How to defend against security attacks on PHP framework?
Jun 03, 2024 pm 06:15 PMCommon PHP framework security attacks include XSS, SQL injection, CSRF, file upload vulnerabilities, and RCE. Defense measures include: validating input; preparing SQL queries; preventing CSRF attacks; restricting file uploads; and patching framework vulnerabilities.
How to defend against security attacks on PHP frameworks
PHP frameworks are widely used to build dynamic websites, but they are also susceptible to various Security attacks. To protect your applications, it's critical to understand these attacks and take appropriate precautions.
Common PHP framework security attacks
- Cross-site scripting (XSS): The attacker injects malicious scripts that are executed in the user's browser.
- SQL Injection: An attacker manipulates a database by entering malicious queries, thereby gaining unauthorized access.
- Cross-site request forgery (CSRF): An attacker tricks a user into sending malicious requests to an application without their knowledge.
- File Upload Vulnerability: An attacker uploads a malicious file, such as a web shell containing a backdoor.
- Remote Code Execution (RCE): An attacker can execute arbitrary code by exploiting a vulnerability in a framework.
Defenses
1. Use validated input
Input validation eliminates the ability of an attacker to enter potentially dangerous characters or codes.
<?php // 使用 PHP 内置函数过滤用户输入 $sanitized_input = filter_input(INPUT_POST, 'input_field', FILTER_SANITIZE_STRING); ?>
2. Prepare SQL queries
Using prepared statements prevents SQL injection attacks because it automatically escapes user input.
<?php // 准备并执行带有占位符的 SQL 查询 $stmt = $conn->prepare("SELECT * FROM users WHERE username = ?"); $stmt->bind_param("s", $username); $stmt->execute(); ?>
3. Prevent CSRF attacks
Using anti-CSRF tokens prevents CSRF attacks because it requires each request to be authenticated within the application.
<?php // 在表单中添加一个隐藏的反 CSRF 令牌 echo '<input type="hidden" name="csrf_token" value="' . $csrf_token . '">'; // 在服务器端验证反 CSRF 令牌 if (!isset($_POST['csrf_token']) || $_POST['csrf_token'] != $csrf_token) { die("Invalid CSRF token"); } ?>
4. Limit file uploads
Limiting file upload sizes, types, and extensions can help prevent file upload vulnerabilities.
<?php // 定义允许的文件类型 $allowed_extensions = ['jpg', 'png', 'pdf']; // 检查文件大小和扩展名 if ($_FILES['file']['size'] > 1000000 || !in_array(pathinfo($_FILES['file']['name'], PATHINFO_EXTENSION), $allowed_extensions)) { die("Invalid file"); } ?>
5. Patch framework vulnerabilities
Framework vendors regularly release patches to fix vulnerabilities. It is crucial to keep your framework version up to date.
composer update
Practical Example
You can prevent cross-site scripting (XSS) attacks by applying precautions (such as filtering input, preparing statements) in the following code snippet:
<?php // 过滤用户输入 $comment = filter_input(INPUT_POST, 'comment', FILTER_SANITIZE_STRING); // 使用准备好的语句插入评论 $stmt = $conn->prepare("INSERT INTO comments (comment) VALUES (?)"); $stmt->bind_param("s", $comment); $stmt->execute(); ?>
Following these steps will help protect your PHP framework application from security attacks.
The above is the detailed content of How to defend against security attacks on PHP framework?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
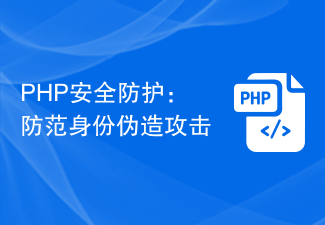
PHP security protection: Prevent identity forgery attacks
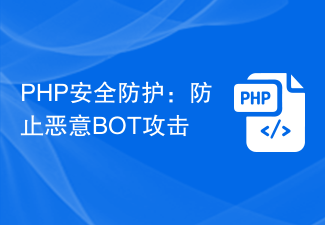
PHP security protection: Prevent malicious BOT attacks
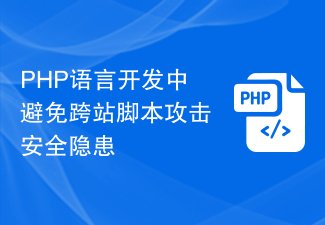
Avoid security risks of cross-site scripting attacks in PHP language development
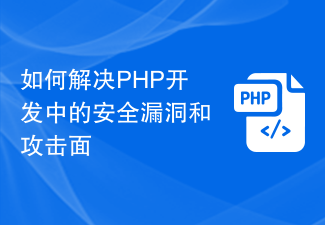
How to address security vulnerabilities and attack surfaces in PHP development
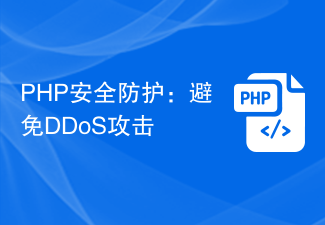
PHP security protection: avoid DDoS attacks
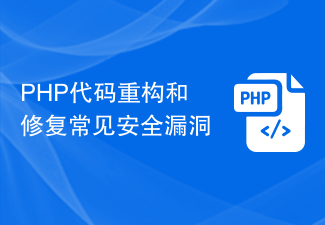
PHP code refactoring and fixing common security vulnerabilities
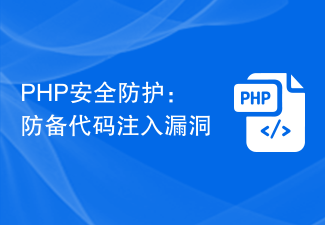
PHP security protection: Prevent code injection vulnerabilities
