Common problems and solutions in asynchronous programming in Java framework
3 common problems and solutions in asynchronous programming in Java framework: Callback Hell: Use Promise or CompletableFuture to manage callbacks in a more intuitive style. Resource contention: Use synchronization primitives (such as locks) to protect shared resources, and consider using thread-safe collections (such as ConcurrentHashMap). Unhandled exceptions: Explicitly handle exceptions in tasks and use an exception handling framework (such as CompletableFuture.exceptionally()) to handle exceptions.
Common problems and solutions in asynchronous programming in Java framework
Using Java framework for asynchronous programming is powerful and efficient, but It can also bring about some common problems. This article will explore these issues and provide effective solutions to help you avoid pitfalls and keep your code simple and efficient.
Problem 1: Callback Hell
Asynchronous operations usually use callbacks to process results. When multiple asynchronous calls are nested, it results in "callback hell" that is difficult to read and maintain.
Solution:
- Use Promise or CompletableFuture to provide a more intuitive asynchronous programming style.
- Wrap callbacks into functions to keep the code structure clear.
For example:
CompletableFuture<String> future = doSomethingAsync(); future.thenApply(result -> doSomethingElse(result));
Question 2: Resource contention
Asynchronous operations run on multiple threads, May lead to resource competition. For example, concurrent writes to shared variables can lead to inconsistent data.
Solution:
- Use synchronization primitives (such as locks and atomic variables) to protect shared resources.
- Consider using a thread-safe collection such as ConcurrentHashMap.
Issue 3: Unhandled exceptions
Exceptions in asynchronous operations may be ignored, causing program crashes or undesired behavior.
Solution:
- Handle the exception explicitly in the task and pass it to the caller.
- Use an exception handling framework, such as Java's
CompletableFuture.exceptionally()
method, to handle exceptions.
Practical case:
Consider a simple e-commerce application where users can add to a shopping cart asynchronously.
// 定义回调处理添加到购物车操作的响应 void addToCartCallback(Cart cart, Throwable error) { if (error != null) { // 处理错误 } else { // 处理成功添加物品到购物车 } } // 发送异步请求添加到购物车 doAddToCartAsync(item, addToCartCallback);
By using callbacks, we avoid blocking the main thread and can handle the response asynchronously when the request completes. To avoid callback hell, you can wrap the callback into a function as follows:
void addToCart(Item item) { doAddToCartAsync(item, addToCartCallback(item)); }
By implementing these best practices, you can significantly reduce common problems in asynchronous programming in Java frameworks and write robust, reliable Maintained code.
The above is the detailed content of Common problems and solutions in asynchronous programming in Java framework. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


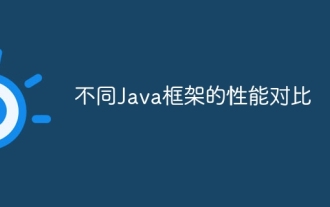
Performance comparison of different Java frameworks: REST API request processing: Vert.x is the best, with a request rate of 2 times SpringBoot and 3 times Dropwizard. Database query: SpringBoot's HibernateORM is better than Vert.x and Dropwizard's ORM. Caching operations: Vert.x's Hazelcast client is superior to SpringBoot and Dropwizard's caching mechanisms. Suitable framework: Choose according to application requirements. Vert.x is suitable for high-performance web services, SpringBoot is suitable for data-intensive applications, and Dropwizard is suitable for microservice architecture.
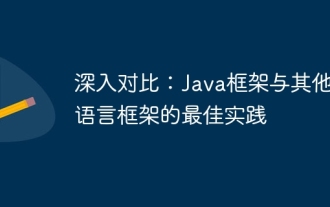
Java frameworks are suitable for projects where cross-platform, stability and scalability are crucial. For Java projects, Spring Framework is used for dependency injection and aspect-oriented programming, and best practices include using SpringBean and SpringBeanFactory. Hibernate is used for object-relational mapping, and best practice is to use HQL for complex queries. JakartaEE is used for enterprise application development, and the best practice is to use EJB for distributed business logic.
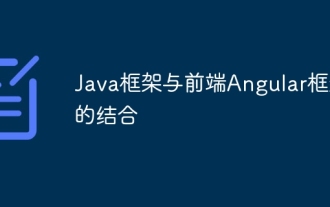
Answer: Java backend framework and Angular frontend framework can be integrated to provide a powerful combination for building modern web applications. Steps: Create Java backend project, select SpringWeb and SpringDataJPA dependencies. Define model and repository interfaces. Create a REST controller and provide endpoints. Create an Angular project. Add SpringBootJava dependency. Configure CORS. Integrate Angular in Angular components.
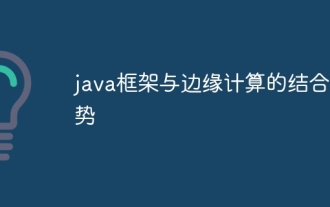
Java frameworks are combined with edge computing to enable innovative applications. They create new opportunities for the Internet of Things, smart cities and other fields by reducing latency, improving data security, and optimizing costs. The main integration steps include selecting an edge computing platform, deploying Java applications, managing edge devices, and cloud integration. Benefits of this combination include reduced latency, data localization, cost optimization, scalability and resiliency.
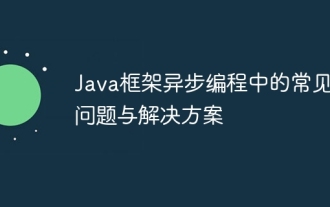
3 common problems and solutions in asynchronous programming in Java frameworks: Callback Hell: Use Promise or CompletableFuture to manage callbacks in a more intuitive style. Resource contention: Use synchronization primitives (such as locks) to protect shared resources, and consider using thread-safe collections (such as ConcurrentHashMap). Unhandled exceptions: Explicitly handle exceptions in tasks and use an exception handling framework (such as CompletableFuture.exceptionally()) to handle exceptions.
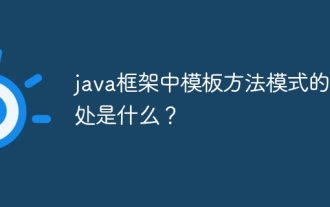
The Template Method pattern defines an algorithm framework with specific steps implemented by subclasses. Its advantages include extensibility, code reuse, and consistency. In a practical case, the beverage production framework uses this pattern to create customizable beverage production algorithms, including coffee and tea classes, which can customize brewing and flavoring steps while maintaining consistency.
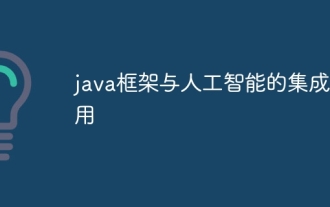
Java frameworks integrated with AI enable applications to take advantage of AI technologies, including automating tasks, delivering personalized experiences, and supporting decision-making. By directly calling or using third-party libraries, the Java framework can be seamlessly integrated with frameworks such as H2O.ai and Weka to achieve functions such as data analysis, predictive modeling, and neural network training, and be used for practical applications such as personalized product recommendations.
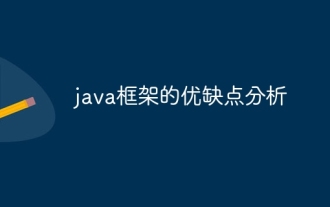
The Java framework provides predefined components with the following advantages and disadvantages: Advantages: code reusability, modularity, testability, security, and versatility. Disadvantages: Learning curve, performance overhead, limitations, complexity, and vendor lock-in.
