How to use atomic operations in C++ to ensure thread safety?
Using atomic operations in C++ can ensure thread safety, using std::atomic
Use atomic operations in C++ to ensure thread safety
In a multi-threaded environment, when multiple threads concurrently access shared data, it may cause Data race issues, resulting in unpredictable results. In order to prevent this situation, you can use the atomic operation mechanism in C++ to ensure thread safety.
Introduction to Atomic Operations
Atomic operations are special instructions used to operate on data in memory to ensure that the operation is performed in an atomic manner, that is, it is either executed in full or not at all. This means that when one thread performs an atomic operation, other threads cannot access the same data at the same time.
Atomic operations in C++
C++11 introduces the <atomic></atomic>
header file, which provides various atomic operations, including:
-
std::atomic<t></t>
: Template class, representing atomic operations of atomic types. -
std::atomic_flag
: No-argument atomic flag, indicating Boolean type atomic operation. -
std::atomic_init(), std::atomic_load(), std::atomic_store()
and other functions: basic functions of atomic operations.
Practical case: Thread-safe counter
The following is an example of using atomic operations to implement a thread-safe counter:
#include <atomic> #include <iostream> #include <thread> std::atomic<int> counter{0}; void increment_counter() { for (int i = 0; i < 1000000; ++i) { // 使用原子操作递增计数器 ++counter; } } int main() { // 创建多个线程并发递增计数器 std::thread threads[4]; for (int i = 0; i < 4; ++i) { threads[i] = std::thread(increment_counter); } // 等待所有线程完成 for (int i = 0; i < 4; ++i) { threads[i].join(); } // 打印最终计数器值 std::cout << "Final counter value: " << counter << std::endl; return 0; }
In this example, we use std::atomic<int></int>
Create an atomic integer counter and increment the counter concurrently in multiple threads. Due to the use of atomic operations, even if multiple threads access the counter at the same time, thread safety will be guaranteed and the correct counter value will eventually be output.
The above is the detailed content of How to use atomic operations in C++ to ensure thread safety?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
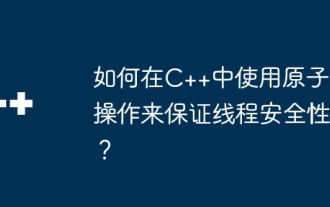
Thread safety can be guaranteed by using atomic operations in C++, using std::atomic template class and std::atomic_flag class to represent atomic types and Boolean types respectively. Atomic operations are performed through functions such as std::atomic_init(), std::atomic_load(), and std::atomic_store(). In the actual case, atomic operations are used to implement thread-safe counters to ensure thread safety when multiple threads access concurrently, and finally output the correct counter value.
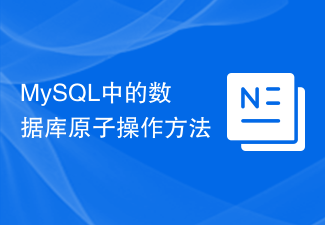
MySQL is a popular relational database management system (RDBMS) used to manage various types of data. In the database, an atomic operation refers to an operation that cannot be interrupted during execution. These operations are either all executed successfully or all fail, and only part of the operation is not executed. This is ACID (atomicity, consistency). , isolation, persistence) principle. In MySQL, you can use the following methods to implement atomic operations on the database. Transactions in MySQL
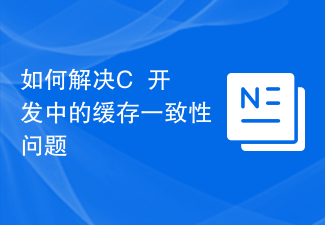
How to solve the cache consistency problem in C++ development In C++ development, the cache consistency problem is a common and important challenge. When threads in a multithreaded program execute on different processors, each processor has its own cache, and there may be data inconsistencies between these caches. This data inconsistency can lead to unexpected errors and undefined behavior of the program. Therefore, solving the cache consistency problem in C++ development is very critical. In C++, there are multiple ways to solve the cache coherency problem

Exploring the principles of Java multithreading: locking mechanism and thread safety Introduction: In the field of software development, multithreaded programming is a very important skill. By using multi-threading, we can perform multiple tasks at the same time and improve the performance and responsiveness of the program. However, multi-threaded programming also brings a series of challenges, the most important of which is thread safety. This article will explore the principles of Java multithreading, focusing on the locking mechanism and its role in thread safety. 1. What is thread safety? In a multi-threaded environment, if an operation does not cause any
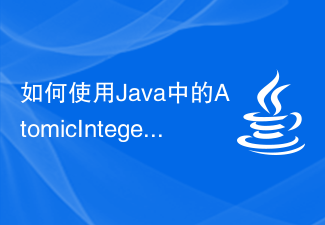
When writing multi-threaded applications, it is very important to consider thread safety. Ensuring thread safety, enabling multiple threads to work together, and improving program running efficiency is an issue worthy of full consideration. Java provides many atomic operation functions, including the atomic integer operation function - AtomicInteger. AtomicInteger is an atomic class in Java that can implement atomic operations on an integer variable. The so-called atomic operation means that there can only be
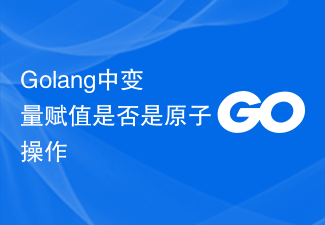
Whether variable assignment in Golang has atomic operations requires specific code examples. In programming, atomic operations refer to operations that cannot be interrupted, that is, either all of them are executed successfully or none of them are executed. In concurrent programming, the importance of atomic operations is self-evident, because in concurrent programs, multiple threads (or goroutines) may access and modify the same variable at the same time. If there are no atomic operations, race conditions will occur. As a programming language that supports concurrency, Golang also provides support for atomic operations. for
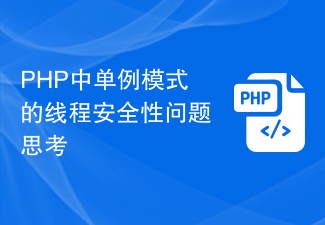
Thinking about thread safety issues of singleton mode in PHP In PHP programming, singleton mode is a commonly used design pattern. It can ensure that a class has only one instance and provide a global access point to access this instance. However, when using the singleton pattern in a multi-threaded environment, thread safety issues need to be considered. The most basic implementation of the singleton pattern includes a private constructor, a private static variable, and a public static method. The specific code is as follows: classSingleton{pr
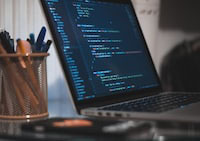
In computer science, concurrent programming is when a program can perform multiple tasks simultaneously. It is often used to fully utilize the computing power of multi-core processors and plays an important role in areas such as user interface, network communication, and database operations. However, concurrent programming also brings some challenges, the most important of which is how to ensure data consistency and program correctness when multiple threads access shared resources simultaneously. Java provides rich thread synchronization and mutual exclusion mechanisms to help developers solve challenges in concurrent programming. These mechanisms mainly include locks, atomic operations and the volatile keyword. Locks are used to protect shared resources. They allow one thread to monopolize a shared resource when accessing it, preventing other threads from accessing it at the same time, thus avoiding data inconsistency and program crashes.
