How to perform memory optimization when using C++ STL?
Use the following optimization strategies to optimize memory usage in C++ STL: 1. Use a custom allocator to control the memory allocation method; 2. Use reserve() to pre-allocate space to avoid dynamic memory allocation overhead; 3. Use move semantics or Reference semantics to avoid unnecessary memory copies.
Memory Optimization in C++ STL
STL (Standard Template Library) is a widely used library in C++ that provides A set of efficient and well-tested data structures and algorithms. However, when using STL, improper memory management can cause performance issues. Here are some tips for optimizing memory usage:
Using custom allocators
You can control how an STL container allocates memory by providing a custom allocator. Custom allocators can implement various optimization strategies, such as:
// 自定义分配器用于使用内存池分配内存 class MyAllocator { std::vector<int> memory_pool; public: void* allocate(std::size_t size) { if (memory_pool.size() >= size) { void* ptr = &memory_pool[0]; memory_pool.erase(memory_pool.begin()); return ptr; } return std::malloc(size); } void deallocate(void* ptr, std::size_t size) { // 将内存返回到池中 memory_pool.push_back(*static_cast<int*>(ptr)); } };
By passing MyAllocator
to the container constructor, we can use custom allocation strategies:
std::vector<int, MyAllocator> my_vector;
Using container size optimization
STL containers often use dynamic memory allocation, so it is critical to pre-allocate enough space. A given number of elements can be preallocated using the reserve()
method:
std::vector<int> my_vector; my_vector.reserve(100);
Avoid unnecessary copying
STL algorithms and container operations can Creates new objects, causing unnecessary memory copying. To avoid this situation, you can use move semantics or reference semantics. For example, use std::move()
to move elements to a container instead of copying:
std::vector<int> my_vector; my_vector.push_back(std::move(my_value));
Practical case
The following example demonstrates Learn how to optimize memory allocation using a custom allocator:
#include#include // 自定义分配器使用内存池分配内存 class MyAllocator : public std::allocator { std::vector memory_pool; public: MyAllocator() {} MyAllocator(const MyAllocator&) = default; template MyAllocator(const MyAllocator&) {} int* allocate(std::size_t n) { if (n <= memory_pool.size()) { int* ptr = &memory_pool[0]; memory_pool.erase(memory_pool.begin()); return ptr; } return std::allocator ::allocate(n); } void deallocate(int* ptr, std::size_t) { // 将内存返回到池中 memory_pool.push_back(*ptr); std::allocator ::deallocate(ptr, 1); } }; int main() { // 使用自定义分配器创建 vector std::vector<int, MyAllocator> my_vector; // 分配 1000 个元素 my_vector.reserve(1000); // 使用自定义分配器分配的内存的效率更高 return 0; }
The above is the detailed content of How to perform memory optimization when using C++ STL?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
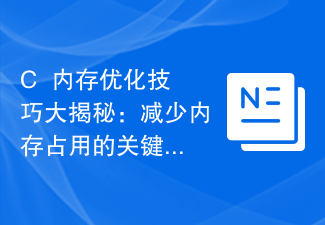
C++ is an efficient and powerful programming language, but when processing large-scale data or running complex programs, memory optimization becomes an issue that developers cannot ignore. Properly managing and reducing memory usage can improve program performance and reliability. This article will reveal some key tips for reducing memory footprint in C++ to help developers build more efficient applications. Use appropriate data types In C++ programming, choosing the appropriate data type is an important step in reducing memory usage. For example, if you only need to represent a small range of integers, you can use
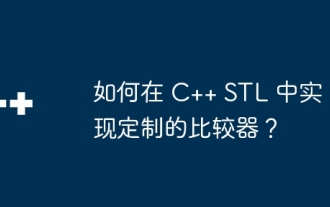
Implementing a custom comparator can be accomplished by creating a class that overloads operator(), which accepts two parameters and indicates the result of the comparison. For example, the StringLengthComparator class sorts strings by comparing their lengths: Create a class and overload operator(), returning a Boolean value indicating the comparison result. Using custom comparators for sorting in container algorithms. Custom comparators allow us to sort or compare data based on custom criteria, even if we need to use custom comparison criteria.
