How to generate random numbers in Golang lambda function?
To generate random numbers in a Go lambda function, you need to use the math/rand library: import the library and set the seed to ensure different outputs. Use rand.Intn(max) to generate a random integer (range [0,max)). Use rand.Float64() to generate a random decimal (range [0.0,1.0)). Use rand.ReadStringN(n) to generate a random string (of length n).
#How to generate random numbers in Golang lambda function?
The Golang language has a built-in powerful random number generation library math/rand
. Using this library we can easily generate random numbers in lambda functions.
Installation and setup
First, import the math/rand
library:
import ( "math/rand" "time" )
time.Now() The .UnixNano()
part is used to generate the seed, ensuring a different output each time a random number is generated.
Generate a random integer
You can use the rand.Intn(max)
function to generate a random integer between [0, max)
A random integer in the range, where max
specifies the upper limit.
max := 10 num := rand.Intn(max) fmt.Println(num) // 输出一个介于 [0, 10) 范围内的随机整数
Generate a random decimal
You can use the rand.Float64()
function to generate a random decimal number between [0.0, 1.0)
Random decimal number within the range.
num := rand.Float64() fmt.Println(num) // 输出一个介于 [0.0, 1.0) 范围内的随机小数
Generate a random string
You can use the rand.ReadStringN(n)
function to generate a length of n
Random string.
length := 10 str := make([]byte, length) rand.ReadStringN(len(str), str) fmt.Println(string(str)) // 输出一个 10 个字符长的随机字符串
Practical case
The following is a simple example of using the math/rand
library to generate random numbers in a Golang lambda function:
package main import ( "context" "encoding/json" "fmt" "log" "math/rand" "time" "github.com/aws/aws-lambda-go/lambda" ) func handler(ctx context.Context, req []byte) (int, error) { rand.Seed(time.Now().UnixNano()) return rand.Intn(10), nil } func main() { lambda.Start(handler) }
This lambda function will generate a random integer in the range [0, 10)
and output it to the function log.
The above is the detailed content of How to generate random numbers in Golang lambda function?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
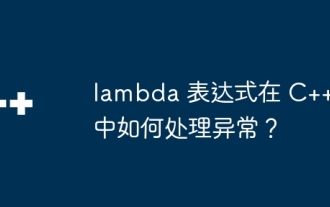
In C++, there are two ways to handle exceptions using Lambda expressions: catch the exception using a try-catch block, and handle or rethrow the exception in the catch block. Using a wrapper function of type std::function, its try_emplace method can catch exceptions in Lambda expressions.
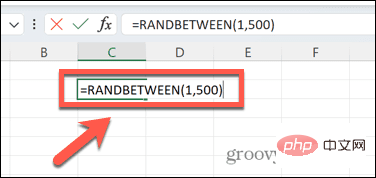
How to use RANDBETWEEN to generate random numbers in Excel If you want to generate random numbers within a specific range, the RANDBETWEEN function is a quick and easy way to do it. This allows you to generate random integers between any two values of your choice. Generate random numbers in Excel using RANDBETWEEN: Click the cell where you want the first random number to appear. Type =RANDBETWEEN(1,500) replacing "1" with the lowest random number you want to generate and "500" with
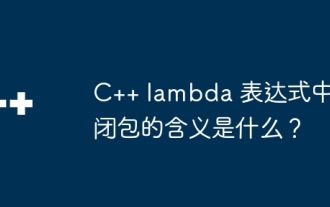
In C++, a closure is a lambda expression that can access external variables. To create a closure, capture the outer variable in the lambda expression. Closures provide advantages such as reusability, information hiding, and delayed evaluation. They are useful in real-world situations such as event handlers, where the closure can still access the outer variables even if they are destroyed.
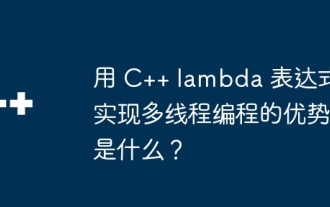
The advantages of lambda expressions in C++ multi-threaded programming include simplicity, flexibility, ease of parameter passing, and parallelism. Practical case: Use lambda expressions to create multi-threads and print thread IDs in different threads, demonstrating the simplicity and ease of use of this method.
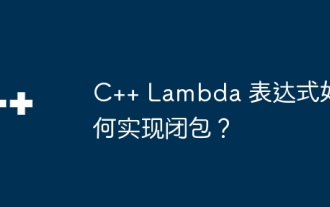
C++ Lambda expressions support closures, which save function scope variables and make them accessible to functions. The syntax is [capture-list](parameters)->return-type{function-body}. capture-list defines the variables to capture. You can use [=] to capture all local variables by value, [&] to capture all local variables by reference, or [variable1, variable2,...] to capture specific variables. Lambda expressions can only access captured variables but cannot modify the original value.
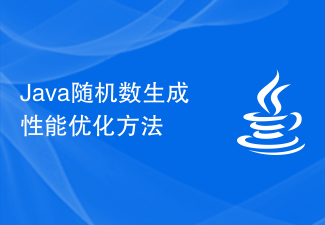
How to optimize random number generation performance in Java development Random numbers are widely used in computer science, especially in cryptography, simulation, games and other fields. In Java development, we often need to generate random numbers to meet various needs. However, the performance of random number generation is often one of the concerns of developers. This article will explore how to optimize random number generation performance in Java development. ThreadLocalRandom class was introduced in Java7 using ThreadLocalRandom class
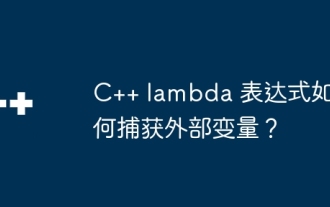
There are three ways to capture lambda expressions of external variables in C++: Capture by value: Create a copy of the variable. Capture by reference: Get a variable reference. Capture by value and reference simultaneously: Allows capturing of multiple variables, either by value or by reference.
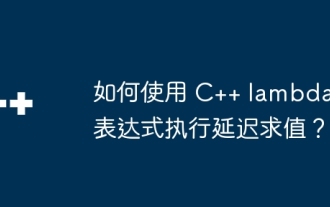
How to perform lazy evaluation using C++ lambda expressions? Use lambda expressions to create lazily evaluated function objects. Delayed computation defers execution until needed. Calculate results only when needed, improving performance.
