How to implement multi-threaded programming in C++ using STL?
Using STL to implement multi-threaded programming in C++ involves: using std::thread to create threads. Protect shared resources using std::mutex and std::lock_guard. Use std::condition_variable to coordinate conditions between threads. This method supports concurrent tasks such as file copying, where multiple threads process file blocks in parallel.
How to use STL to implement multi-threaded programming in C++
STL (Standard Template Library) provides a powerful set of Concurrency primitives and containers can easily implement multi-threaded programming. This article demonstrates how to use key components in STL to create multi-threaded applications.
Using Threads
To create a thread, use std::thread
Class:
std::thread t1(some_function); t1.join(); // 等待线程完成
some_function
is a function to be executed concurrently.
Mutexes and locks
Mutexes can be used to prevent multiple threads from accessing shared resources at the same time. Using std::mutex
:
std::mutex m; { std::lock_guard<std::mutex> lock(m); // 在此处访问共享资源 } // 解除 m 的锁定
Condition variable
Condition variable allows a thread to wait for a specific condition, such as when a shared resource is available. Using std::condition_variable
:
std::condition_variable cv; std::unique_lock<std::mutex> lock(m); cv.wait(lock); // 等待 cv 信号 cv.notify_one(); // 唤醒一个等待线程
Practical case: multi-threaded file copy
The following code demonstrates how to use STL to implement multi-threaded file copy:
#include <fstream> #include <iostream> #include <thread> #include <vector> void copy_file(const std::string& src, const std::string& dst) { std::ifstream infile(src); std::ofstream outfile(dst); outfile << infile.rdbuf(); } int main() { std::vector<std::thread> threads; const int num_threads = 4; // 创建线程池 for (int i = 0; i < num_threads; ++i) { threads.emplace_back(copy_file, "input.txt", "output" + std::to_string(i) + ".txt"); } // 等待所有线程完成 for (auto& t : threads) { t.join(); } std::cout << "Files copied successfully!" << std::endl; return 0; }
The above is the detailed content of How to implement multi-threaded programming in C++ using STL?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
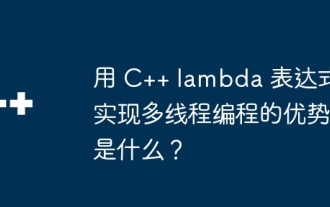
The advantages of lambda expressions in C++ multi-threaded programming include simplicity, flexibility, ease of parameter passing, and parallelism. Practical case: Use lambda expressions to create multi-threads and print thread IDs in different threads, demonstrating the simplicity and ease of use of this method.
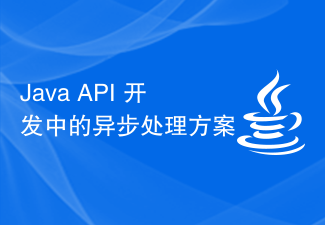
With the continuous development of Java technology, Java API has become one of the mainstream solutions developed by many enterprises. During the development process of Java API, a large number of requests and data often need to be processed, but the traditional synchronous processing method cannot meet the needs of high concurrency and high throughput. Therefore, asynchronous processing has become one of the important solutions in JavaAPI development. This article will introduce asynchronous processing solutions commonly used in Java API development and how to use them. 1. Java differences
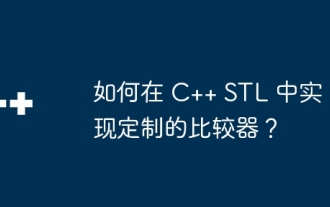
Implementing a custom comparator can be accomplished by creating a class that overloads operator(), which accepts two parameters and indicates the result of the comparison. For example, the StringLengthComparator class sorts strings by comparing their lengths: Create a class and overload operator(), returning a Boolean value indicating the comparison result. Using custom comparators for sorting in container algorithms. Custom comparators allow us to sort or compare data based on custom criteria, even if we need to use custom comparison criteria.
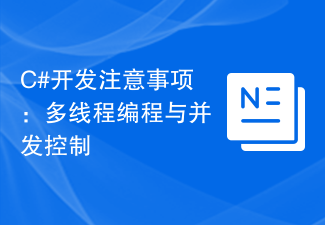
In C# development, multi-threaded programming and concurrency control are particularly important in the face of growing data and tasks. This article will introduce some matters that need to be paid attention to in C# development from two aspects: multi-threaded programming and concurrency control. 1. Multi-threaded programming Multi-threaded programming is a technology that uses multi-core resources of the CPU to improve program efficiency. In C# programs, multi-thread programming can be implemented using Thread class, ThreadPool class, Task class and Async/Await. But when doing multi-threaded programming
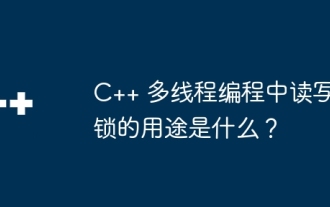
In multi-threading, read-write locks allow multiple threads to read data at the same time, but only allow one thread to write data to improve concurrency and data consistency. The std::shared_mutex class in C++ provides the following member functions: lock(): Gets write access and succeeds when no other thread holds the read or write lock. lock_read(): Obtain read access permission, which can be held simultaneously with other read locks or write locks. unlock(): Release write access permission. unlock_shared(): Release read access permission.

Using STL function objects can improve reusability and includes the following steps: Define the function object interface (create a class and inherit from std::unary_function or std::binary_function) Overload operator() to define the function behavior in the overloaded operator() Implement the required functionality using function objects via STL algorithms (such as std::transform)
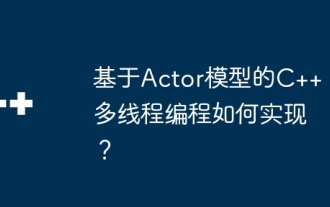
C++ multi-threaded programming implementation based on the Actor model: Create an Actor class that represents an independent entity. Set the message queue where messages are stored. Defines the method for an Actor to receive and process messages from the queue. Create Actor objects and start threads to run them. Send messages to Actors via the message queue. This approach provides high concurrency, scalability, and isolation, making it ideal for applications that need to handle large numbers of parallel tasks.
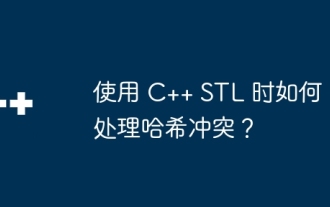
The methods for handling C++STL hash conflicts are: chain address method: using linked lists to store conflicting elements, which has good applicability. Open addressing method: Find available locations in the bucket to store elements. The sub-methods are: Linear detection: Find the next available location in sequence. Quadratic Detection: Search by skipping positions in quadratic form.
