How to manage Goroutine lifecycle in Go?
The core methods of managing the Goroutine life cycle in Go are as follows: Use context.Context: Control the Goroutine life cycle through cancellation signals and deadlines. Use sync.WaitGroup: Wait for the Goroutine to complete its task so that the main Goroutine can continue execution. Use channels: coordinate multiple Goroutines through signal communication, and wait for subsequent processing after all Goroutines are completed.
Manage the life cycle of Goroutine in Go
Goroutine is a lightweight thread in Go that supports concurrency. Their management is crucial to prevent resource leaks and program crashes. This article will explore various methods of managing the Goroutine life cycle, including:
1. Using context.Context
context.Context
Provides a mechanism to propagate cancellation and deadline signals within Goroutine. To use it to manage the Goroutine life cycle, you can use the following steps:
package main import ( "context" "fmt" "time" ) func main() { ctx, cancel := context.WithTimeout(context.Background(), 10*time.Second) defer cancel() go func() { // Goroutine 的代码 }() // 等待 Goroutine 完成,或超时。 select { case <-ctx.Done(): fmt.Println("Goroutine 已取消或超时。") } }
2. Use sync.WaitGroup
sync .WaitGroup
Allows Goroutines to wait for each other until they complete their respective tasks. Use it to manage the life cycle of Goroutine. You can wait in the main Goroutine for all child Goroutines to complete.
package main import ( "fmt" "runtime" "sync" ) func main() { wg := sync.WaitGroup{} // 创建 5 个子 Goroutine for i := 0; i < 5; i++ { wg.Add(1) // 递增WaitGroup计数,表明正在等待另一个Goroutine完成 go func(i int) { fmt.Printf("Goroutine %d 结束。\n", i) wg.Done() // 递减WaitGroup计数,表明Goroutine已完成 }(i) } // 等待所有子 Goroutine 完成 wg.Wait() fmt.Println("所有子 Goroutine 都已完成。") }
3. Using channels
Channels can communicate between Goroutines and can also be used to manage their life cycles. A master goroutine can wait for all child goroutines to complete by sending a signal to a channel that marks the goroutine as completed.
package main import ( "fmt" "sync" ) func main() { var wg sync.WaitGroup signals := make(chan struct{}) // 创建 5 个子 Goroutine for i := 0; i < 5; i++ { wg.Add(1) go func(i int) { defer wg.Done() // Goroutine退出时递减WaitGroup计数 // 等待其他Goroutine完成 <-signals fmt.Printf("Goroutine %d 已完成。\n", i) }(i) } // 等待所有子 Goroutine完成 wg.Wait() // 发送信号标记所有子Goroutine都已完成 close(signals) fmt.Println("所有子 Goroutine 都已完成。") }
The above is the detailed content of How to manage Goroutine lifecycle in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
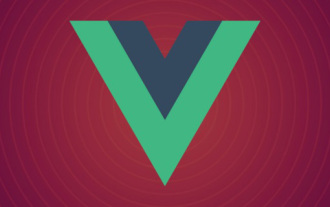
vue3 changed 4 life cycle functions. The Vue3 combined API cancels the beforeCreated and created hook functions and uses the step hook instead, and this cannot be used in it. The hook functions for component destruction in Vue3 have been changed from destroyed and beforeDestroy to beforeUnmount and unmounted.
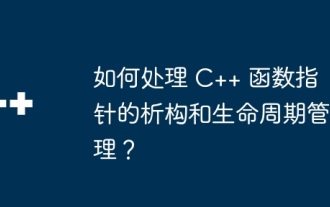
In C++, function pointers require proper destruction and life cycle management. This can be achieved by manually destructing the function pointer and releasing the memory. Use smart pointers, such as std::unique_ptr or std::shared_ptr, to automatically manage the life cycle of function pointers. Bind the function pointer to the object, and the object life cycle manages the destruction of the function pointer. In GUI programming, using smart pointers or binding to objects ensures that callback functions are destructed at the appropriate time, avoiding memory leaks and inconsistencies.
