


Example of using golang framework extension to solve business pain points
Abstract: Using framework extensions in Golang can solve various business pain points. For example: Use gRPC to improve microservice communication efficiency: Import the necessary libraries to connect to the gRPC server. Create a gRPC client. Send an RPC request and print a response. Other useful Golang framework extensions: Echo (Web framework) Gin (High-performance Web framework) GORM (ORM) Celery (distributed task queue) Ent (feature-rich ORM)
Use framework extensions to solve business pain points in Golang
In software development, a framework is a library of pre-built components that help developers quickly build powerful, scalable applications. In the Golang ecosystem, there are many excellent frameworks that can be used to solve common business pain points.
Practical Case: Using gRPC to Improve Microservice Communication Efficiency
Microservice architecture has become a popular choice for modern application development. However, managing communication between microservices can be complex and time-consuming. gRPC is a Golang framework that provides an efficient and flexible RPC (Remote Procedure Call) mechanism suitable for microservice communication.
Here are the steps to extend a Golang application using gRPC to improve the efficiency of microservice communication:
import ( "context" "log" pb "example.com/mypackage/proto" "google.golang.org/grpc" ) func main() { // 连接到 gRPC 服务端 conn, err := grpc.Dial("localhost:8080", grpc.WithInsecure()) if err != nil { log.Fatalf("Failed to connect: %v", err) } // 创建 gRPC 客户端 client := pb.NewUserServiceClient(conn) // 发送 RPC 请求 resp, err := client.GetUser(context.Background(), &pb.GetUserRequest{Id: 1}) if err != nil { log.Fatalf("Failed to get user: %v", err) } // 打印响应 log.Printf("User: %#v", resp) }
Examples of other Golang framework extensions
In addition to gRPC , there are many other useful frameworks in Golang that can help you solve various business pain points:
- Echo: A simple and efficient web framework for building APIs and RESTful services .
- Gin: Another high-performance web framework known for its high scalability and modularity.
- GORM: A complete ORM (Object Relational Mapper) for interacting with the database and simplifying data model management.
- Celery: A distributed task queue for asynchronously executing time-consuming tasks to improve application performance and scalability.
- Ent: A feature-rich ORM designed to solve complex database modeling and querying problems.
By using the appropriate Golang framework, you can quickly and efficiently scale your applications, solve business pain points and improve development efficiency.
The above is the detailed content of Example of using golang framework extension to solve business pain points. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




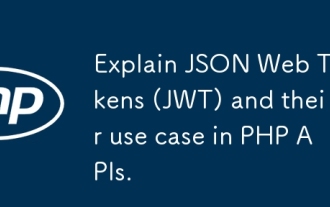
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
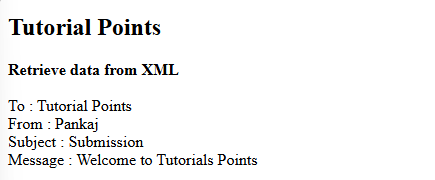
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
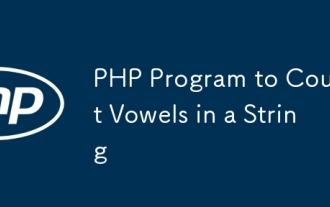
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
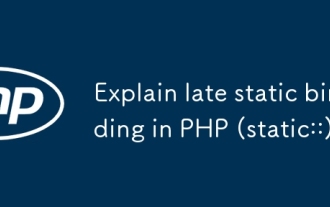
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
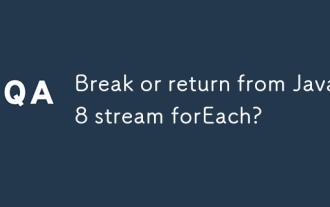
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
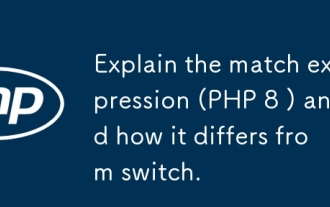
In PHP8, match expressions are a new control structure that returns different results based on the value of the expression. 1) It is similar to a switch statement, but returns a value instead of an execution statement block. 2) The match expression is strictly compared (===), which improves security. 3) It avoids possible break omissions in switch statements and enhances the simplicity and readability of the code.

In PHP, you can effectively prevent CSRF attacks by using unpredictable tokens. Specific methods include: 1. Generate and embed CSRF tokens in the form; 2. Verify the validity of the token when processing the request.
