How to improve iteration efficiency in C++ STL?
Methods to improve the efficiency of C++ STL iteration include: choosing appropriate containers, such as using vector for fast random access and unordered_map/set for efficient search. Leverage range loops to simplify iteration syntax, and consider using const or reverse iterators to optimize performance. Parallelize iterations in C++17 and higher to take advantage of multi-core processors for greater efficiency.
How to improve iteration efficiency in C++ STL?
STL (Standard Template Library) is a powerful toolset in the C++ standard library that provides various containers and algorithms. However, when it comes to iterating on large data sets, efficiency is crucial. Here are some strategies to improve iteration efficiency in C++ STL:
1. Choose the right container
- Use vector instead of list:If you need it frequently For random access, use vector because it provides fast and efficient random access capabilities.
- Consider unordered_map or unordered_set: For lookup operations, unordered_map and unordered_set tend to be more efficient than map and set because they use hash tables to quickly find elements.
2. Using range loops
-
C++11 introduces range loops: It allows you to use range loops more concisely and more efficiently syntax to iterate over a container. For example:
for (auto& element : container) { // 使用 element }
Copy after login
3. Optimize iterator type
- Use const iterator: Use const when the container does not need to be modified. Iterators can improve performance because the compiler can optimize the code more aggressively.
- Use reverse_iterator: If you need to iterate from the end of the container to the beginning, please use reverse_iterator, which avoids the performance overhead of reverse iteration.
4. Parallelized iteration
For C++17 and higher:You can use the parallel algorithm to parallelize iteration, Thus taking advantage of multi-core processors. For example:
std::for_each(std::execution::par, container.begin(), container.end(), [](auto& element) { // 使用 element });
Copy after login
Practical case
Consider the following example, which uses list and vector to store a list of integers:
#include <iostream> #include <list> #include <vector> int main() { // 使用 list 进行迭代 std::list<int> list = {1, 2, 3, 4, 5}; for (auto& element : list) { std::cout << element << " "; } std::cout << std::endl; // 使用 vector 进行迭代 std::vector<int> vector = {1, 2, 3, 4, 5}; for (auto& element : vector) { std::cout << element << " "; } std::cout << std::endl; return 0; }
Using vector for iteration is better than using list is faster because vector has more efficient random access capabilities.
The above is the detailed content of How to improve iteration efficiency in C++ STL?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


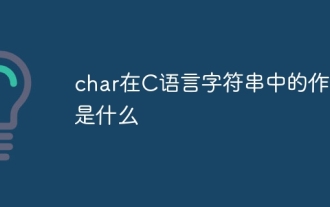
In C, the char type is used in strings: 1. Store a single character; 2. Use an array to represent a string and end with a null terminator; 3. Operate through a string operation function; 4. Read or output a string from the keyboard.
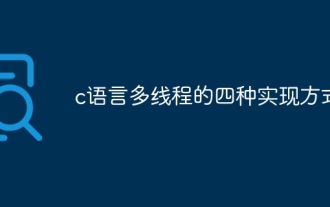
Multithreading in the language can greatly improve program efficiency. There are four main ways to implement multithreading in C language: Create independent processes: Create multiple independently running processes, each process has its own memory space. Pseudo-multithreading: Create multiple execution streams in a process that share the same memory space and execute alternately. Multi-threaded library: Use multi-threaded libraries such as pthreads to create and manage threads, providing rich thread operation functions. Coroutine: A lightweight multi-threaded implementation that divides tasks into small subtasks and executes them in turn.
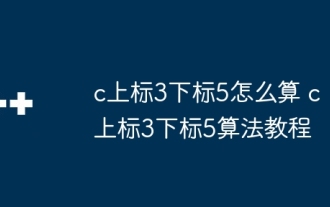
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
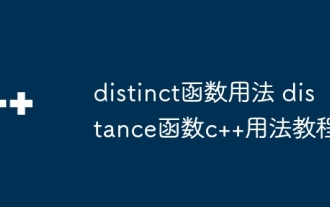
std::unique removes adjacent duplicate elements in the container and moves them to the end, returning an iterator pointing to the first duplicate element. std::distance calculates the distance between two iterators, that is, the number of elements they point to. These two functions are useful for optimizing code and improving efficiency, but there are also some pitfalls to be paid attention to, such as: std::unique only deals with adjacent duplicate elements. std::distance is less efficient when dealing with non-random access iterators. By mastering these features and best practices, you can fully utilize the power of these two functions.
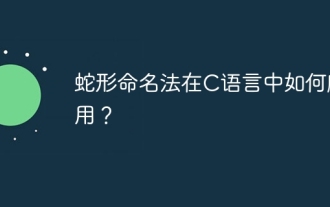
In C language, snake nomenclature is a coding style convention, which uses underscores to connect multiple words to form variable names or function names to enhance readability. Although it won't affect compilation and operation, lengthy naming, IDE support issues, and historical baggage need to be considered.
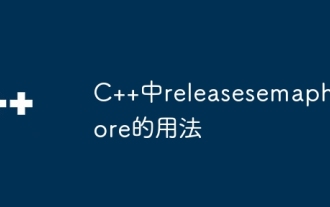
The release_semaphore function in C is used to release the obtained semaphore so that other threads or processes can access shared resources. It increases the semaphore count by 1, allowing the blocking thread to continue execution.
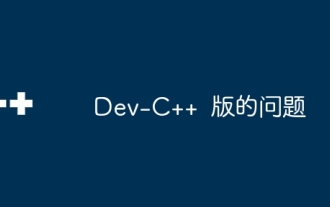
Dev-C 4.9.9.2 Compilation Errors and Solutions When compiling programs in Windows 11 system using Dev-C 4.9.9.2, the compiler record pane may display the following error message: gcc.exe:internalerror:aborted(programcollect2)pleasesubmitafullbugreport.seeforinstructions. Although the final "compilation is successful", the actual program cannot run and an error message "original code archive cannot be compiled" pops up. This is usually because the linker collects
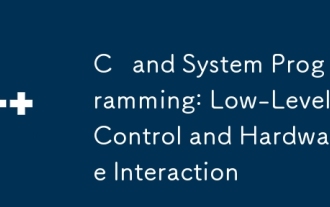
C is suitable for system programming and hardware interaction because it provides control capabilities close to hardware and powerful features of object-oriented programming. 1)C Through low-level features such as pointer, memory management and bit operation, efficient system-level operation can be achieved. 2) Hardware interaction is implemented through device drivers, and C can write these drivers to handle communication with hardware devices.
