


Spring Boot3.x connects with Alibaba Cloud face recognition service to implement face recognition
This topic is dedicated to an in-depth discussion of how to implement efficient face detection and face recognition systems through the Spring Boot 3.x framework and the OpenCV library. Through 10 systematic articles, from basic concepts to advanced applications, combined with code examples and practical cases, we will gradually guide you to master the entire process of building a complete face detection and recognition system from scratch.
Alibaba Cloud face recognition service is an artificial intelligence service based on deep learning, which can provide functions such as face detection, face attribute analysis, and face comparison. Compared with other services, Alibaba Cloud has become the first choice of many enterprises in China due to its ultra-high accuracy, low latency, strong technical support and compliance. Its advantages include:
- High accuracy: Relying on Alibaba's strong artificial intelligence research capabilities, Alibaba Cloud's face recognition service has extremely high recognition accuracy.
- Low latency: Alibaba Cloud has many data centers in China, which can provide extremely low network latency.
- Technical support: Alibaba Cloud provides comprehensive technical support and rich documentation to help developers get started quickly.
- Compliance: Alibaba Cloud complies with domestic data privacy protection regulations to ensure data security.
Configure the Spring Boot project to interface with Alibaba Cloud face recognition service
First, we need to create an account for the face recognition service on Alibaba Cloud and obtain the API Key and Secret .
- Create an Alibaba Cloud account and obtain the API Key and Secret:
Log in to the Alibaba Cloud console, search for "Face Recognition Service" and activate the service.
Create a new AccessKey in "Access Control".
- Spring Boot project configuration:
Introduce dependencies: We need to add the dependencies of Alibaba Cloud SDK in pom.xml.
<dependency> <groupId>com.aliyun</groupId> <artifactId>aliyun-java-sdk-core</artifactId> <version>4.5.0</version> </dependency> <dependency> <groupId>com.aliyun</groupId> <artifactId>aliyun-java-sdk-facebody</artifactId> <version>2019-12-30</version> </dependency>
Configuration file
Add Alibaba Cloud related configurations in application.properties.
aliyun.accessKeyId=your_access_key_id aliyun.accessKeySecret=your_access_key_secret aliyun.regionId=cn-shanghai
Create a REST API to implement the face recognition function
Next, we create a REST API to receive images and call the Alibaba Cloud face recognition service.
Create the Spring Boot main class:
import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication public class FaceRecognitionApplication { public static void main(String[] args) { SpringApplication.run(FaceRecognitionApplication.class, args); } }
Configure the Alibaba Cloud face recognition client:
import com.aliyun.facebody20191230.Client; import com.aliyun.teaopenapi.models.Config; import org.springframework.beans.factory.annotation.Value; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; @Configuration public class AliyunConfig { @Value("${aliyun.accessKeyId}") private String accessKeyId; @Value("${aliyun.accessKeySecret}") private String accessKeySecret; @Value("${aliyun.regionId}") private String regionId; @Bean public Client faceClient() throws Exception { Config config = new Config() .setAccessKeyId(accessKeyId) .setAccessKeySecret(accessKeySecret); config.endpoint = "facebody." + regionId + ".aliyuncs.com"; return new Client(config); } }
Implement the face Recognized REST API:
import com.aliyun.facebody20191230.Client; import com.aliyun.facebody20191230.models.DetectFaceRequest; import com.aliyun.facebody20191230.models.DetectFaceResponse; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.http.ResponseEntity; import org.springframework.web.bind.annotation.*; import org.springframework.web.multipart.MultipartFile; import java.io.IOException; import java.util.Base64; @RestController @RequestMapping("/api/face") public class FaceRecognitionController { @Autowired private Client faceClient; @PostMapping("/detect") public ResponseEntity<String> detectFace(@RequestParam("image") MultipartFile image) throws IOException { byte[] imageBytes = image.getBytes(); String encodedImage = Base64.getEncoder().encodeToString(imageBytes); DetectFaceRequest request = new DetectFaceRequest() .setImageData(encodedImage); DetectFaceResponse response; try { response = faceClient.detectFace(request); } catch (Exception e) { return ResponseEntity.status(500).body("Error: " + e.getMessage()); } return ResponseEntity.ok(response.body.toString()); } }
The above code includes the following parts:
- Upload pictures: accept the pictures uploaded by the client and convert them into Base64 encoded for use by Alibaba Cloud API.
