How to handle exceptions in Goroutine?
Handling exceptions in Goroutine: Use recover to get the exception from the recovery point. Handle exceptions within defer statements, such as a print-friendly errorMessage. Practical case: Asynchronously check user access permissions and capture exceptions with insufficient permissions, and display friendly messages to users.
#How to handle exceptions in Goroutine?
In concurrent programming, coroutines or Goroutines are lightweight threads that execute independently. However, handling exceptions in Goroutines is not quite the same as with traditional threads.
Handling exceptions in Goroutine
First, let us create a Goroutine:
func main() { go func() { // 可能会抛出异常的代码 }() }
Go does not catch exceptions in Goroutine by default. If the Goroutine throws an exception, the program will crash. In order to handle the exception, we need to use the recover
function:
func main() { go func() { defer func() { if r := recover(); r != nil { // 处理异常 fmt.Println("捕获到异常:", r) } }() }() }
Inside the defer
statement, we use recover
to get the exception from the recovery point and Process it as needed.
Practical Case: Accessing Protected Resources
Suppose we have a protected resource that only users with specific access permissions can access it. We can use Goroutine to check the user's permissions asynchronously:
func checkAccess(userId int) error { user, err := getUserByID(userId) if err != nil { return err } if user.accessLevel != ADMIN { return errors.New("没有访问权限") } return nil } func main() { userIDs := []int{1, 2, 3} for _, id := range userIDs { go func(userId int) { if err := checkAccess(userId); err != nil { defer func() { if r := recover(); r != nil { // 处理异常:权限不足 fmt.Println("用户", userId, ": 权限不足") } }() panic(err) } fmt.Println("用户", userId, ": 有访问权限") }(id) } }
In this example, Goroutine may throw an errors.New("No access permission")
exception, which will cause the program to collapse. By using the defer
statement and the recover
function, we are able to catch the exception and display a friendly errorMessage to the user.
The above is the detailed content of How to handle exceptions in Goroutine?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


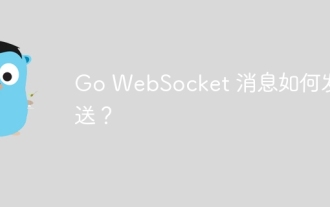
In Go, WebSocket messages can be sent using the gorilla/websocket package. Specific steps: Establish a WebSocket connection. Send a text message: Call WriteMessage(websocket.TextMessage,[]byte("Message")). Send a binary message: call WriteMessage(websocket.BinaryMessage,[]byte{1,2,3}).
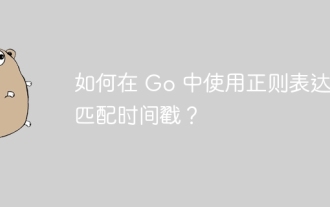
In Go, you can use regular expressions to match timestamps: compile a regular expression string, such as the one used to match ISO8601 timestamps: ^\d{4}-\d{2}-\d{2}T \d{2}:\d{2}:\d{2}(\.\d+)?(Z|[+-][0-9]{2}:[0-9]{2})$ . Use the regexp.MatchString function to check if a string matches a regular expression.
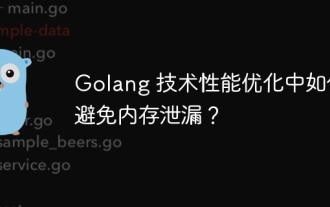
Memory leaks can cause Go program memory to continuously increase by: closing resources that are no longer in use, such as files, network connections, and database connections. Use weak references to prevent memory leaks and target objects for garbage collection when they are no longer strongly referenced. Using go coroutine, the coroutine stack memory will be automatically released when exiting to avoid memory leaks.
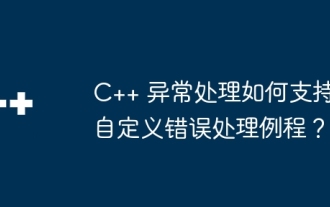
C++ exception handling allows the creation of custom error handling routines to handle runtime errors by throwing exceptions and catching them using try-catch blocks. 1. Create a custom exception class derived from the exception class and override the what() method; 2. Use the throw keyword to throw an exception; 3. Use the try-catch block to catch exceptions and specify the exception types that can be handled.
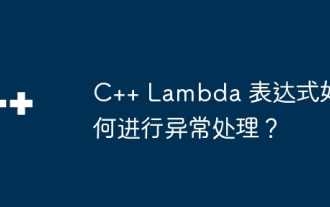
Exception handling in C++ Lambda expressions does not have its own scope, and exceptions are not caught by default. To catch exceptions, you can use Lambda expression catching syntax, which allows a Lambda expression to capture a variable within its definition scope, allowing exception handling in a try-catch block.
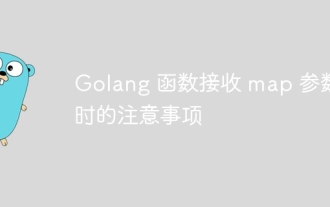
When passing a map to a function in Go, a copy will be created by default, and modifications to the copy will not affect the original map. If you need to modify the original map, you can pass it through a pointer. Empty maps need to be handled with care, because they are technically nil pointers, and passing an empty map to a function that expects a non-empty map will cause an error.
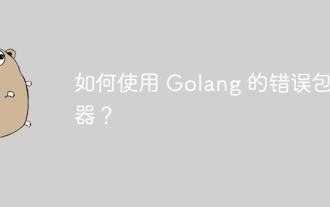
In Golang, error wrappers allow you to create new errors by appending contextual information to the original error. This can be used to unify the types of errors thrown by different libraries or components, simplifying debugging and error handling. The steps are as follows: Use the errors.Wrap function to wrap the original errors into new errors. The new error contains contextual information from the original error. Use fmt.Printf to output wrapped errors, providing more context and actionability. When handling different types of errors, use the errors.Wrap function to unify the error types.
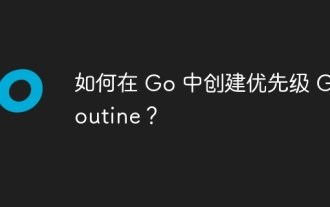
There are two steps to creating a priority Goroutine in the Go language: registering a custom Goroutine creation function (step 1) and specifying a priority value (step 2). In this way, you can create Goroutines with different priorities, optimize resource allocation and improve execution efficiency.
