Golang framework source code unit testing practice
Best practices for unit testing of Go framework source code: Use BDD style to write test cases to enhance readability. Use mock objects to simulate external dependencies and focus on the code under test. Cover all code branches to ensure the code runs correctly under all circumstances. Use coverage tools to monitor the scope of test cases and improve test reliability. Ensure the independence of test cases and facilitate problem isolation and debugging.
Go framework source code unit testing practice
Unit testing is a crucial link in software development and can help verify the code Correctness and robustness. For the Go framework source code, unit testing is particularly important because it can ensure the correct operation of the framework. This article will introduce the best practices for source code unit testing of the Go framework and demonstrate it through practical cases.
Best Practices
- Follow the BDD style: Write test cases using the BDD (Behavior-Driven Development) style, that is, Given-When -Then format. This helps improve the readability and maintainability of test cases.
- Use mock objects: Mock objects can simulate external dependencies, allowing you to focus on testing the correctness of the code under test without being affected by external factors.
- Cover all branches: Unit tests should cover all branches in the code, including true and false branches. This helps ensure that the code runs correctly under all circumstances.
- Use code coverage tools: Code coverage tools can help you monitor the coverage of test cases. Ensure a high level of coverage to improve test reliability.
- Keep test cases independent: Unit test cases should be independent of each other to avoid relying on other test cases. This helps isolate the problem and simplifies the debugging process.
Practical case
Let’s take the following simple Go function as an example:
func Sum(a, b int) int { return a + b }
Unit test
import ( "testing" ) func TestSum(t *testing.T) { tests := []struct { a, b, exp int }{ {1, 2, 3}, {-1, 0, -1}, {0, 5, 5}, } for _, test := range tests { t.Run("input: "+fmt.Sprintf("%d, %d", test.a, test.b), func(t *testing.T) { got := Sum(test.a, test.b) if got != test.exp { t.Errorf("expected: %d, got: %d", test.exp, got) } }) } }
In this test case, we used some best practices:
- Write test cases using BDD style.
- Use data-driven testing methods to manage different inputs and expected outputs.
- Create an independent subtest for each set of inputs.
Run the test
Run the unit test using the go test
command:
$ go test
The output should look similar to:
PASS ok command-line-arguments 0.535s
Conclusion
This article introduces the best practices for unit testing of Go framework source code and demonstrates it through practical cases. Following these best practices allows you to write robust and reliable unit tests, ensuring the correctness and reliability of your framework's code.
The above is the detailed content of Golang framework source code unit testing practice. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
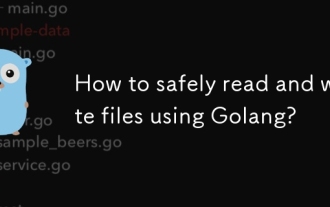
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
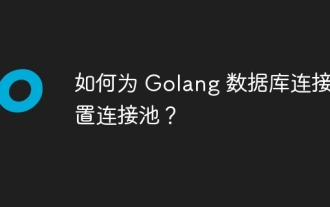
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
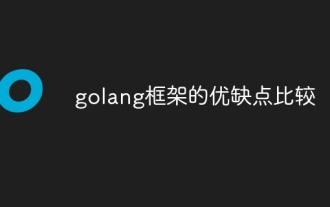
The Go framework stands out due to its high performance and concurrency advantages, but it also has some disadvantages, such as being relatively new, having a small developer ecosystem, and lacking some features. Additionally, rapid changes and learning curves can vary from framework to framework. The Gin framework is a popular choice for building RESTful APIs due to its efficient routing, built-in JSON support, and powerful error handling.
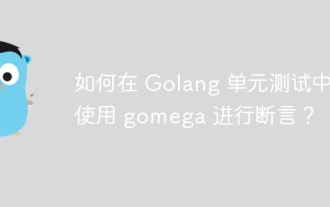
How to use Gomega for assertions in Golang unit testing In Golang unit testing, Gomega is a popular and powerful assertion library that provides rich assertion methods so that developers can easily verify test results. Install Gomegagoget-ugithub.com/onsi/gomega Using Gomega for assertions Here are some common examples of using Gomega for assertions: 1. Equality assertion import "github.com/onsi/gomega" funcTest_MyFunction(t*testing.T){

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
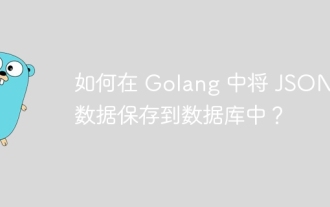
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
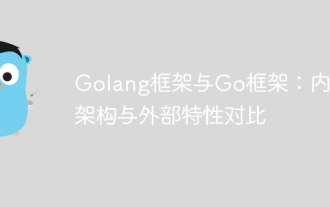
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
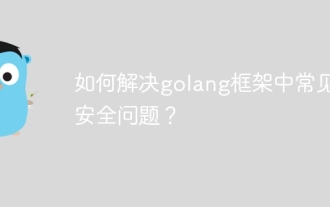
How to address common security issues in the Go framework With the widespread adoption of the Go framework in web development, ensuring its security is crucial. The following is a practical guide to solving common security problems, with sample code: 1. SQL Injection Use prepared statements or parameterized queries to prevent SQL injection attacks. For example: constquery="SELECT*FROMusersWHEREusername=?"stmt,err:=db.Prepare(query)iferr!=nil{//Handleerror}err=stmt.QueryR
