How to handle empty file names in Golang file upload?
Jun 04, 2024 am 10:43 AMFor empty file names in Go file uploads, the following steps need to be taken: Check whether the FormFile object exists, and return an error if it is empty. Create a custom parser to check whether the uploaded file name is empty, and return an error if it is empty.
Guidelines for handling empty file names in Golang file uploads
When processing file uploads in the Go language, you may encounter Scenario where user submits an empty file name. This can lead to various errors that affect the stability of the application and the user experience. To solve this problem, here is a guide on how to safely and efficiently handle empty filenames in Golang file uploads.
Checking the FormFile
object
Always check whether the FormFile
object exists before processing an uploaded file. You can use the IsZero
method to check if the object is empty:
file, header, err := request.FormFile("file") if file.IsZero() { return errors.New("Empty file received") }
If the FormFile
object is empty, an error message is returned, such as "Empty file received".
Custom parsers
In some cases you may not be able to use the FormFile
object. In this case you can create a custom parser. The parser checks the uploaded file and returns a multipart.FileHeader
object or error:
func parseFile(r *http.Request, key string) (*multipart.FileHeader, error) { if r.MultipartForm == nil { return nil, errors.New("No multipart form found") } file, header, err := r.MultipartForm.File[key] if err != nil { return nil, err } if header.Filename == "" { return nil, errors.New("Empty file received") } return file, nil }
The custom parser also checks if the name of the uploaded file is empty and returns an error message , such as "Empty file received".
Practical case
The following code example shows how to apply the above technology to a real scenario:
func handleFileUpload(w http.ResponseWriter, r *http.Request) { file, header, err := r.FormFile("file") if err != nil { http.Error(w, "Error getting file: "+err.Error(), http.StatusInternalServerError) return } if file.IsZero() { http.Error(w, "Empty file received", http.StatusBadRequest) return } // 处理上传文件 // ... }
Conclusion
By following these guidelines, you can effectively handle empty file names in Golang file uploads, ensuring that your applications function properly in a variety of situations.
The above is the detailed content of How to handle empty file names in Golang file upload?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
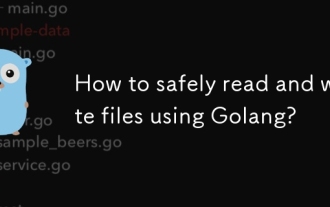
How to safely read and write files using Golang?
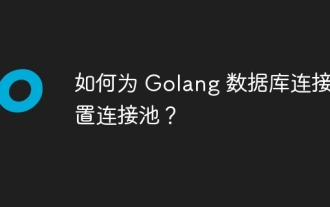
How to configure connection pool for Golang database connection?
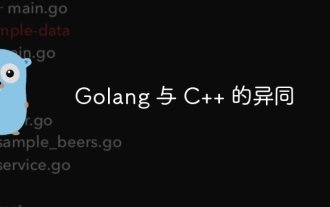
Similarities and Differences between Golang and C++
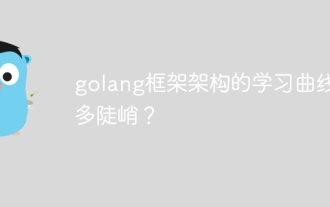
How steep is the learning curve of golang framework architecture?
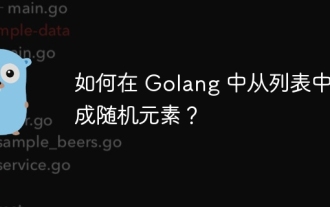
How to generate random elements from list in Golang?
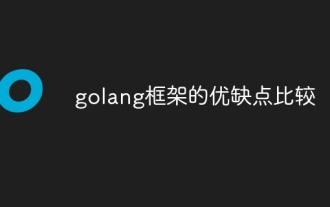
Comparison of advantages and disadvantages of golang framework

What are the best practices for error handling in Golang framework?
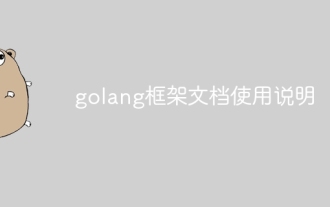
golang framework document usage instructions
