


Security and synchronization mechanism of Golang functions in concurrent environment
Function safety: goroutine safety: safe to call in concurrent goroutine. Non-goroutine safe: accessing shared state or relying on a specific goroutine. Synchronization mechanism: Mutex: protects concurrent access to shared resources. RWMutex: Allows concurrent reading and only one write. Cond: Wait for specific conditions to be met. WaitGroup: Wait for a group of goroutines to complete. Practical case: Concurrency counter uses Mutex to protect shared state and ensure correctness under concurrency.
The security and synchronization mechanism of Go functions in a concurrent environment
Understand the safety of functions in a concurrent environment of Go stability and correct synchronization mechanisms are crucial. This article will explore these concepts and demonstrate them through a practical case.
Function safety
- goroutine safety: If a function can be safely called in concurrent goroutines, it is called goroutine safety of. This means it does not modify global variables or shared state, and it does not compete with other goroutines.
- Non-goroutine safe: A function is non-goroutine safe if it accesses or modifies shared state, or relies on a specific goroutine being running.
Synchronization mechanism
In order to ensure data consistency and avoid competition in a concurrent environment, a synchronization mechanism needs to be used. Go provides several built-in synchronization types:
- Mutex: Mutex, used to protect concurrent access to shared resources.
- RWMutex: Read and write mutex, allowing concurrent reading but only writing at one time.
- Cond: Condition variable, used to wait for specific conditions to be met.
- WaitGroup: Waiting group, used to wait for a group of goroutines to complete.
Practical case: Concurrency counter
Consider an example of a concurrency counter. It is a value stored in a goroutine safe variable that can be incremented in parallel. In order to ensure the correctness of the counter under concurrency, a synchronization mechanism needs to be used.
package main import ( "fmt" "sync" "sync/atomic" ) var ( cnt int64 // 原子计数器 mu sync.Mutex // 互斥锁 ) func main() { wg := &sync.WaitGroup{} // 并发增量计数器 for i := 0; i < 10; i++ { wg.Add(1) go func() { mu.Lock() cnt++ mu.Unlock() wg.Done() }() } wg.Wait() fmt.Println("最终计数:", cnt) }
In this example, we declare the counter cnt
as an atomic variable to ensure safe incrementation for concurrency. Use mutex mu
to protect concurrent access to cnt
to prevent race conditions.
Running this program will output:
最终计数: 10
This confirms that the counter is correctly incremented 10 times in parallel.
The above is the detailed content of Security and synchronization mechanism of Golang functions in concurrent environment. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
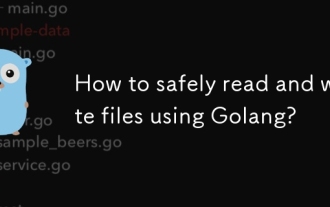
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
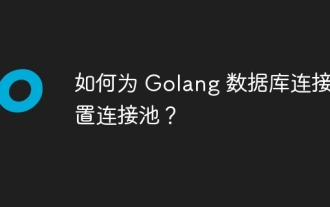
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
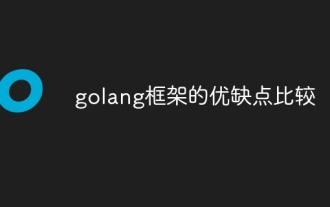
The Go framework stands out due to its high performance and concurrency advantages, but it also has some disadvantages, such as being relatively new, having a small developer ecosystem, and lacking some features. Additionally, rapid changes and learning curves can vary from framework to framework. The Gin framework is a popular choice for building RESTful APIs due to its efficient routing, built-in JSON support, and powerful error handling.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
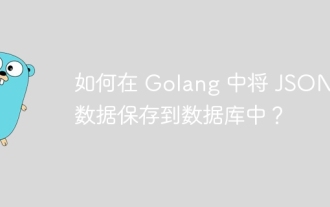
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
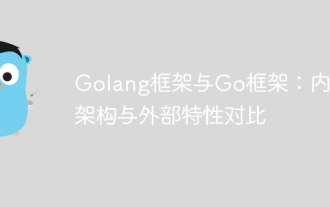
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
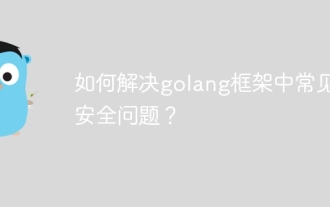
How to address common security issues in the Go framework With the widespread adoption of the Go framework in web development, ensuring its security is crucial. The following is a practical guide to solving common security problems, with sample code: 1. SQL Injection Use prepared statements or parameterized queries to prevent SQL injection attacks. For example: constquery="SELECT*FROMusersWHEREusername=?"stmt,err:=db.Prepare(query)iferr!=nil{//Handleerror}err=stmt.QueryR
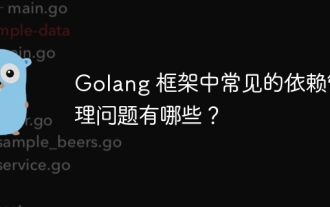
Common problems and solutions in Go framework dependency management: Dependency conflicts: Use dependency management tools, specify the accepted version range, and check for dependency conflicts. Vendor lock-in: Resolved by code duplication, GoModulesV2 file locking, or regular cleaning of the vendor directory. Security vulnerabilities: Use security auditing tools, choose reputable providers, monitor security bulletins and keep dependencies updated.
