


Challenges and countermeasures of C++ memory management in multi-threaded environment?
In a multi-threaded environment, C++ memory management faces the following challenges: data races, deadlocks and memory leaks. Countermeasures include: 1. Using synchronization mechanisms, such as mutex locks and atomic variables; 2. Using lock-free data structures; 3. Using smart pointers; 4. (Optional) Implementing garbage collection.
Challenges and countermeasures of C++ memory management in a multi-threaded environment
In a multi-threaded environment, C++ memory management becomes is particularly complex. Concurrent access to a shared memory region by multiple threads can lead to data corruption, deadlocks, and undefined behavior.
Challenge
- Data race: When multiple threads access the same memory location at the same time and try to write to it When, a data race occurs. This can lead to undefined behavior and data corruption.
- Deadlock: A deadlock occurs when two or more threads wait for each other. Each thread holds resources that the other needs, preventing any progress.
- Memory leak (memory leak): A memory leak occurs when a thread no longer uses a piece of memory, but the memory is not released correctly. This consumes memory and causes performance degradation.
Countermeasures
-
Synchronization: Use synchronization mechanisms such as mutexes, mutexes or atomic variables. They ensure that only one thread can access a shared resource at a time. For example,
std::mutex
andstd::atomic
are standard library types used for synchronization in C++. - Lock-free data structures: Use lock-free data structures that do not rely on locks, such as concurrent queues and hash tables. These structures allow threads to access data concurrently, avoiding data races.
-
Smart pointers: Use smart pointers in C++ for memory management. Smart pointers automatically manage the lifetime of objects and help prevent memory leaks. For example,
std::shared_ptr
andstd::unique_ptr
are commonly used smart pointers. - Garbage collection (optional): There is no built-in garbage collection mechanism in C++. However, third-party libraries, such as Boost.SmartPointers, can be used to implement garbage collection.
Practical case
Consider a multi-threaded application that shares a thread-safe queue to deliver messages. The queue is synchronized using a mutex:
class ThreadSafeQueue { public: void push(const std::string& msg) { std::lock_guard<std::mutex> lock(mtx); queue.push(msg); } bool pop(std::string& msg) { std::lock_guard<std::mutex> lock(mtx); if (queue.empty()) { return false; } msg = queue.front(); queue.pop(); return true; } private: std::queue<std::string> queue; std::mutex mtx; };
Conclusion
C++ memory management in a multi-threaded environment is a complex challenge. By understanding the challenges and applying appropriate countermeasures, shared memory can be managed safely and efficiently.
The above is the detailed content of Challenges and countermeasures of C++ memory management in multi-threaded environment?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics

C++ object layout and memory alignment optimize memory usage efficiency: Object layout: data members are stored in the order of declaration, optimizing space utilization. Memory alignment: Data is aligned in memory to improve access speed. The alignas keyword specifies custom alignment, such as a 64-byte aligned CacheLine structure, to improve cache line access efficiency.
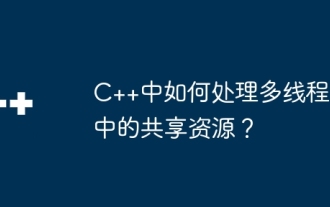
Mutexes are used in C++ to handle multi-threaded shared resources: create mutexes through std::mutex. Use mtx.lock() to obtain a mutex and provide exclusive access to shared resources. Use mtx.unlock() to release the mutex.
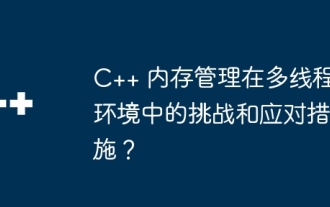
In a multi-threaded environment, C++ memory management faces the following challenges: data races, deadlocks, and memory leaks. Countermeasures include: 1. Use synchronization mechanisms, such as mutexes and atomic variables; 2. Use lock-free data structures; 3. Use smart pointers; 4. (Optional) implement garbage collection.

The reference counting mechanism is used in C++ memory management to track object references and automatically release unused memory. This technology maintains a reference counter for each object, and the counter increases and decreases when references are added or removed. When the counter drops to 0, the object is released without manual management. However, circular references can cause memory leaks, and maintaining reference counters increases overhead.
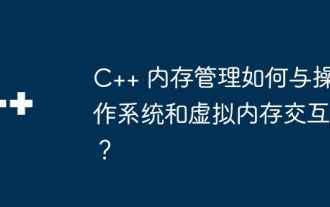
C++ memory management interacts with the operating system, manages physical memory and virtual memory through the operating system, and efficiently allocates and releases memory for programs. The operating system divides physical memory into pages and pulls in the pages requested by the application from virtual memory as needed. C++ uses the new and delete operators to allocate and release memory, requesting memory pages from the operating system and returning them respectively. When the operating system frees physical memory, it swaps less used memory pages into virtual memory.
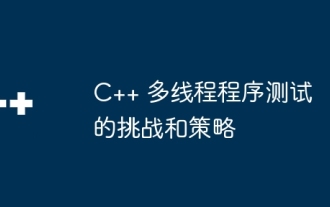
Multi-threaded program testing faces challenges such as non-repeatability, concurrency errors, deadlocks, and lack of visibility. Strategies include: Unit testing: Write unit tests for each thread to verify thread behavior. Multi-threaded simulation: Use a simulation framework to test your program with control over thread scheduling. Data race detection: Use tools to find potential data races, such as valgrind. Debugging: Use a debugger (such as gdb) to examine the runtime program status and find the source of the data race.
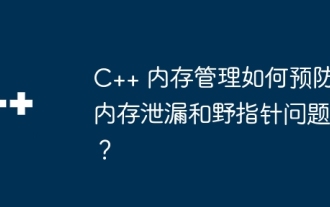
When it comes to memory management in C++, there are two common errors: memory leaks and wild pointers. Methods to solve these problems include: using smart pointers (such as std::unique_ptr and std::shared_ptr) to automatically release memory that is no longer used; following the RAII principle to ensure that resources are released when the object goes out of scope; initializing the pointer and accessing only Valid memory, with array bounds checking; always use the delete keyword to release dynamically allocated memory that is no longer needed.

In multithreaded C++, exception handling follows the following principles: timeliness, thread safety, and clarity. In practice, you can ensure thread safety of exception handling code by using mutex or atomic variables. Additionally, consider reentrancy, performance, and testing of your exception handling code to ensure it runs safely and efficiently in a multi-threaded environment.
