The key role of function pointers in C++ code extensibility
The key role of function pointers in C++ code is to improve extensibility and allow functions to be called without specifying the function name. Its usage includes declaring, allocating, and calling function pointers. Function pointers play a vital role in sorting algorithms by passing different comparators to implement multiple sorting methods. This makes C++ code more flexible and reusable, greatly improving code quality.
The key role of function pointers in C++ code extensibility
A function pointer is a pointer to a function that allows Call a function without specifying a function name. This is useful when highly flexible and scalable code is required.
Usage
To declare a function pointer, use the following syntax:
type (*function_pointer_name)(arguments);
For example, declare a pointer to a function that takes two int parameters and returns an int Pointer to a function:
int (*func_ptr)(int, int);
To assign a function pointer to a function, use the function address operator (&):
func_ptr = &my_function;
Calling a function pointer is like calling a normal function:
int result = func_ptr(1, 2);
Practical case
Sort algorithm
Consider a sorting algorithm that needs to sort an array based on a specific comparator. Using function pointers, you can easily pass different comparators to the sorting algorithm, allowing for multiple sorting methods.
typedef int (*Comparator)(const int&, const int&); void Sort(int* array, int size, Comparator comparator) { for (int i = 0; i < size; ++i) { for (int j = i + 1; j < size; ++j) { if (comparator(array[i], array[j])) { swap(array[i], array[j]); } } } } int Ascending(const int& a, const int& b) { return a < b; } int Descending(const int& a, const int& b) { return a > b; } int main() { int array[] = {5, 2, 8, 3, 1}; int size = sizeof(array) / sizeof(array[0]); Sort(array, size, Ascending); for (int num : array) { cout << num << " "; } cout << endl; Sort(array, size, Descending); for (int num : array) { cout << num << " "; } return 0; }
In this example, the Sort
function accepts a Comparator
function pointer that defines how to compare two elements. The Ascending
and Descending
functions are two comparators used to sort arrays in ascending and descending order respectively.
Conclusion
Function pointers are an extensibility tool in C++ that can greatly improve code quality by making the code more flexible and reusable. By understanding the use of function pointers, you can take full advantage of the power of C++ to build complex and maintainable applications.
The above is the detailed content of The key role of function pointers in C++ code extensibility. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


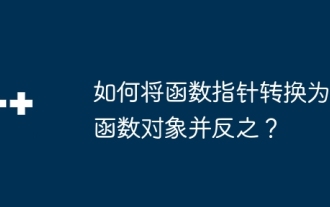
In C++, function pointers can be converted into function objects through the std::function template: use std::function to wrap function pointers into function objects. Use the std::function::target member function to convert a function object to a function pointer. This transformation is useful in scenarios such as event handling, function callbacks, and generic algorithms, providing greater flexibility and code reusability.
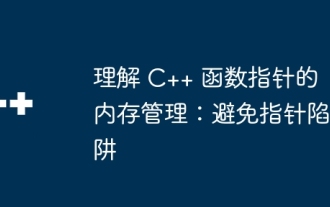
When using function pointers in C++, memory management must be carefully considered to avoid pitfalls. These traps include dangling pointers (pointing to functions outside their scope) and wild pointers (function pointers that are never initialized or set to nullptr). To avoid these pitfalls, follow these best practices: always initialize function pointers, manage memory carefully, and use smart pointers. This way you can use function pointers safely and avoid falling into pointer traps.
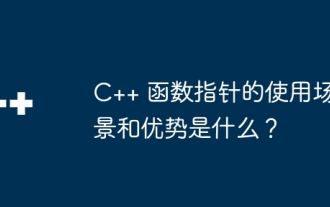
Function pointers allow storing references to functions, providing additional flexibility. Usage scenarios include event processing, algorithm sorting, data transformation and dynamic polymorphism. Benefits include flexibility, decoupling, code reuse, and performance optimization. Practical applications include event processing, algorithmic sorting, and data transformation. With function pointers, C++ programmers can create flexible and dynamic code.
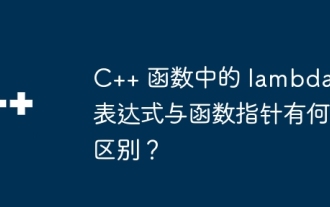
Lambda expressions and function pointers are both mechanisms for encapsulating code in C++, but they differ in implementation and characteristics: Implementation: function pointers point to the memory address of the function, while lambda expressions are inline anonymous code blocks. Return type: The return type of a function pointer is fixed, while the return type of a Lambda expression is determined by its body code block. Variable capture: Function pointers cannot capture external variables, but Lambda expressions can capture external variables by reference or value through the [&] or [=] keywords. Syntax: Use asterisks (*) for function pointers and square brackets ([]) for lambda expressions.
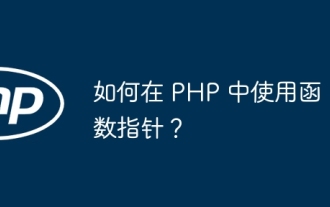
In PHP, a function pointer is a variable called a callback function that points to the function address. It allows dynamic processing of functions: Syntax: $functionPointer='function_name' Practical example: Perform operations on arrays: usort($numbers,'sortAscending') as function parameters: array_map(function($string){...},$strings )Note: The function pointer points to the function name, which must match the specified type and ensure that the pointed function always exists.
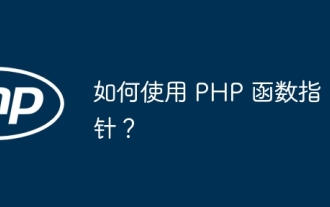
PHP function pointers allow functions to be passed as arguments and can be used to create callback functions or reuse code. Syntax: $functionPointer=function_name; or anonymous function: $functionPointer=function($arg1,$arg2){...}; call the function pointer through call_user_func($function,$a,$b), for example, the applyFunction() function receives function pointer parameter and use call_user_func() to call the function. Note: The function pointer must be a valid function or an anonymous function; it cannot point to a private method; it will be generated if the function does not exist
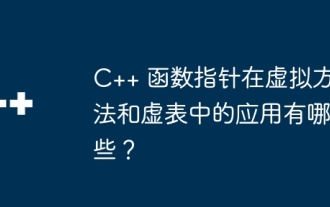
C++ function pointers are used in virtual methods to store pointers to overridden method implementations in derived classes, and in virtual tables to initialize virtual tables and store pointers to virtual method implementations, thereby achieving runtime polymorphism and allowing derived classes to override Virtual methods in the base class and call the correct implementation based on the actual type of the object at runtime.
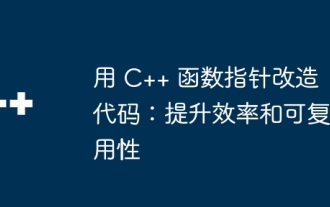
Function pointer technology can improve code efficiency and reusability, specifically as follows: Improved efficiency: Using function pointers can reduce repeated code and optimize the calling process. Improve reusability: Function pointers allow the use of general functions to process different data, improving program reusability.
