How to create parallel tasks using pipelines in Go?
Pipeline is an unbuffered communication mechanism that can be used to create parallel tasks: Create a pipeline: ch := make(chan int) Send data: ch <- iReceive data: for v := range ch { fmt.Println (v) }
How to use pipelines in the Go language to create parallel tasks
Pipelines are an unbuffered communication mechanism used in the Go protocol transfer data between processes. Pipes can be used to create parallel tasks to process multiple inputs or outputs simultaneously.
Create a pipe
Use the make
function to create a pipe and specify the type of value to be sent or received:
var ch chan int ch = make(chan int)
Send data
Use the <-
operator to send data to the pipe:
go func() { for i := 1; i <= 5; i++ { ch <- i } }()
Receive data
Use the <-
operator to receive data from the pipe :
for v := range ch { fmt.Println(v) }
Practical Example: Sum
The following example demonstrates how to use pipelines to create parallel tasks to calculate the sum of a bunch of numbers:
package main import ( "fmt" "time" ) func main() { ch := make(chan int) go func() { sum := 0 for i := 1; i <= 1000000; i++ { sum += i } ch <- sum }() result := <-ch fmt.Println("The sum is:", result) }
In this example, a The coroutine is responsible for calculating the sum and sending it to the pipeline. The main coroutine receives the results from the pipe and prints them.
This is just a simple example of using pipelines to create parallel tasks. It can be used in various scenarios, such as:
- Multiple coroutines processing data
- Distributed computing
- Event processing
The above is the detailed content of How to create parallel tasks using pipelines in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
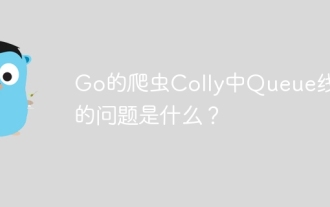
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
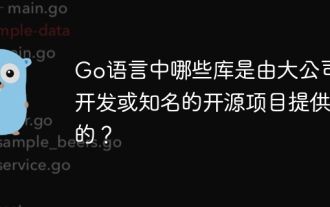
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
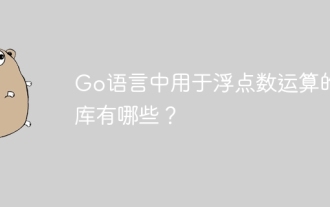
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
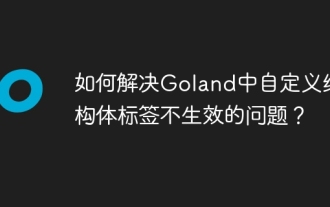
Regarding the problem of custom structure tags in Goland When using Goland for Go language development, you often encounter some configuration problems. One of them is...
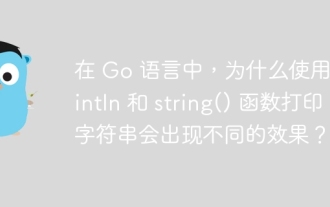
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
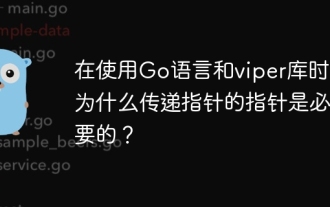
Go pointer syntax and addressing problems in the use of viper library When programming in Go language, it is crucial to understand the syntax and usage of pointers, especially in...
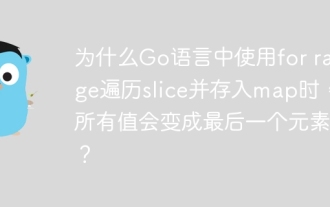
Why does map iteration in Go cause all values to become the last element? In Go language, when faced with some interview questions, you often encounter maps...
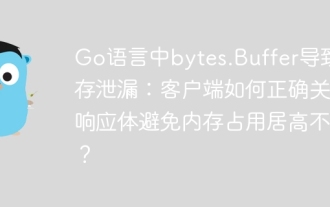
Analysis of memory leaks caused by bytes.makeSlice in Go language In Go language development, if the bytes.Buffer is used to splice strings, if the processing is not done properly...
