The application of golang framework panic and recovery in debugging
Panic in the Go framework is used to raise unrecoverable exceptions, and Recover is used to recover from Panic and perform cleanup operations. They can handle exceptions such as database connection failures, ensuring application stability and user experience.
Use Go framework Panic and Recover in debugging
Panic and Recover are two key mechanisms for handling exceptions in the Go framework. During debugging, They can play a vital role.
Panic
Panic is a built-in method in Go used to raise exceptions. It will immediately terminate the currently running goroutine and print out the exception information. In general, panic should only be used when an unrecoverable error is encountered.
Usage scenarios:
- When the application encounters an internal error and cannot recover from the error.
- When the application encounters a violation of business logic or data inconsistency.
Syntax:
func(parameters) (result, parameters) { // 判断是否需要抛出异常 if (condition) { panic(reason) } return result, parameters }
Recover
Recover is used to recover from panic. It can obtain the exception information caused by panic and return to execution. process.
Usage scenarios:
- Catch panic in goroutine and perform necessary cleanup operations.
- Catch panics in web servers and display friendly error messages to users.
Syntax:
func(parameters) (result, parameters) { defer func() { if err := recover(); err != nil { // 处理 panic 异常 } }() return result, parameters }
Practical case
Consider a simple Go web server that uses panic to handle database connection failure errors:
package main import ( "database/sql" "fmt" "log" "net/http" ) func main() { // 连接数据库 db, err := sql.Open("mysql", "user:password@/database") if err != nil { // 数据库连接失败则抛出 panic panic(err) } // 启动 web 服务器 http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) { defer func() { if err := recover(); err != nil { // 捕获 panic 并在 Web 响应中显示错误消息 w.WriteHeader(http.StatusInternalServerError) w.Write([]byte("Internal error occurred. Please try again later.")) log.Printf("Panic occurred: %s", err) } }() // 访问数据库并执行查询 rows, err := db.Query("SELECT * FROM users") if err != nil { // 数据库查询失败则抛出 panic panic(err) } // 处理查询结果 // ... }) log.Fatal(http.ListenAndServe(":8080", nil)) }
In the above example, the Open()
function will connect to the database and return a sql.DB
instance. If the connection fails, the Open()
function will throw a panic. The main()
function uses defer
and recover()
to catch panic.
When the web server processes a request, the Query()
function queries the database and returns the query results. If the query fails, the Query()
function will throw a panic. recover()
The function will capture this panic and write the error message to the log.
By using panic and recover, applications can handle exceptions gracefully while providing users with friendly error messages. This is crucial to ensure application stability and improve user experience.
The above is the detailed content of The application of golang framework panic and recovery in debugging. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


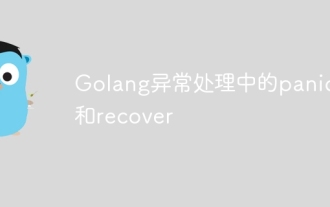
In Go, Panic and Recover are used for exception handling. Panic is used to report exceptions, and Recover is used to recover from exceptions. Panic will stop program execution and throw an exception value of type interface{}. Recover can catch exceptions from deferred functions or goroutines and return the exception value of type interface{} it throws.
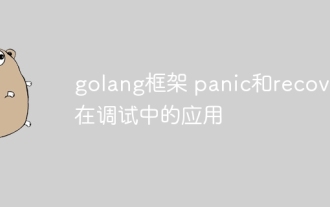
Panic in the Go framework is used to raise unrecoverable exceptions, and Recover is used to recover from Panic and perform cleanup operations. They can handle exceptions such as database connection failures, ensuring application stability and user experience.
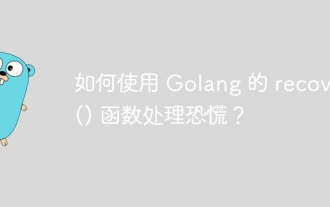
By using the recover() function, you can capture panics in the current function context, prevent program crashes and handle errors gracefully: recover() returns nil when a panic does not occur, and returns a panic when an uncaught panic occurs or when recovering from the function where the panic occurred value. Add defer callbacks around function calls to catch panics and perform custom handling, such as logging error information. recover() can only capture panics in the current function context, it will not cancel panics, and it only works on unhandled errors.
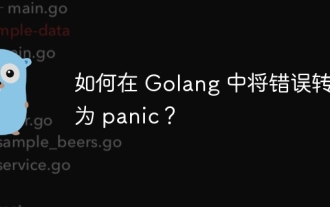
Yes, in Go it is possible to use the panic() function to convert an error into a panic, thus terminating the program immediately and returning the error stack.
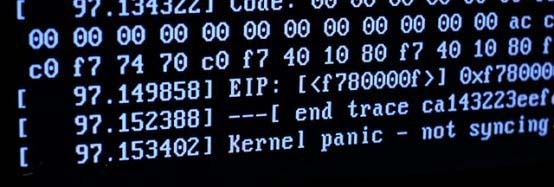
Thanks to the netizen Yuanyin Yuanyin of this website for his contribution. There is a reason for writing this article. In order to configure a completely silent boot, I performed improper mkinitcpio operations on Linux running on my work computer because I ignored a logic error in the mkinitcpio.conf file. This causes mkinitcpio to produce a new kernel file, but this kernel file does not work properly. When restarting, the kernel startup aborts in the Panic state. Under normal circumstances, when the new kernel does not work properly, you can temporarily start the system by using the fallback version of the initramfs kernel file, or even directly overwrite the fallback version back to roll back the changes, but this time
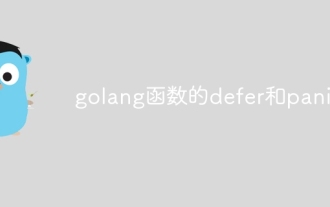
The defer and panic keywords are used to control exceptions and post-processing: defer: push the function onto the stack and execute it after the function returns. It is often used to release resources. Panic: Throws an exception to interrupt program execution and is used to handle serious errors that cannot continue running. The difference: defer is only executed when the function returns normally, while panic is executed under any circumstances, even if an error occurs.
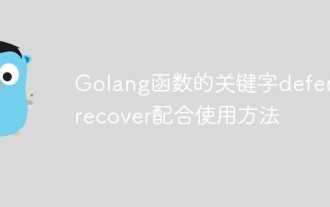
Golang is an efficient, concise and easy-to-learn programming language that was originally developed by Google and first released in 2009. It is designed to improve programmer productivity and code clarity. In Golang, the function keywords defer and recover are often used together to handle errors that may occur in the program. This article will introduce the use of these two keywords and illustrate their practical application through some examples. 1. How to use defer defer is a keyword
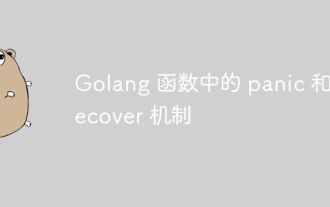
The panic function raises an exception and terminates the current function, and the recover function captures the exception caused by panic. In Golang, the panic and recover functions are used to handle errors and exceptions in the program. panic is used to raise exceptions and bubble up. recover is used to catch exceptions and return the panic value. If recover successfully catches the exception, the program will not crash. Instead, code execution continues.
