


How to build a RESTful API and implement health checks using Golang?
Jun 05, 2024 pm 12:33 PMUse Golang to build a RESTful API and implement health checks: Build a RESTful API: Create a new project, define the data model, define routes, and implement handlers. Implement health checks: Define health check endpoints and implement health check handlers. This example shows how to build an API that returns a list of users and implements the health check endpoint: Get the list of users via GET /users. Check API health via GET /healthz.
How to use Golang to build RESTful API and implement health check
Introduction
As a modern application communication standard, RESTful API is increasingly favored by developers. Golang is ideal for building RESTful APIs due to its high performance and concurrency. At the same time, implementing health checks is critical to ensuring the API is functioning properly. This article will guide you to build a RESTful API and implement health checks using Golang.
Build RESTful API
1. Create a new project
go mod init rest-api
2. Define the data model
type User struct { ID int `json:"id"` Username string `json:"username"` Password string `json:"password"` }
3. Define routing
func main() { router := mux.NewRouter() router.HandleFunc("/users", getUsers).Methods("GET") // ... 更多路由 log.Fatal(http.ListenAndServe(":8080", router)) }
4. Implement handler
func getUsers(w http.ResponseWriter, r *http.Request) { users := []User{ {ID: 1, Username: "user1", Password: "password1"}, // ... 更多用户 } json.NewEncoder(w).Encode(users) }
Implement health check
1. Define the health check endpoint
router.HandleFunc("/healthz", healthz).Methods("GET")
2. Implement the health check handler
func healthz(w http.ResponseWriter, r *http.Request) { // TODO: 检查数据库连接性、缓存可用性等指标 if healthy { w.WriteHeader(http.StatusOK) w.Write([]byte("OK")) } else { w.WriteHeader(http.StatusServiceUnavailable) w.Write([]byte("ERROR")) } }
Practical combat Case
This example shows how to use Golang to build a RESTful API (returning a user list) and implement a health check endpoint:
- via HTTP GET request
/users
Get user list - Via HTTP GET request
/healthz
Check API health
Full code:
package main import ( "encoding/json" "net/http" "github.com/gorilla/mux" ) type User struct { ID int `json:"id"` Username string `json:"username"` Password string `json:"password"` } func getUsers(w http.ResponseWriter, r *http.Request) { users := []User{ {ID: 1, Username: "user1", Password: "password1"}, {ID: 2, Username: "user2", Password: "password2"}, } json.NewEncoder(w).Encode(users) } func healthz(w http.ResponseWriter, r *http.Request) { // TODO: 检查数据库连接性、缓存可用性等指标 if healthy { w.WriteHeader(http.StatusOK) w.Write([]byte("OK")) } else { w.WriteHeader(http.StatusServiceUnavailable) w.Write([]byte("ERROR")) } } func main() { router := mux.NewRouter() router.HandleFunc("/users", getUsers).Methods("GET") router.HandleFunc("/healthz", healthz).Methods("GET") http.ListenAndServe(":8080", router) }
The above is the detailed content of How to build a RESTful API and implement health checks using Golang?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
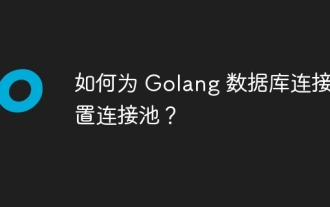
How to configure connection pool for Golang database connection?
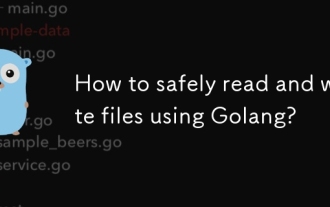
How to safely read and write files using Golang?
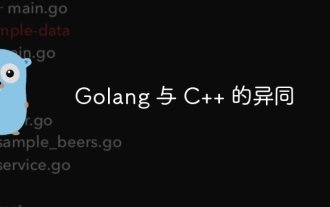
Similarities and Differences between Golang and C++
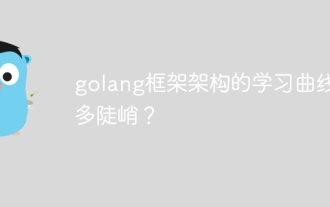
How steep is the learning curve of golang framework architecture?
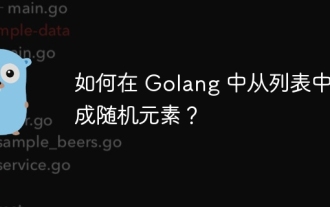
How to generate random elements from list in Golang?
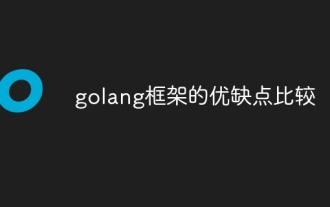
Comparison of advantages and disadvantages of golang framework

What are the best practices for error handling in Golang framework?
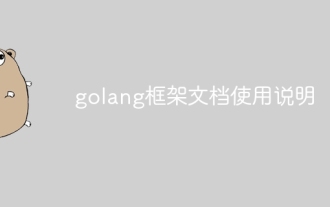
golang framework document usage instructions