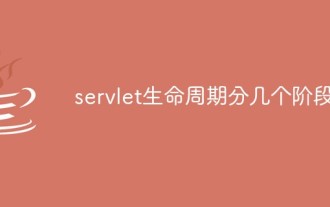
The Servlet life cycle refers to the entire process from creation to destruction of a servlet, which can be divided into three stages: 1. Initialization stage, calling the init() method to initialize the Servlet; 2. Running stage (processing requests), the container will Request to create a ServletRequest object representing an HTTP request and a ServletResponse object representing an HTTP response, and then pass them as parameters to the service() method of the Servlet; 3. Destruction phase.
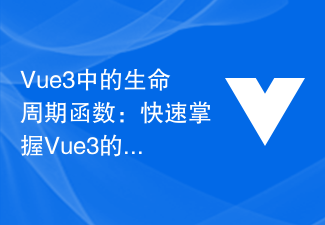
Vue3 is currently one of the most popular frameworks in the front-end world, and the life cycle function of Vue3 is a very important part of Vue3. Vue3's life cycle function allows us to trigger specific events at specific times, enhancing the high degree of controllability of components. This article will explore and explain in detail the basic concepts of Vue3's life cycle functions, the roles and usage of each life cycle function, and implementation cases, to help readers quickly master Vue3's life cycle functions. 1. Vue3’s life cycle function
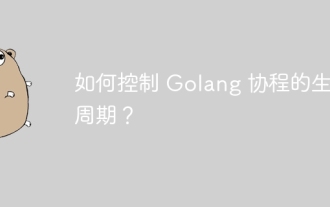
Controlling the life cycle of a Go coroutine can be done in the following ways: Create a coroutine: Use the go keyword to start a new task. Terminate coroutines: wait for all coroutines to complete, use sync.WaitGroup. Use channel closing signals. Use context context.Context.
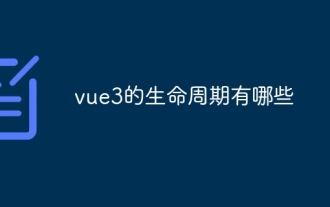
vue3的生命周期:1、beforeCreate;2、created;3、beforeMount;4、mounted;5、beforeUpdate;6、updated;7、beforeDestroy;8、destroyed;9、activated;10、deactivated;11、errorCaptured;12、getDerivedStateFromProps等等
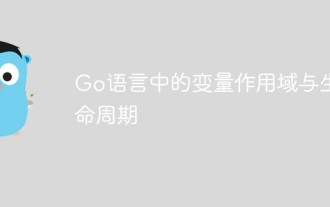
Go language is an open source statically typed language. It has the characteristics of simplicity, efficiency and reliability, and is increasingly loved by developers. In the Go language, variables are the most basic form of data storage in programs. The scope and life cycle of variables are very important to the correctness and efficiency of the program. The scope of a variable refers to the visibility and accessibility of the variable, that is, where the variable can be accessed. In the Go language, the scope of variables is divided into global variables and local variables. Global variables are variables defined outside a function and can be used anywhere in the entire program
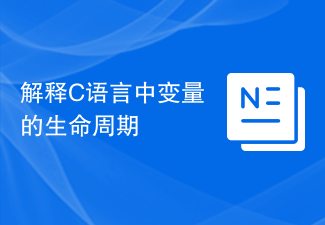
Storage classes specify the scope, lifetime, and binding of variables. To fully define a variable, it is necessary to mention not only its "type" but also its storage class. A variable name identifies a physical location in computer memory where a set of bits is allocated to store the variable's value. Storage class tells us the following factors - Where are the variables stored (in memory or CPU registers)? If not initialized, what is the initial value of the variable? What is the scope of a variable (the scope within which the variable can be accessed)? What is the life cycle of a variable? The lifetime of a lifetime variable defines the duration for which the computer allocates memory (the duration between memory allocation and deallocation). In C language, variables can have automatic, static or dynamic life cycle. Automatic - create a life cycle with automatic
