


Connection differences between different database management systems in PHP
Differences in connection between different DBMS in PHP: MySQL: Using the mysqli extension, host name, user name, and password are required. PostgreSQL: Using the PDO extension, use the pgsql clause to specify the hostname, port, and database name. MongoDB: Use the MongoDB client library, specifying the hostname and port. By understanding these differences, developers can establish reliable database connections based on their specific DBMS.
Connection differences between different database management systems in PHP
PHP is a powerful server-side programming language. Allows developers to connect to various database management systems (DBMS) to store and retrieve data. Although the basic steps of the join process are generally similar, there are some key differences depending on the specific DBMS being used.
Connect to MySQL
<?php $servername = "localhost"; $username = "username"; $password = "password"; // 创建一个 MySQL 连接 $conn = new mysqli($servername, $username, $password); // 检查连接 if ($conn->connect_error) { die("连接失败:" . $conn->connect_error); } ?>
Connect to PostgreSQL
<?php $servername = "localhost"; $username = "username"; $password = "password"; $dbname = "database_name"; // 创建一个 PostgreSQL 连接 $conn = new PDO("pgsql:host=$servername;port=5432;dbname=$dbname", $username, $password); // 检查连接 if (!$conn) { die("连接失败:" . pg_last_error()); } ?>
Connect to MongoDB
<?php $servername = "localhost"; $port = 27017; $dbname = "database_name"; // 创建一个 MongoDB 连接 $client = new MongoDB\Client("mongodb://localhost:27017"); // 选择数据库 $db = $client->selectDatabase($dbname); ?>
Practical case: Retrieving data using MySQL
<?php // 创建一个 MySQL 连接 $conn = new mysqli($servername, $username, $password); // 准备一个 SQL 查询 $sql = "SELECT * FROM employees"; // 执行查询 $result = $conn->query($sql); // 如果查询成功,则遍历结果 if ($result->num_rows > 0) { while($row = $result->fetch_assoc()) { echo "员工姓名: " . $row["first_name"] . " " . $row["last_name"] . "<br>"; } } else { echo "没有记录找到"; } ?>
By understanding the connection differences of different DBMS, developers can establish reliable and efficient database connections in PHP to access, store and manipulate data .
The above is the detailed content of Connection differences between different database management systems in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
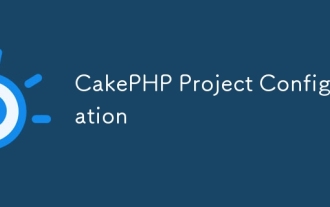
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
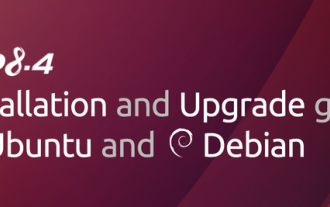
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
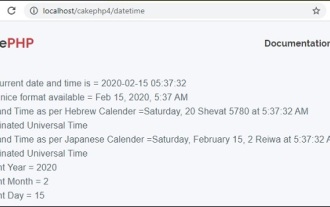
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
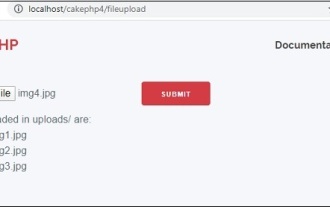
To work on file upload we are going to use the form helper. Here, is an example for file upload.
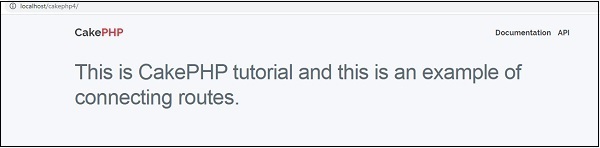
In this chapter, we are going to learn the following topics related to routing ?
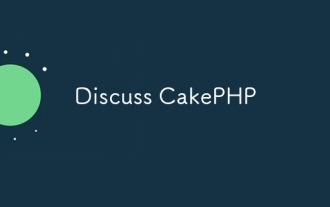
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
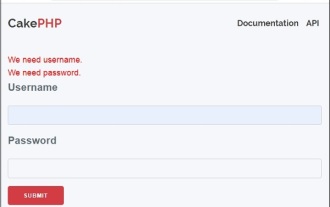
Validator can be created by adding the following two lines in the controller.
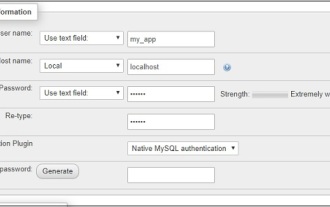
Working with database in CakePHP is very easy. We will understand the CRUD (Create, Read, Update, Delete) operations in this chapter.