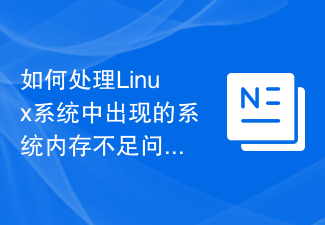
How to deal with the problem of insufficient system memory in the Linux system Summary: The Linux system is an operating system with strong stability and high security, but sometimes it encounters the problem of insufficient system memory. This article will introduce some common processing methods to help users solve this problem. Keywords: Linux system, system memory, shortage, processing method Text: Introduction Linux system, as an open source operating system, is widely used in various servers and embedded devices. However, sometimes we will find that during operation, the system
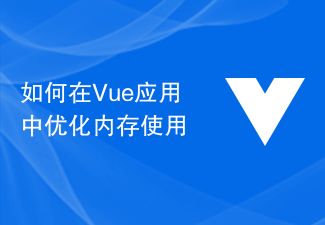
How to optimize memory usage in Vue applications With the popularity of Vue, more and more developers are beginning to use Vue to build applications. However, in larger Vue applications, memory usage can become an issue due to DOM manipulation and Vue's reactive system. This article will introduce some tips and suggestions on how to optimize memory usage in Vue applications. Reasonable use of v-if and v-for It is very common to use v-if and v-for directives in Vue applications. However, excessive use of these two instructions may cause memory
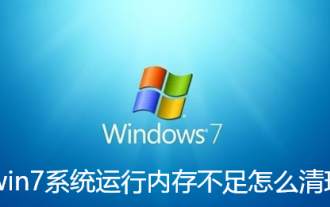
How to clean up insufficient memory in win7 system? When the computer was running, some software was opened. Soon after, the computer manager displayed a memory prompt, indicating that our computer had insufficient memory space. In this situation, if we don’t open many software ourselves, it may be caused by the self-starting of the program the day after tomorrow. Many friends don’t know how to operate in detail. The editor has compiled a tutorial on how to solve the problem of insufficient memory when running Windows 7 system. If you are interested, Follow the editor and take a look below! Tutorial on solving insufficient memory when running Windows 7 system Method 1. Disable automatic updates 1. Click Start to open the Control Panel. 2. Click Windowsupdate. 3. Click on the left to change settings. 4. Choose never to check
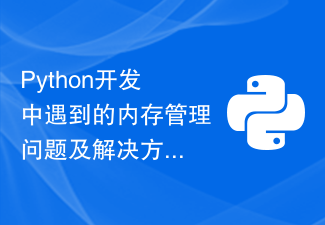
Summary of memory management problems and solutions encountered in Python development: In the Python development process, memory management is an important issue. This article will discuss some common memory management problems and introduce corresponding solutions, including reference counting, garbage collection mechanism, memory allocation, memory leaks, etc. Specific code examples are provided to help readers better understand and deal with these issues. Reference Counting Python uses reference counting to manage memory. Reference counting is a simple and efficient memory management method that records every

Using STL function objects can improve reusability and includes the following steps: Define the function object interface (create a class and inherit from std::unary_function or std::binary_function) Overload operator() to define the function behavior in the overloaded operator() Implement the required functionality using function objects via STL algorithms (such as std::transform)
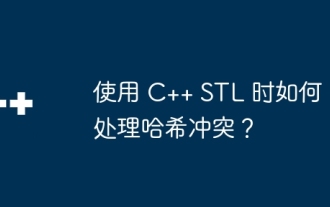
The methods for handling C++STL hash conflicts are: chain address method: using linked lists to store conflicting elements, which has good applicability. Open addressing method: Find available locations in the bucket to store elements. The sub-methods are: Linear detection: Find the next available location in sequence. Quadratic Detection: Search by skipping positions in quadratic form.