- Build the request: Create a DetectFaceRequest object and set the request parameters.
- Calling the API: Call the Alibaba Cloud face recognition API through the faceClient object and process the returned results.
Discuss the advantages and disadvantages of using Alibaba Cloud services and solutions to common problems
Advantages:
- Data privacy protection: Alibaba Cloud strictly abides by domestic data Privacy protection regulations ensure the security of user data.
- Low latency and high performance: With multiple data centers in China, Alibaba Cloud is able to provide extremely low network latency and high-performance services.
- Powerful technical support: Alibaba Cloud provides rich documentation and technical support to help developers solve various problems.
Disadvantages and solutions:
- API fees: Although Alibaba Cloud’s services are powerful, the corresponding fees are relatively high. It is recommended to choose an appropriate billing plan based on actual needs and conduct cost control.
- Usage restrictions: There are certain restrictions on the use of Alibaba Cloud APIs, such as call frequency limits. It is recommended to perform reasonable request offloading and optimization in high concurrency scenarios.
- Network problems: In some special circumstances, you may encounter network instability. It is recommended to use a retry mechanism and timeout settings to deal with this.
In summary, through the introduction and code examples of this article, I believe you have understood how to integrate the Alibaba Cloud face recognition service in the Spring Boot project and implement the face recognition function. At the same time, we also discussed the advantages and disadvantages of using Alibaba Cloud services and solutions to common problems, hoping to be helpful to everyone.
The above is the detailed content of Spring Boot3.x connects with Alibaba Cloud face recognition service to implement face recognition. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
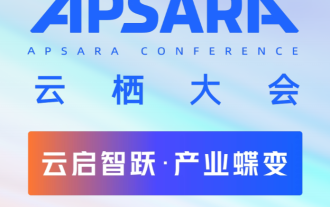
According to news from this website on August 5, Alibaba Cloud announced that the 2024 Yunqi Conference will be held in Yunqi Town, Hangzhou from September 19th to 21st. There will be a three-day main forum, 400 sub-forums and parallel topics, as well as nearly four Ten thousand square meters of exhibition area. Yunqi Conference is free and open to the public. From now on, the public can apply for free tickets through the official website of Yunqi Conference. An all-pass ticket of 5,000 yuan can be purchased. The ticket website is attached on this website: https://yunqi.aliyun.com/2024 /ticket-list According to reports, the Yunqi Conference originated in 2009 and was originally named the First China Website Development Forum. In 2011, it evolved into the Alibaba Cloud Developer Conference. In 2015, it was officially renamed the "Yunqi Conference" and has continued to successful move
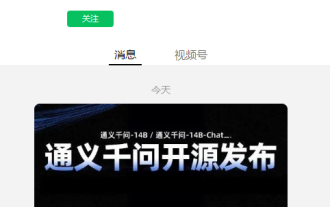
Alibaba Cloud today announced an open source project called Qwen-14B, which includes a parametric model and a conversation model. This open source project allows free commercial use. This site states: Alibaba Cloud has previously open sourced a parameter model Qwen-7B worth 7 billion. The download volume in more than a month has exceeded 1 million times. According to the data provided by Alibaba Cloud, Qwen -14B surpasses models of the same size in multiple authoritative evaluations, and some indicators are even close to Llama2-70B. According to reports, Qwen-14B is a high-performance open source model that supports multiple languages. Its overall training data exceeds 3 trillion Tokens, has stronger reasoning, cognition, planning and memory capabilities, and supports a maximum context window of 8k
