How to close a file using C++?
There are two ways to close a C++ file: using the fclose() function (applicable to C stream files) and using the close() member function of the ifstream and ofstream classes (applicable to C++ standard library file streams). These methods ensure that the file is closed before the program ends to avoid resource leaks, and the close() member function can automatically close the file, while fclose() requires an explicit call.
How to close a file using C++
File manipulation is a common task in programming. In C++, there are several ways to close a file. This article will detail the method of closing C++ files and provide a practical case to demonstrate how to use it.
Method 1: Use the fclose() function
fclose()
is a standard C function used to close a file. It accepts a FILE* type pointer as parameter, pointing to the file to be closed. The syntax is as follows:
int fclose(FILE *stream);
Method 2: Use the close() member function of the ifstream and ofstream classes
ifstream
and # in the C++ standard library The ##ofstream class provides the
close() member function for closing the file. The syntax is as follows:
void close();
Practical case
The following code example demonstrates how to use thefclose() function to close a file:
#include <stdio.h> int main() { // 打开一个文件 FILE *file = fopen("test.txt", "w"); // ... 在文件中写入数据 ... // 关闭文件 int result = fclose(file); // 检查是否关闭成功 if (result != 0) { printf("无法关闭文件!\n"); return 1; } printf("文件已成功关闭。\n"); return 0; }
close() member function of the
ifstream class to close a file:
#include <iostream> #include <fstream> int main() { // 打开一个文件 std::ifstream file("test.txt"); // ... 从文件中读取数据 ... // 关闭文件 file.close(); // 检查是否关闭成功 if (!file.is_open()) { std::cout << "文件已成功关闭。" << std::endl; } else { std::cout << "无法关闭文件!" << std::endl; } return 0; }
Note:
- Make sure to close all files before the program ends to avoid resource leaks.
- For C stream files, please use the
- fclose()
function to explicitly close.
For - ifstream
and
ofstreamobjects, automatic closing can be done using the destructor, but explicit closing ensures that the file is closed as quickly as possible.
The above is the detailed content of How to close a file using C++?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


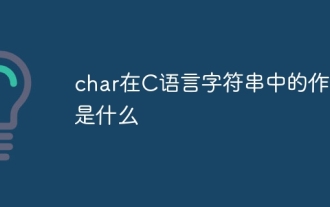
In C, the char type is used in strings: 1. Store a single character; 2. Use an array to represent a string and end with a null terminator; 3. Operate through a string operation function; 4. Read or output a string from the keyboard.
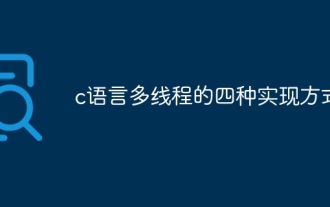
Multithreading in the language can greatly improve program efficiency. There are four main ways to implement multithreading in C language: Create independent processes: Create multiple independently running processes, each process has its own memory space. Pseudo-multithreading: Create multiple execution streams in a process that share the same memory space and execute alternately. Multi-threaded library: Use multi-threaded libraries such as pthreads to create and manage threads, providing rich thread operation functions. Coroutine: A lightweight multi-threaded implementation that divides tasks into small subtasks and executes them in turn.
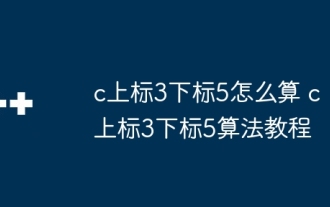
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
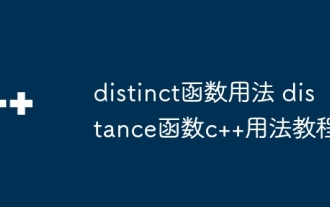
std::unique removes adjacent duplicate elements in the container and moves them to the end, returning an iterator pointing to the first duplicate element. std::distance calculates the distance between two iterators, that is, the number of elements they point to. These two functions are useful for optimizing code and improving efficiency, but there are also some pitfalls to be paid attention to, such as: std::unique only deals with adjacent duplicate elements. std::distance is less efficient when dealing with non-random access iterators. By mastering these features and best practices, you can fully utilize the power of these two functions.
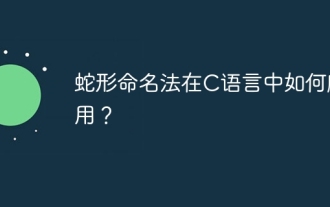
In C language, snake nomenclature is a coding style convention, which uses underscores to connect multiple words to form variable names or function names to enhance readability. Although it won't affect compilation and operation, lengthy naming, IDE support issues, and historical baggage need to be considered.
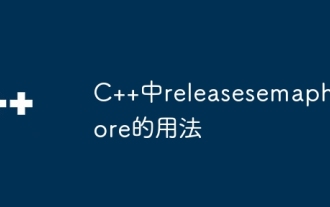
The release_semaphore function in C is used to release the obtained semaphore so that other threads or processes can access shared resources. It increases the semaphore count by 1, allowing the blocking thread to continue execution.
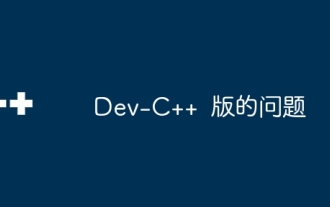
Dev-C 4.9.9.2 Compilation Errors and Solutions When compiling programs in Windows 11 system using Dev-C 4.9.9.2, the compiler record pane may display the following error message: gcc.exe:internalerror:aborted(programcollect2)pleasesubmitafullbugreport.seeforinstructions. Although the final "compilation is successful", the actual program cannot run and an error message "original code archive cannot be compiled" pops up. This is usually because the linker collects
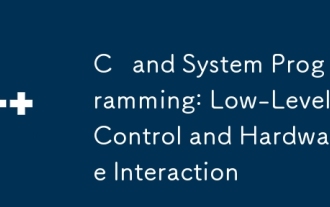
C is suitable for system programming and hardware interaction because it provides control capabilities close to hardware and powerful features of object-oriented programming. 1)C Through low-level features such as pointer, memory management and bit operation, efficient system-level operation can be achieved. 2) Hardware interaction is implemented through device drivers, and C can write these drivers to handle communication with hardware devices.
