Common problems and solutions to PHP database connections
Common problems and solutions for PHP database connections are: connection failure: check connection information and MySQL service status; query failure: check query syntax, tables and fields, and connection validity; insertion, update, and delete failures: check SQL Statements, target tables and fields, and connection validity; database connection leaks: explicitly close the connection or use a try...catch...finally block.
Common problems and solutions to PHP database connections
1. Connection failure
Problem 1: mysqli_connect()
Returns false
$conn = mysqli_connect("localhost", "username", "password", "database"); if (!$conn) { echo "连接失败:" . mysqli_connect_error(); }
Solution:
- Check the host name , user name, password and database name are correct.
- Make sure the MySQL server is running.
- Check that PHP has MySQL extension enabled.
Problem 2: PDO::__construct()
throws an exception
$dsn = "mysql:host=localhost;dbname=database"; $conn = new PDO($dsn, "username", "password");
Solution:
- Check whether the connection string is correct.
- Make sure the PDO MySQL driver is enabled.
- Check that PHP has MySQL extension enabled.
2. Query failed
Question 1: mysqli_query()
Returns false
$query = "SELECT * FROM users"; $result = mysqli_query($conn, $query); if (!$result) { echo "查询失败:" . mysqli_error($conn); }
Solution:
- Check whether the query syntax is correct.
- Make sure the table and fields exist.
- Check whether the connection is valid.
Problem 2: PDOStatement::execute() throws an exception
$stmt = $conn->prepare($query); $stmt->execute();
Solution:
- Check whether the query parameters are correct.
- Check whether the connection is valid.
- Make sure there are no errors in the query results.
3. Insertion, update, and deletion failed
Problem: mysqli_affected_rows()
or PDOStatement:: rowCount()
returns 0
Solution:
- Check whether the SQL statement is correct.
- Make sure the target table and fields exist.
- Check whether the connection is valid.
4. Other problems
Problem: Database connection leakage
Solution:
- Use
mysqli_close()
orPDO::close()
to explicitly close the connection. - Use PHP's
try...catch...finally
block to ensure that the connection is closed in any case.
Practical case
Connect to MySQL database
// PHP >= 8.0,推荐使用 PDO $dsn = "mysql:host=localhost;dbname=database"; $conn = new PDO($dsn, "username", "password"); // PHP < 8.0,使用 mysqli_connect() $conn = mysqli_connect("localhost", "username", "password", "database");
Query database
// PDO $stmt = $conn->prepare("SELECT * FROM users WHERE id = ?"); $stmt->execute([$id]); $result = $stmt->fetchAll(); // mysqli $query = "SELECT * FROM users WHERE id = " . $id; $result = mysqli_query($conn, $query); $result = mysqli_fetch_all($result);
The above is the detailed content of Common problems and solutions to PHP database connections. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


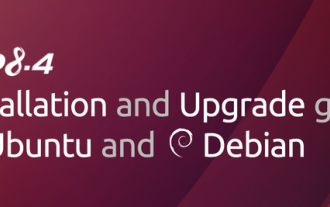
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
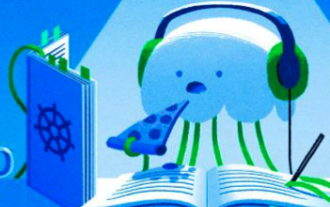
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
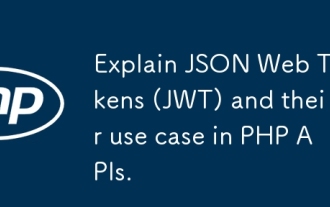
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
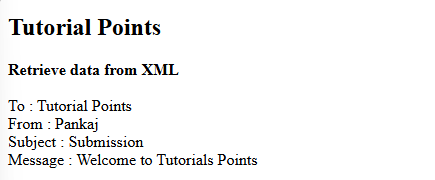
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
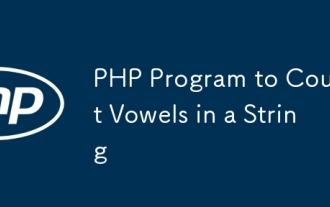
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
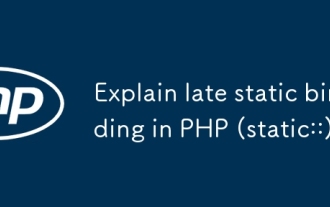
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
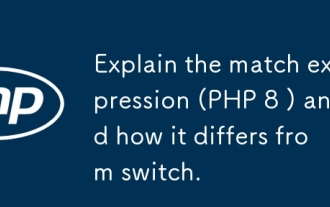
In PHP8, match expressions are a new control structure that returns different results based on the value of the expression. 1) It is similar to a switch statement, but returns a value instead of an execution statement block. 2) The match expression is strictly compared (===), which improves security. 3) It avoids possible break omissions in switch statements and enhances the simplicity and readability of the code.
