How to use HeapTrack to debug C++ memory management?
HeapTrack is a Microsoft Visual C++ tool for debugging C++ memory management issues, including: Enable HeapTrack: Enable "HeapCheck" in the Debug settings of the project properties. Create a HeapTrack instance: Use the HeapCreate() function in code. Practical example: HeapTrack helps identify memory leaks by detecting memory block usage.
Debug C++ memory management using HeapTrack
HeapTrack is a powerful tool in Microsoft Visual C++ that can be used to detect and fix memory management problems.
Enable HeapTrack
Before enabling HeapTrack, some changes need to be made to the project.
- Open the project properties page: Right-click the project and select "Properties".
- Configure the "Debug" setting: Under "Configuration Properties" > "Debugging", find the "HeapCheck" setting and set it to "Detailed".
Create a HeapTrack instance
In the code, you need to create a HeapTrack instance. This will initialize HeapTrack and start monitoring memory allocations.
#include <windows.h> int main() { // 创建 HeapTrack 实例 HANDLE heapTrack = HeapCreate(0, 0, 0); if (heapTrack == NULL) { return ERROR_INVALID_HANDLE; } // ... 您的代码 ... // 销毁 HeapTrack 实例 if (!HeapDestroy(heapTrack)) { return ERROR_INVALID_HANDLE; } return 0; }
Practical Case
Now, let us look at a practical case demonstrating how to use HeapTrack to detect memory leaks.
Code Example:
#include <windows.h> int main() { // 创建 HeapTrack 实例 HANDLE heapTrack = HeapCreate(0, 0, 0); if (heapTrack == NULL) { return ERROR_INVALID_HANDLE; } // 分配内存并泄漏 int* ptr = new int; // ... 您的代码 ... // 检测内存泄漏 HEAP_SUMMARY summary; if (!HeapSummary(heapTrack, &summary)) { return ERROR_INVALID_HANDLE; } // 检查内存泄漏 if (summary.BlocksInUse != 0) { // 内存泄漏已检测到 return ERROR_MEMORY_LEAK; } // 销毁 HeapTrack 实例 if (!HeapDestroy(heapTrack)) { return ERROR_INVALID_HANDLE; } return 0; }
In the above example, the ptr
pointer is allocated memory and leaked because is not used The delete
operator releases memory. When the HeapTrack is destroyed, it will detect the unreleased memory and report a memory leak.
The above is the detailed content of How to use HeapTrack to debug C++ memory management?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


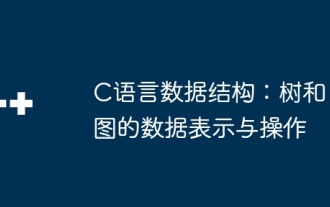
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
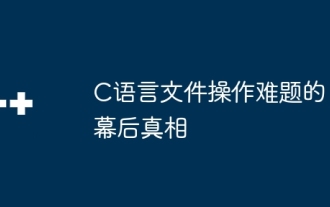
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
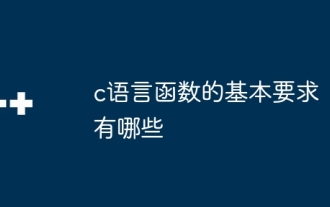
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
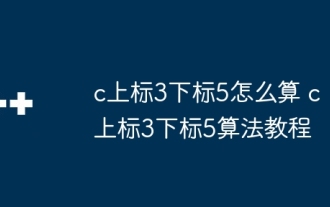
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
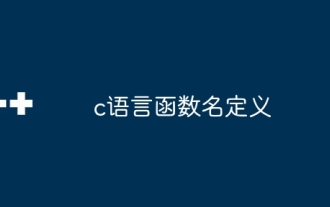
The C language function name definition includes: return value type, function name, parameter list and function body. Function names should be clear, concise and unified in style to avoid conflicts with keywords. Function names have scopes and can be used after declaration. Function pointers allow functions to be passed or assigned as arguments. Common errors include naming conflicts, mismatch of parameter types, and undeclared functions. Performance optimization focuses on function design and implementation, while clear and easy-to-read code is crucial.
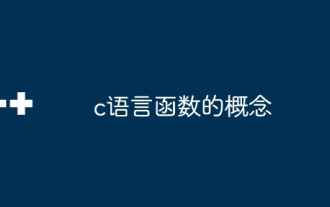
C language functions are reusable code blocks. They receive input, perform operations, and return results, which modularly improves reusability and reduces complexity. The internal mechanism of the function includes parameter passing, function execution, and return values. The entire process involves optimization such as function inline. A good function is written following the principle of single responsibility, small number of parameters, naming specifications, and error handling. Pointers combined with functions can achieve more powerful functions, such as modifying external variable values. Function pointers pass functions as parameters or store addresses, and are used to implement dynamic calls to functions. Understanding function features and techniques is the key to writing efficient, maintainable, and easy to understand C programs.
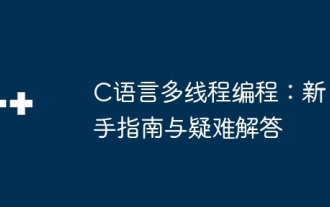
C language multithreading programming guide: Creating threads: Use the pthread_create() function to specify thread ID, properties, and thread functions. Thread synchronization: Prevent data competition through mutexes, semaphores, and conditional variables. Practical case: Use multi-threading to calculate the Fibonacci number, assign tasks to multiple threads and synchronize the results. Troubleshooting: Solve problems such as program crashes, thread stop responses, and performance bottlenecks.
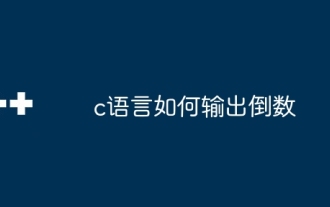
How to output a countdown in C? Answer: Use loop statements. Steps: 1. Define the variable n and store the countdown number to output; 2. Use the while loop to continuously print n until n is less than 1; 3. In the loop body, print out the value of n; 4. At the end of the loop, subtract n by 1 to output the next smaller reciprocal.
