How to debug Golang database connection?
Use the log package and pprof tool to debug database connection problems in the Go language. log only logs connection attempts and errors, while pprof generates a more in-depth performance analysis report that can be used to pinpoint database-related functionality. Demonstrated through practical cases, these methods can quickly resolve common database connection errors, including file non-existence and connection refused.
How to debug Golang database connections
In Go, debugging database connection issues is a common task. This article will guide you on how to use debugging techniques to pinpoint and resolve database connection-related issues.
Using the log
package
One of the simplest methods is to use the log
package to log database connection attempts and encounters Any errors encountered:
import ( "context" "fmt" "log" "github.com/jackc/pgx/v4" ) func main() { conn, err := pgx.Connect(context.Background(), "user=postgres password=secret dbname=my-database") if err != nil { log.Fatalf("连接到数据库失败: %v", err) } _ = conn // 防止编译器抱怨未使用变量 }
Run this code and if any connection errors are encountered, they will be printed to the standard output in red.
Using the pprof
tool
For more in-depth debugging, you can use the pprof
tool, which can generate a performance analysis Report.
To use pprof
, first compile your program and enable debugging information:
go build -gcflags="all=-N -l"
Then, follow these steps:
- Run your program, then terminate it when it exits with an error.
- Use
pprof
to analyze the heap dump:pprof x :.pprof
- Type ## in the
pprof
interactive shell #top, find the functions related to database connection.
Practical Case
Let us illustrate how to use these methods through a practical case.Error: no such file or directory
Error message:
setupConnect: sql: open /Users/myuser/go/bin/data/secrets.db: no such file or directory
Debugging:
Using thelog package, we can see that the connection attempt failed when opening the file containing the database credentials. Check whether the file path is correct or make sure the file exists.
Error: dial tcp 127.0.0.1:5432: connect: connection refused
Error message:
setupConnect: dial tcp 127.0.0.1:5432: connect: connection refused
Debug:
Usingpprof we can see that the connection attempt is trying to connect to port# on
127.0.0.1 ##5432
, but failed. Just check whether the database server is running and whether the port is correct. By using these debugging techniques, you can quickly pinpoint and resolve database connection issues, making your Golang applications more reliable.
The above is the detailed content of How to debug Golang database connection?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
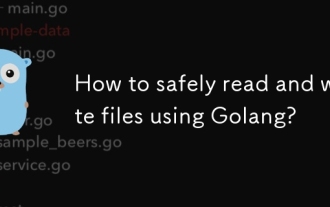
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
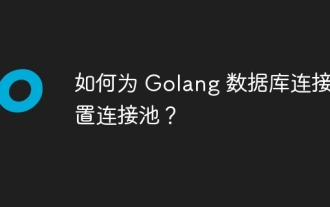
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
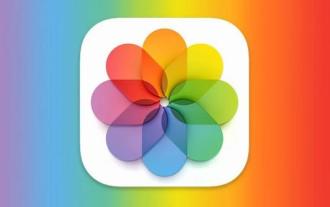
Apple's latest releases of iOS18, iPadOS18 and macOS Sequoia systems have added an important feature to the Photos application, designed to help users easily recover photos and videos lost or damaged due to various reasons. The new feature introduces an album called "Recovered" in the Tools section of the Photos app that will automatically appear when a user has pictures or videos on their device that are not part of their photo library. The emergence of the "Recovered" album provides a solution for photos and videos lost due to database corruption, the camera application not saving to the photo library correctly, or a third-party application managing the photo library. Users only need a few simple steps
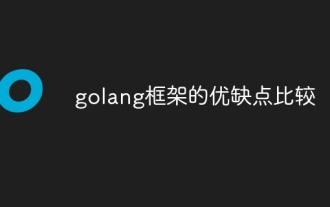
The Go framework stands out due to its high performance and concurrency advantages, but it also has some disadvantages, such as being relatively new, having a small developer ecosystem, and lacking some features. Additionally, rapid changes and learning curves can vary from framework to framework. The Gin framework is a popular choice for building RESTful APIs due to its efficient routing, built-in JSON support, and powerful error handling.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
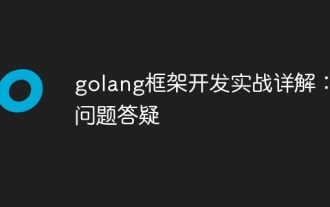
In Go framework development, common challenges and their solutions are: Error handling: Use the errors package for management, and use middleware to centrally handle errors. Authentication and authorization: Integrate third-party libraries and create custom middleware to check credentials. Concurrency processing: Use goroutines, mutexes, and channels to control resource access. Unit testing: Use gotest packages, mocks, and stubs for isolation, and code coverage tools to ensure sufficiency. Deployment and monitoring: Use Docker containers to package deployments, set up data backups, and track performance and errors with logging and monitoring tools.
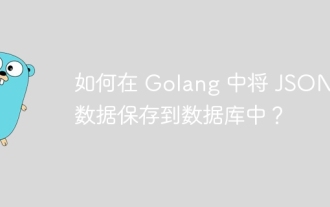
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
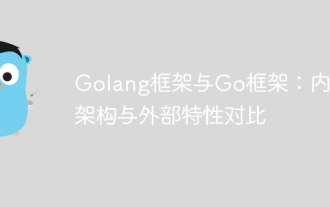
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
