C++ Tips Guide: Unlocking the Secrets of Efficient Coding
Use the following tips to improve C++ coding efficiency: smart pointers: prevent memory leaks and double-free range-based for loops: simplify container traversal lambda expressions: for one-time code block movement semantics: efficient object ownership transfer optimized container selection: Choose the right container based on performance needs
C++ Tips Guide: Unlocking the Secret to Efficient Coding
Introduction
C++ is A high-level programming language known for excellence in performance, efficiency, and plasticity. Mastering its tips and tricks can greatly increase coding speed and enhance code quality.
1. Use smart pointers
Smart pointers (such as unique_ptr
and shared_ptr
) can help manage memory and prevent Leaks and double releases. Using them can significantly improve the stability and maintainability of your code.
class Node { public: Node* next; int data; Node(int data) : data(data), next(nullptr) {} }; int main() { std::unique_ptr<Node> ptr(new Node(10)); }
2. Apply range-based for loop
The range-based for loop provides a concise and efficient way to traverse the container. They are more readable and maintainable than traditional C-style for loops.
std::vector<int> numbers = {1, 2, 3, 4, 5}; for (int num : numbers) { std::cout << num << std::endl; }
3. Use lambda expressions
Lambda expressions are anonymous functions that allow you to write concise, one-time use blocks of code. They are particularly useful for event handling and functional programming.
std::function<int(int)> square = [](int x) { return x * x; }; std::cout << square(5) << std::endl; // 输出:25
4. Understand move semantics
Move semantics allow the transfer of ownership of an object without copying it. It improves performance and avoids unnecessary deep copies.
class Box { public: int length; std::string name; Box(int length, std::string name) : length(length), name(name) {} // 移动构造函数 Box(Box&& other) : length(std::move(other.length)), name(std::move(other.name)) { other.length = 0; other.name = ""; } };
5. Optimize Containers
Choosing the right container and applying appropriate optimizations can significantly improve the performance of your code. For example, for applications that need to find elements quickly, std::unordered_map
is a better choice.
Practical Case
Let us consider an application that needs to process large amounts of data efficiently. By applying these tips, we can drastically improve its performance:
// 使用智能指针避免内存泄漏 std::vector<std::unique_ptr<Data>> records; // 使用 range-based for 循环高效遍历容器 for (auto& record : records) { // 使用 lambda 表达式进行快速计算 double average = std::accumulate(record->values.begin(), record->values.end(), 0.0) / record->values.size(); }
Conclusion
By mastering these C++ tips, developers can unlock the powerful potential of efficient coding. Applying these tips can help improve code speed, stability, and maintainability, creating more powerful applications.
The above is the detailed content of C++ Tips Guide: Unlocking the Secrets of Efficient Coding. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


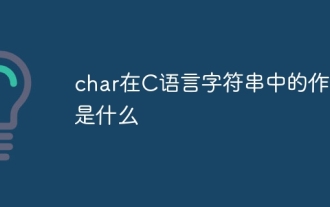
In C, the char type is used in strings: 1. Store a single character; 2. Use an array to represent a string and end with a null terminator; 3. Operate through a string operation function; 4. Read or output a string from the keyboard.
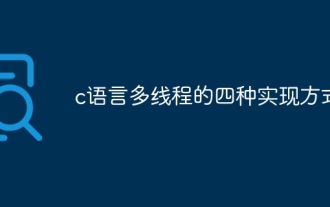
Multithreading in the language can greatly improve program efficiency. There are four main ways to implement multithreading in C language: Create independent processes: Create multiple independently running processes, each process has its own memory space. Pseudo-multithreading: Create multiple execution streams in a process that share the same memory space and execute alternately. Multi-threaded library: Use multi-threaded libraries such as pthreads to create and manage threads, providing rich thread operation functions. Coroutine: A lightweight multi-threaded implementation that divides tasks into small subtasks and executes them in turn.
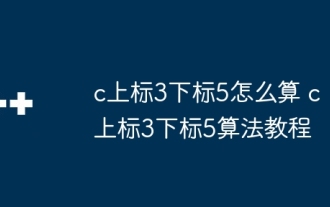
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
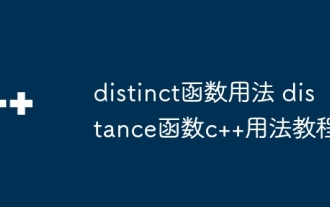
std::unique removes adjacent duplicate elements in the container and moves them to the end, returning an iterator pointing to the first duplicate element. std::distance calculates the distance between two iterators, that is, the number of elements they point to. These two functions are useful for optimizing code and improving efficiency, but there are also some pitfalls to be paid attention to, such as: std::unique only deals with adjacent duplicate elements. std::distance is less efficient when dealing with non-random access iterators. By mastering these features and best practices, you can fully utilize the power of these two functions.
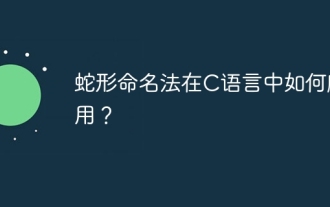
In C language, snake nomenclature is a coding style convention, which uses underscores to connect multiple words to form variable names or function names to enhance readability. Although it won't affect compilation and operation, lengthy naming, IDE support issues, and historical baggage need to be considered.
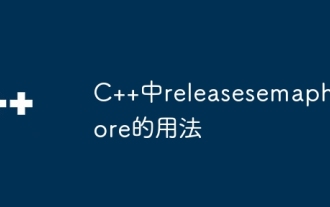
The release_semaphore function in C is used to release the obtained semaphore so that other threads or processes can access shared resources. It increases the semaphore count by 1, allowing the blocking thread to continue execution.
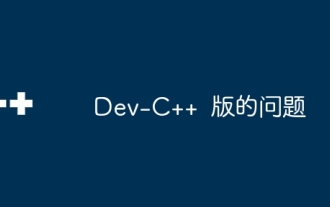
Dev-C 4.9.9.2 Compilation Errors and Solutions When compiling programs in Windows 11 system using Dev-C 4.9.9.2, the compiler record pane may display the following error message: gcc.exe:internalerror:aborted(programcollect2)pleasesubmitafullbugreport.seeforinstructions. Although the final "compilation is successful", the actual program cannot run and an error message "original code archive cannot be compiled" pops up. This is usually because the linker collects
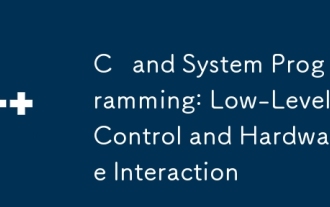
C is suitable for system programming and hardware interaction because it provides control capabilities close to hardware and powerful features of object-oriented programming. 1)C Through low-level features such as pointer, memory management and bit operation, efficient system-level operation can be achieved. 2) Hardware interaction is implemented through device drivers, and C can write these drivers to handle communication with hardware devices.
