


How to choose the best golang framework for different application scenarios
Choose the best Go framework based on application scenarios: consider application type, language features, performance requirements, and ecosystem. Common Go frameworks: Gin (Web application), Echo (Web service), Fiber (high throughput), gorm (ORM), fasthttp (speed). Practical case: building REST API (Fiber) and interacting with database (gorm). Choose a framework: choose fasthttp for key performance, Gin/Echo for flexible web applications, and gorm for database interaction.
How to choose the best Go framework for different application scenarios
Introduction
The Go language is known for its speed, concurrency, and powerful standard library. It is widely used to develop a variety of applications, from web servers to distributed systems. To simplify application development and provide common functionality, the Go community has developed a wide range of frameworks. This guide is designed to help you choose the most appropriate Go framework for your specific application scenario.
Framework considerations
When choosing a Go framework, you need to consider the following key factors:
- Application type: The framework should support the type of application you are developing, such as a web application, API service, or CLI tool.
- Supported Language Features: The framework should support the Go language features you intend to use in your application, such as generics or concurrency.
- Performance Requirements: The performance requirements you have for your application will influence the framework you choose.
- Ecosystem and Support: An active community, rich documentation, and third-party library support are critical to the long-term success of the framework.
Common Go framework
- **Gin: Used to build fast, flexible web applications.
- **Echo: Another popular web framework known for its lightweight and scalability.
- **Fiber: A high-performance web framework optimized for extremely high throughput and low latency.
- **gorm: A powerful ORM (Object Relational Mapping) framework for interacting with databases.
- **fasthttp: A high-performance HTTP server and client library focused on speed and scalability.
Practical case
Building a simple REST API (Fiber)
package main import ( "log" "github.com/gofiber/fiber/v2" "github.com/gofiber/fiber/v2/middleware/logger" ) func main() { // 创建一个 Fiber 应用程序 app := fiber.New() // 使用 Logger 中间件记录请求 app.Use(logger.New()) // 定义一个处理 GET 请求的路由 app.Get("/api/v1/users", func(c *fiber.Ctx) error { return c.SendString("Hello, World!") }) // 监听端口 3000 log.Fatal(app.Listen("0.0.0.0:3000")) }
With database Interaction (gorm)
package main import ( "fmt" "gorm.io/driver/sqlite" "gorm.io/gorm" ) type User struct { ID uint Username string Email string } func main() { // 连接到 SQLite 数据库 db, err := gorm.Open(sqlite.Open("database.db"), &gorm.Config{}) if err != nil { log.Fatal(err) } // 创建一个新的用户 user := User{Username: "johndoe", Email: "johndoe@example.com"} db.Create(&user) // 查找所有用户 users := []User{} db.Find(&users) // 打印用户信息 fmt.Println("Users:", users) }
Choose the appropriate framework
In short, choosing the best Go framework depends on the specific requirements of the application scenario. For performance-critical applications, fasthttp is a good choice. For flexible and scalable web applications, Gin or Echo are a good choice. Gorm is a powerful ORM framework for applications that need to interact with databases. By considering framework considerations and evaluating real-world use cases, you can make informed choices and build successful Go applications.
The above is the detailed content of How to choose the best golang framework for different application scenarios. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


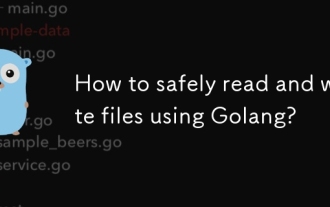
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
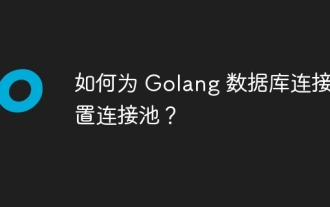
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
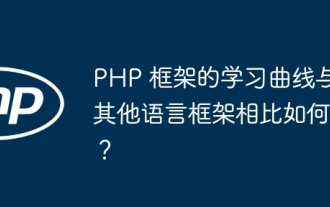
The learning curve of a PHP framework depends on language proficiency, framework complexity, documentation quality, and community support. The learning curve of PHP frameworks is higher when compared to Python frameworks and lower when compared to Ruby frameworks. Compared to Java frameworks, PHP frameworks have a moderate learning curve but a shorter time to get started.

The lightweight PHP framework improves application performance through small size and low resource consumption. Its features include: small size, fast startup, low memory usage, improved response speed and throughput, and reduced resource consumption. Practical case: SlimFramework creates REST API, only 500KB, high responsiveness and high throughput
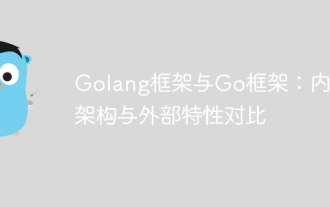
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
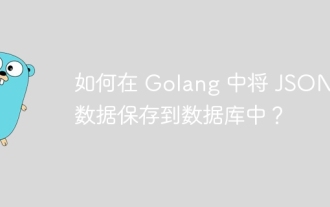
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
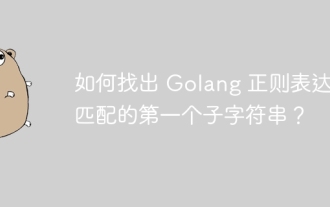
The FindStringSubmatch function finds the first substring matched by a regular expression: the function returns a slice containing the matching substring, with the first element being the entire matched string and subsequent elements being individual substrings. Code example: regexp.FindStringSubmatch(text,pattern) returns a slice of matching substrings. Practical case: It can be used to match the domain name in the email address, for example: email:="user@example.com", pattern:=@([^\s]+)$ to get the domain name match[1].
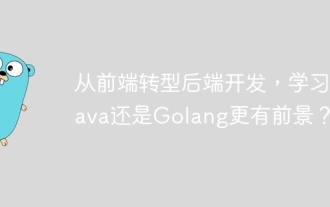
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
