


How to implement function template reuse in generic programming in C++?
Jun 05, 2024 pm 02:57 PMGeneric programming in C++ is implemented through function templates, making the code independent of data types and reusable. Function templates are general-purpose functions whose arguments are specified as type names and can handle any type of data. By using function template reuse, you can achieve code reusability, reduce redundancy, improve scalability, and create efficient and flexible C++ code.
Generic programming in C++: Implementing function template reuse
Generic programming is a technique for writing code. Allowing it to work on multiple data types independently of the concrete type. In C++, generic programming can be implemented through function templates.
Function template
A function template is a general function that can handle any type of data. To create a function template, use the following syntax:
template<typename T> T add(T a, T b) { return a + b; }
typename T
Specifies that the argument to the template is a type name.
Practical Case
Suppose we have a function that adds two numbers. Using generic programming, we can write a general function that can handle any type of number:
#include <iostream> template<typename T> T add(T a, T b) { return a + b; } int main() { int x = 5; int y = 3; std::cout << add(x, y) << '\n'; // 输出 8 double d1 = 3.14; double d2 = 2.71; std::cout << add(d1, d2) << '\n'; // 输出 5.85 }
In this example, the add() function accepts two types of template parameters T and can be used to combine the two types. Add numbers of different types.
Advantages
Function template reuse provides many advantages, including:
- Code reusability: You can reuse common functions on multiple data types.
- Reduce code redundancy: You don’t need to write a separate function for each data type.
- Extensibility: When adding new data types, you don’t have to modify existing code.
By using function templates, you can create efficient, flexible, and reusable C++ code.
The above is the detailed content of How to implement function template reuse in generic programming in C++?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics

Generic programming in PHP and its applications
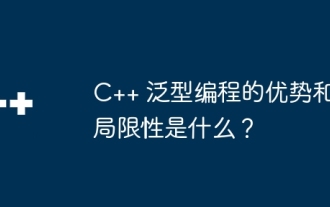
What are the advantages and limitations of C++ generic programming?
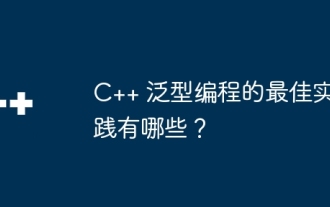
What are the best practices for generic programming in C++?
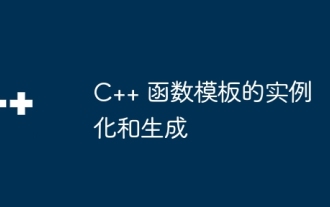
Instantiation and generation of C++ function templates
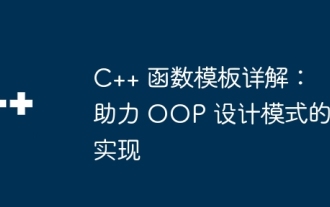
Detailed explanation of C++ function templates: assisting the implementation of OOP design patterns
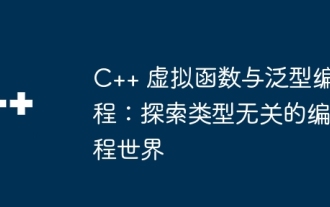
C++ Virtual Functions and Generic Programming: Exploring the World of Type-Independent Programming
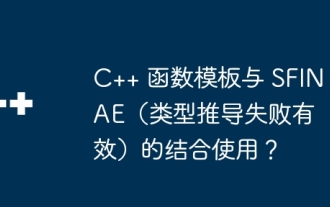
C++ function templates combined with SFINAE (type derivation fails valid)?
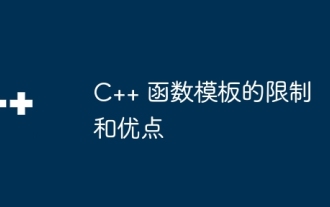
Limitations and advantages of C++ function templates
