Things to note when Golang functions receive map parameters
When passing a map to a function in Go, a copy will be created by default, and modifications to the copy will not affect the original map. If you need to modify the original map, you can pass it through a pointer. Empty maps need to be handled with care, because they are technically nil pointers, and passing an empty map to a function that expects a non-empty map will cause an error.
Things to note when Go functions receive map parameters
In Go, when passing map to a function as a parameter, there are Some precautions, if not paid attention to, may lead to unexpected results.
Copy Passing
When passing a map to a function, a copy is created by default. In other words, the map within the function is a copy of the parameter map, and any modifications to the copy will not affect the original map.
Practical case 1:
func modifyMap(m map[string]int) { m["key"] = 100 } func main() { m := make(map[string]int) modifyMap(m) fmt.Println(m) // 输出:{} }
As shown in the example, the modifications made to the map by the modifyMap
function will not affect the original map.
Avoid copy passing
If you need to modify the original map, you can use a pointer to pass it. When passed a pointer, the map within the function points to the memory address of the original map.
Practical case 2:
func modifyMap(m *map[string]int) { (*m)["key"] = 100 } func main() { m := make(map[string]int) modifyMap(&m) fmt.Println(m) // 输出:{key: 100} }
As shown in the example, the modifications made by the modifyMap
function to the map will affect the original map.
Empty map
Be careful when passing an empty map to a function. An empty map is technically a nil pointer, not a valid map. Therefore, if a function expects a non-empty map, an error may occur when passing an empty map.
Practical Case 3:
func printMap(m map[string]int) { for k, v := range m { fmt.Println(k, v) } } func main() { var m map[string]int // 空 map printMap(m) // 运行时错误:panic: runtime error: invalid memory address or nil pointer dereference }
As shown in the example, passing an empty map to the function will cause a runtime error. To solve this problem, you can perform a non-null check on the map, or provide a default value.
Conclusion:
- When you pass a map to a function, a copy is created by default.
- To modify the original map, you can use a pointer to pass it.
- Be careful with empty maps.
The above is the detailed content of Things to note when Golang functions receive map parameters. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


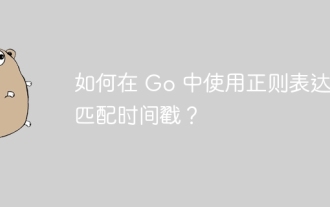
In Go, you can use regular expressions to match timestamps: compile a regular expression string, such as the one used to match ISO8601 timestamps: ^\d{4}-\d{2}-\d{2}T \d{2}:\d{2}:\d{2}(\.\d+)?(Z|[+-][0-9]{2}:[0-9]{2})$ . Use the regexp.MatchString function to check if a string matches a regular expression.
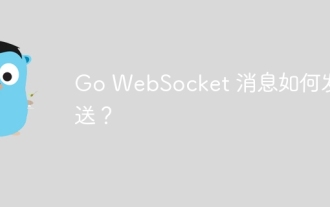
In Go, WebSocket messages can be sent using the gorilla/websocket package. Specific steps: Establish a WebSocket connection. Send a text message: Call WriteMessage(websocket.TextMessage,[]byte("Message")). Send a binary message: call WriteMessage(websocket.BinaryMessage,[]byte{1,2,3}).
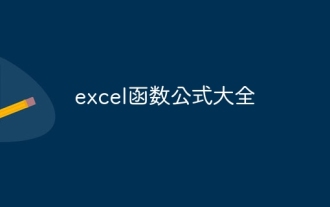
1. The SUM function is used to sum the numbers in a column or a group of cells, for example: =SUM(A1:J10). 2. The AVERAGE function is used to calculate the average of the numbers in a column or a group of cells, for example: =AVERAGE(A1:A10). 3. COUNT function, used to count the number of numbers or text in a column or a group of cells, for example: =COUNT(A1:A10) 4. IF function, used to make logical judgments based on specified conditions and return the corresponding result.
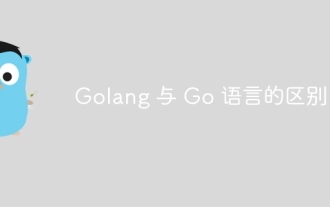
Go and the Go language are different entities with different characteristics. Go (also known as Golang) is known for its concurrency, fast compilation speed, memory management, and cross-platform advantages. Disadvantages of the Go language include a less rich ecosystem than other languages, a stricter syntax, and a lack of dynamic typing.
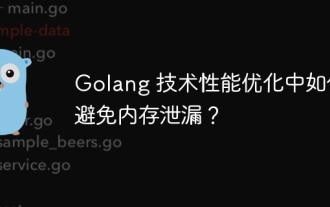
Memory leaks can cause Go program memory to continuously increase by: closing resources that are no longer in use, such as files, network connections, and database connections. Use weak references to prevent memory leaks and target objects for garbage collection when they are no longer strongly referenced. Using go coroutine, the coroutine stack memory will be automatically released when exiting to avoid memory leaks.
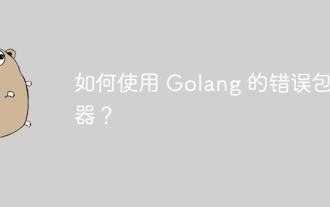
In Golang, error wrappers allow you to create new errors by appending contextual information to the original error. This can be used to unify the types of errors thrown by different libraries or components, simplifying debugging and error handling. The steps are as follows: Use the errors.Wrap function to wrap the original errors into new errors. The new error contains contextual information from the original error. Use fmt.Printf to output wrapped errors, providing more context and actionability. When handling different types of errors, use the errors.Wrap function to unify the error types.
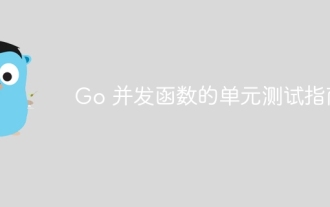
Unit testing concurrent functions is critical as this helps ensure their correct behavior in a concurrent environment. Fundamental principles such as mutual exclusion, synchronization, and isolation must be considered when testing concurrent functions. Concurrent functions can be unit tested by simulating, testing race conditions, and verifying results.
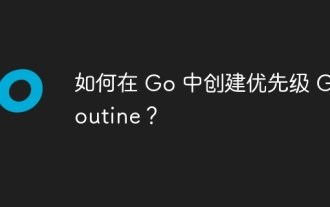
There are two steps to creating a priority Goroutine in the Go language: registering a custom Goroutine creation function (step 1) and specifying a priority value (step 2). In this way, you can create Goroutines with different priorities, optimize resource allocation and improve execution efficiency.
