PHP microservice architecture practice
PHP Microservice Architecture Practice: Installing the LEMP stack: Install Linux, Nginx, MySQL and PHP. Create a MySQL database: Create a database to store data. Install Composer: Use Composer to manage PHP dependencies. Build the microservice: Use Symfony to create a new Composer project and configure the service. Create entities: Define entities for mapping to database tables. Create a database schema: Use Doctrine to create a database schema. Create an API controller: A controller that handles user requests. Running microservices: Start microservices using PHP built-in server.
PHP microservice architecture practice
Introduction
Microservice is a software architecture style , decompose applications into independent and scalable services. PHP is a popular backend language that is ideal for building microservices. This article will guide you through a practical case to complete the construction of PHP microservice architecture.
Install the LEMP stack
First, you need to install the LEMP (Linux, Nginx, MySQL, PHP) stack:
# Ubuntu/Debian sudo apt update && sudo apt install nginx mysql-server php8.1 # CentOS/Fedora sudo yum update && sudo yum install epel-release sudo yum install nginx mariadb php81
Create the MySQL database
Next, create a MySQL database for storing data:
CREATE DATABASE micro_services; GRANT ALL PRIVILEGES ON micro_services.* TO 'user'@'localhost' IDENTIFIED BY 'password';
Install Composer
Composer is a PHP dependency management tool:
sudo curl -sS https://getcomposer.org/installer | php sudo mv composer.phar /usr/local/bin/composer
Building Microservices
Next, create a new Composer project:
composer create-project symfony/skeleton micro_services cd micro_services
Add the following content to the config/services.yaml
file:
services: database.connection: # 数据库连接 class: Doctrine\DBAL\Connection arguments: dsn: '%env(DATABASE_URL)%' monolog.logger: # 日志记录器 class: Monolog\Logger arguments: [micro_services] calls: - [pushHandler, [new Monolog\Handler\StreamHandler('logs/dev.log')]]
Create src/Entity/User.php
entity that maps to the user table in the database:
namespace App\Entity; use Doctrine\ORM\Mapping as ORM; /** * @ORM\Entity * @ORM\Table(name="users") */ class User { /** * @ORM\Id * @ORM\GeneratedValue(strategy="AUTO") * @ORM\Column(type="integer") */ private $id; /** * @ORM\Column(type="string", length=255) */ private $email; // ... }
Run the following command to create the database schema:
composer dump-autoload && php bin/console doctrine:database:create
Create API Controller
Create an API controller to handle user requests:
namespace App\Controller; use Symfony\Bundle\FrameworkBundle\Controller\AbstractController; use Symfony\Component\HttpFoundation\Response; use Symfony\Component\Routing\Annotation\Route; use Doctrine\ORM\EntityManagerInterface; class UserController extends AbstractController { /** * @Route("/api/users", methods={"GET"}) */ public function index(EntityManagerInterface $em): Response { $users = $em->getRepository(User::class)->findAll(); return $this->json($users); } }
Run the microservice
Finally, start the PHP built-in server to Run the microservice:
php -S localhost:8000 public/index.php
Visit http://localhost:8000/api/users
to get the user list.
The above is the detailed content of PHP microservice architecture practice. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


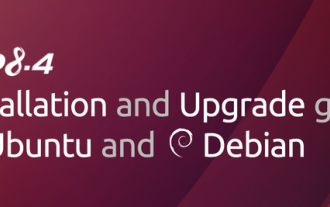
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
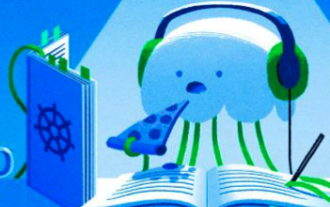
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
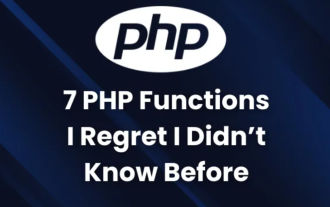
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
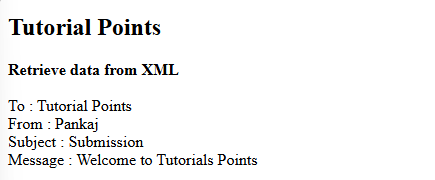
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
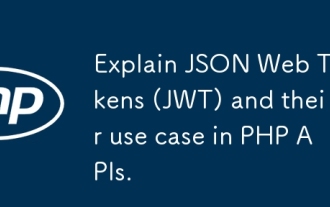
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
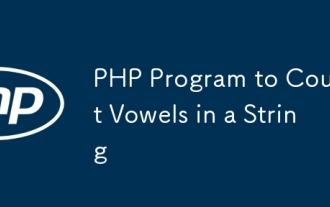
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
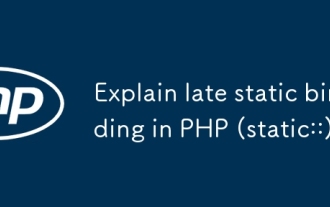
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