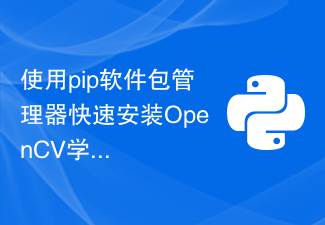
Use the pip command to easily install OpenCV tutorial, which requires specific code examples. OpenCV (OpenSource Computer Vision Library) is an open source computer vision library. It contains a large number of computer vision algorithms and functions, which can help developers quickly build image and video processing related applications. Before using OpenCV, we need to install it first. Fortunately, Python provides a powerful tool pip to manage third-party libraries

PHP study notes: Face recognition and image processing Preface: With the development of artificial intelligence technology, face recognition and image processing have become hot topics. In practical applications, face recognition and image processing are mostly used in security monitoring, face unlocking, card comparison, etc. As a commonly used server-side scripting language, PHP can also be used to implement functions related to face recognition and image processing. This article will take you through face recognition and image processing in PHP, with specific code examples. 1. Face recognition in PHP Face recognition is a
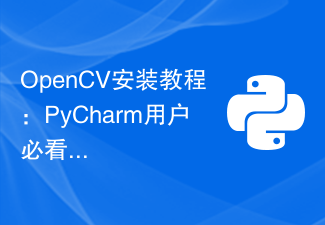
OpenCV is an open source library for computer vision and image processing, which is widely used in machine learning, image recognition, video processing and other fields. When developing using OpenCV, in order to better debug and run programs, many developers choose to use PyCharm, a powerful Python integrated development environment. This article will provide PyCharm users with an installation tutorial for OpenCV, with specific code examples. Step One: Install Python First, make sure you have Python installed
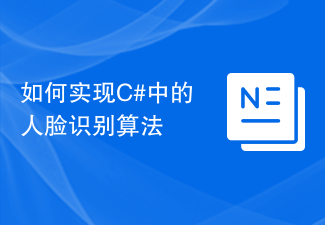
How to implement face recognition algorithm in C# Face recognition algorithm is an important research direction in the field of computer vision. It can be used to identify and verify faces, and is widely used in security monitoring, face payment, face unlocking and other fields. In this article, we will introduce how to use C# to implement the face recognition algorithm and provide specific code examples. The first step in implementing a face recognition algorithm is to obtain image data. In C#, we can use the EmguCV library (C# wrapper for OpenCV) to process images. First, we need to create the project
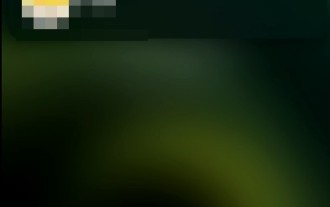
1. We can ask Siri before going to bed: Whose phone is this? Siri will automatically help us disable face recognition. 2. If you don’t want to disable it, you can turn on Face ID and choose to turn on [Require gaze to enable Face ID]. In this way, the lock screen can only be opened when we are watching.
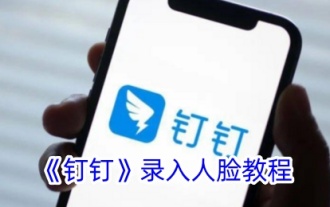
As an intelligent service software, DingTalk not only plays an important role in learning and work, but is also committed to improving user efficiency and solving problems through its powerful functions. With the continuous advancement of technology, facial recognition technology has gradually penetrated into our daily life and work. So how to use the DingTalk app for facial recognition entry? Below, the editor will bring you a detailed introduction. Users who want to know more about it can follow the pictures and text of this article! How to record faces on DingTalk? After opening the DingTalk software on your mobile phone, click "Workbench" at the bottom, then find "Attendance and Clock" and click to open. 2. Then click "Settings" on the lower right side of the attendance page to enter, and then click "My Settings" on the settings page to switch.
