Golang framework automated testing common problems and solutions
Common problem: The test granularity is too large: break the test into smaller units. Testing is slow: Use parallel testing and data-driven testing. Test instability: use mocks and test fixtures to isolate tests. Insufficient test coverage: Use code coverage tools and mutate tests.
GoLang framework automated testing common problems and their solutions
Introduction
Automated testing is critical to ensuring software quality. In GoLang, there are various frameworks available for automated testing. However, there are some common problems that are often encountered when using these frameworks. This article explores these common problems and provides solutions.
Problem 1: The test granularity is too large
Problem:The test case is too large, making it difficult to maintain and debug.
Solution:
-
Break the test cases into smaller units, each unit testing a specific functionality of the application.
1
2
3
4
5
6
func TestAddNumbers(t *testing.T) {
result := AddNumbers(1, 2)
if
result != 3 {
t.Errorf(
"Expected 3, got %d"
, result)
}
}
Copy after login
Problem 2: Testing is slow
Problem:The test suite executes slowly, hindering development progress.
Solution:
Use parallel testing to run multiple test cases simultaneously.
1
2
3
4
5
6
7
8
9
import
"testing"
func TestAddNumbers(t *testing.T) {
t.Parallel()
result := AddNumbers(1, 2)
if
result != 3 {
t.Errorf(
"Expected 3, got %d"
, result)
}
}
Copy after loginUse data-driven testing to reduce code duplication.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
type AddNumbersTestData struct {
a int
b int
result int
}
func TestAddNumbers(t *testing.T) {
tests := []AddNumbersTestData{
{1, 2, 3},
{3, 4, 7},
}
for
_, test := range tests {
result := AddNumbers(test.a, test.b)
if
result != test.result {
t.Errorf(
"For a=%d, b=%d, expected %d, got %d"
, test.a, test.b, test.result, result)
}
}
}
Copy after loginProblem 3: The test is unstable
Problem: The test results are inconsistent, making debugging difficult.
Solution:
Use mocks and stubs to isolate tests and avoid the impact of external dependencies.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
type NumberGenerator
interface
{
Generate() int
}
type MockNumberGenerator struct {
numbers []int
}
func (m *MockNumberGenerator) Generate() int {
return
m.numbers[0]
}
func TestAddNumbersWithMock(t *testing.T) {
m := &MockNumberGenerator{[]int{1, 2}}
result := AddNumbers(m, m)
if
result != 3 {
t.Errorf(
"Expected 3, got %d"
, result)
}
}
Copy after loginSet up and tear down the test environment using test fixtures.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
import
"testing"
type TestFixture struct {
// Setup and teardown code
}
func TestAddNumbersWithFixture(t *testing.T) {
fixture := &TestFixture{}
t.Run(
"case 1"
, fixture.testFunc1)
t.Run(
"case 2"
, fixture.testFunc2)
}
func (f *TestFixture) testFunc1(t *testing.T) {
// ...
}
func (f *TestFixture) testFunc2(t *testing.T) {
// ...
}
Copy after login
Problem 4: Insufficient test coverage
Problem: The tests do not cover enough code paths of the application, Causing potential errors to be ignored.
Solution:
Use a code coverage tool to identify uncovered code.
1
2
3
4
5
6
7
8
9
10
11
12
import (
"testing"
"github.com/stretchr/testify/assert"
)
func TestAddNumbers(t *testing.T) {
assert.Equal(t, 3, AddNumbers(1, 2))
}
func TestCoverage(t *testing.T) {
// Coverage report generation code
}
Copy after loginUse mutate testing to generate variants of your program and execute tests to detect unexpected behavior.
1
2
3
4
5
6
7
8
9
10
import (
"testing"
"github.com/dvyukov/go-fuzz-corpus/fuzz"
)
func FuzzAddNumbers(f *fuzz.Fuzz) {
a := f.Intn(100)
b := f.Intn(100)
f.Check(AddNumbers(a, b))
}
Copy after login
The above is the detailed content of Golang framework automated testing common problems and solutions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
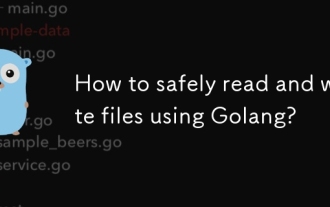
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
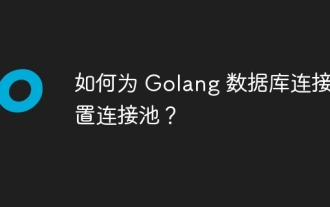
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
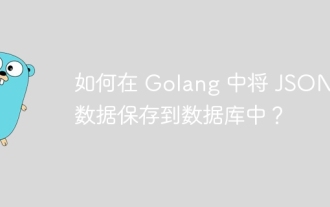
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
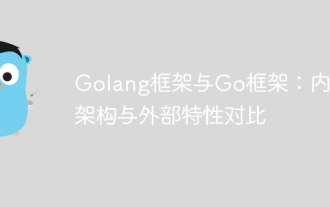
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
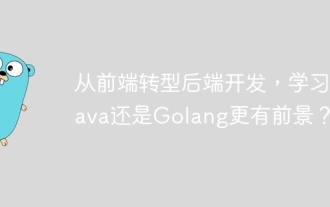
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
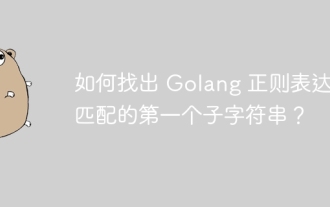
The FindStringSubmatch function finds the first substring matched by a regular expression: the function returns a slice containing the matching substring, with the first element being the entire matched string and subsequent elements being individual substrings. Code example: regexp.FindStringSubmatch(text,pattern) returns a slice of matching substrings. Practical case: It can be used to match the domain name in the email address, for example: email:="user@example.com", pattern:=@([^\s]+)$ to get the domain name match[1].
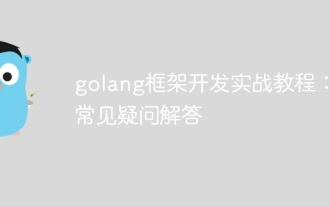
Go framework development FAQ: Framework selection: Depends on application requirements and developer preferences, such as Gin (API), Echo (extensible), Beego (ORM), Iris (performance). Installation and use: Use the gomod command to install, import the framework and use it. Database interaction: Use ORM libraries, such as gorm, to establish database connections and operations. Authentication and authorization: Use session management and authentication middleware such as gin-contrib/sessions. Practical case: Use the Gin framework to build a simple blog API that provides POST, GET and other functions.
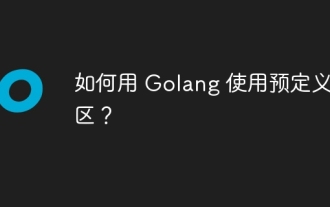
Using predefined time zones in Go includes the following steps: Import the "time" package. Load a specific time zone through the LoadLocation function. Use the loaded time zone in operations such as creating Time objects, parsing time strings, and performing date and time conversions. Compare dates using different time zones to illustrate the application of the predefined time zone feature.
