers and human (natural) languages."; // 分词并词干化 std::vector<std::string> tokens = tokenize(text); for (auto& token : tokens) { std::cout << stem(token) << " "; } std::cout << std::endl; // 创建词袋模型 std::map<std::string, int> bag_of_words = create_bag_of_words(tokens); for (const auto& [word, count] : bag_of_words) { std::cout << word << ": " << count << std::endl; } }
Output:
nat lang process subfield linguist comput sci inf engin artifi intell concern interact comput hum nat lang nat: 1 lang: 2 process: 1 subfield: 1 linguist: 1 comput: 1 sci: 1 inf: 1 engin: 1 artifi: 1 intell: 1 concern: 1 interact: 1 hum: 1
The above is the detailed content of How to use C++ for natural language processing and text analysis?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
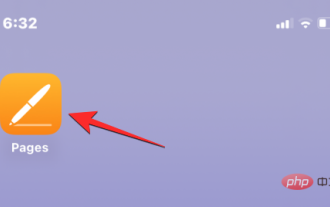
Color helps how we process things visually, so using a variety of colors in documents, emails, lock screens, and other elements looks better. As with font styles, choosing different font colors can be a good way to avoid text on your phone looking monotonous. How to Change Font Color in Pages App You can change the text color of a document on your iPhone, or you can do it by opening the Pages app on iOS. Within Pages, click the document you want to open. If the document is open in screen view, click the Edit button in the upper right corner. The selected document will now enter editing mode. To change the font color of text in this document, click the desired text to highlight it. Highlight

With the development of artificial intelligence technology, Natural Language Processing (NLP) has become a very important technology. NLP can help us better understand and analyze human language to achieve some automated tasks, such as intelligent customer service, sentiment analysis, machine translation, etc. In this article, we will cover the basics and tools for natural language processing using PHP. What is natural language processing? Natural language processing is a method that uses artificial intelligence technology to process
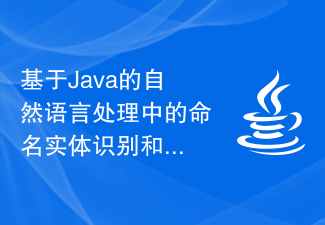
With the advent of the Internet era, a large amount of text information has flooded into our field of vision, followed by people's growing needs for information processing and analysis. At the same time, the Internet era has also brought about the rapid development of natural language processing technology, allowing people to better obtain valuable information from texts. Among them, named entity recognition and relationship extraction technology are one of the important research directions in the field of natural language processing applications. 1. Named entity recognition technology Named entities refer to people, places, organizations, time, currency, encyclopedia knowledge, measurement terms, and professions.
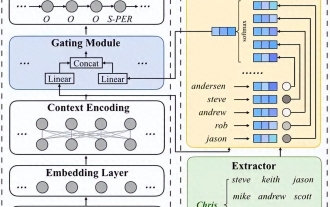
Natural Language Processing (NLP) is an important and exciting technology in the field of artificial intelligence. Its goal is to enable computers to understand, parse and generate human language. The development of NLP has made tremendous progress, enabling computers to better interact with humans and achieve a wider range of applications. This article will explore the concepts, technologies, applications and future prospects of natural language processing. The concept of natural language processing. Natural language processing is a discipline that studies how to enable computers to understand and process human language. The complexity and ambiguity of human language make computers face huge challenges in understanding and processing. The goal of NLP is to develop algorithms and models that enable computers to extract information from text
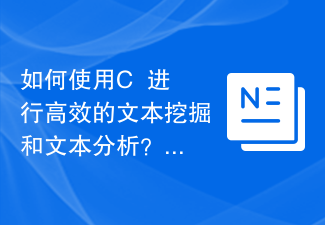
How to use C++ for efficient text mining and text analysis? Overview: Text mining and text analysis are important tasks in the field of modern data analysis and machine learning. In this article, we will introduce how to use C++ language for efficient text mining and text analysis. We will focus on techniques in text preprocessing, feature extraction, and text classification, accompanied by code examples. Text preprocessing: Before text mining and text analysis, the original text usually needs to be preprocessed. Preprocessing includes removing punctuation, stop words and special
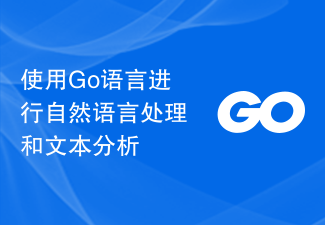
Natural Language Processing (NLP) is an interdisciplinary field involving computer science, artificial intelligence, linguistics and other disciplines. Its purpose is to aid the computer's ability to understand, interpret and generate natural language. Text analysis (TextAnalysis) is one of the important directions of NLP. Its main purpose is to extract meaningful information from large amounts of text data to support application scenarios such as business decision-making, linguistic research, and public opinion analysis. Go language in
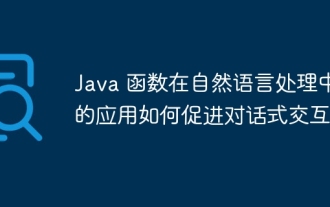
Java functions are widely used in NLP to create custom solutions that enhance the experience of conversational interactions. These functions can be used for text preprocessing, sentiment analysis, intent recognition, and entity extraction. For example, by using Java functions for sentiment analysis, applications can understand the user's tone and respond appropriately, enhancing the conversational experience.
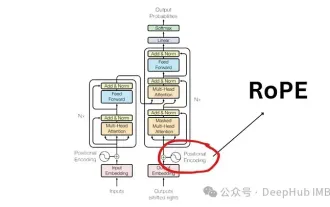
Since the "AttentionIsAllYouNeed" paper published in 2017, the Transformer architecture has been a cornerstone of the natural language processing (NLP) field. Its design has remained largely unchanged for years, with 2022 marking a major development in the field with the introduction of Rotary Position Encoding (RoPE). Rotated position embedding is the state-of-the-art NLP position embedding technique. Most popular large-scale language models (such as Llama, Llama2, PaLM and CodeGen) already use it. In this article, we’ll take a deep dive into what rotational position encodings are and how they neatly combine the benefits of absolute and relative position embeddings. The need for positional encoding in order to understand Ro
