Considerations for security of golang framework
Security considerations for the Go framework include: Input validation: Prevents malicious code injection. Session Management: Securely store and manage sensitive data. CSRF Protection: Prevent unauthorized operations. SQL injection protection: Prevent malicious database operations using parameterized queries. XSS Protection: Prevent malicious script execution through HTML escaping.
Go Framework Security Considerations
The Go framework is popular among developers for its ease of use and high performance, but just as important Consider its safety. Here are some key considerations for Go framework security:
1. Input Validation
The Go framework can help validate user-supplied input, such as form data or query parameters. This prevents attackers from exploiting applications by injecting malicious code.
Code Example:
package main import ( "fmt" "net/http" "strconv" "github.com/julienschmidt/httprouter" ) func main() { router := httprouter.New() router.POST("/update-user", func(w http.ResponseWriter, r *http.Request, _ httprouter.Params) { uid := r.FormValue("id") username := r.FormValue("username") // 将传入的 ID 转换为整数 id, err := strconv.Atoi(uid) if err != nil { http.Error(w, "Invalid user ID", http.StatusBadRequest) return } // 对输入进行进一步的验证和清理... }) }
2. Session Management
Session management is critical for tracking authorized users and protecting sensitive data. The Go framework provides session handlers that help you store and manage session data securely.
Code Example:
package main import ( "fmt" "net/http" "time" sessions "github.com/goincremental/negroni-sessions" "github.com/julienschmidt/httprouter" "github.com/urfave/negroni" ) func main() { router := httprouter.New() // 创建一个新的会话处理程序 store := sessions.NewCookieStore([]byte("secret-key")) sessionsMiddleware := sessions.Sessions("my-session", store) // 定义需要会话保护的路由 protectedRouter := negroni.New(negroni.HandlerFunc(sessionsMiddleware), httprouter.Router{}.Handler) protectedRouter.POST("/update-user", func(w http.ResponseWriter, r *http.Request, _ httprouter.Params) { session := sessions.GetSession(r) session.Set("last_active", time.Now()) session.Save() // 其余路由逻辑... }) }
3. Cross-Site Request Forgery (CSRF) Protection
CSRF attacks exploit the victim’s session or cookie to perform unintended Authorized operation. The Go framework provides CSRF protection middleware that can help prevent such attacks.
Code Example:
package main import ( "fmt" "net/http" "github.com/julienschmidt/httprouter" "github.com/rs/xid" "github.com/unrolled/secure" ) func main() { router := httprouter.New() // 创建一个新的安全处理程序 secureMiddleware := secure.New(secure.Options{ CSRF: &secure.CSRF{ Key: []byte("secret-key"), Form: "_csrf", }, }) // 为需要 CSRF 保护的路由应用中间件 csrfProtectedRouter := httprouter.Router{}.Handler csrfProtectedRouter = secureMiddleware.Handler(csrfProtectedRouter) csrfProtectedRouter.POST("/submit-form", func(w http.ResponseWriter, r *http.Request, _ httprouter.Params) { // 验证表单提交是否包含有效的 CSRF 令牌 // 其余路由逻辑... }) }
4. SQL Injection Protection
SQL injection attacks exploit vulnerable queries to perform unauthorized database operations . The Go framework's database connection pool and query builder can help prevent SQL injection attacks.
Code Example:
package main import ( "database/sql" "fmt" "log" _ "github.com/go-sql-driver/mysql" ) func main() { db, err := sql.Open("mysql", "username:password@tcp(localhost:3306)/database") if err != nil { log.Fatal(err) } defer db.Close() // 使用准备好的语句来执行参数化查询 stmt, err := db.Prepare("SELECT * FROM users WHERE username = ?") if err != nil { log.Fatal(err) } defer stmt.Close() username := "test-user" row := stmt.QueryRow(username) // 提取查询结果... }
5. Execute malicious scripts in the server. The Go framework provides XSS protection mechanisms such as templates and HTML escaping.
Code example: An online store is built using the Go framework, and the following security needs to be considered Factors: The above is the detailed content of Considerations for security of golang framework. For more information, please follow other related articles on the PHP Chinese website!package main
import (
"fmt"
"html/template"
"net/http"
)
func main() {
router := httprouter.New()
// 使用 HTML/Text 模板引擎,它可以自动转义 HTML 字符
tmpl := template.Must(template.ParseFiles("template.html"))
router.GET("/render-template", func(w http.ResponseWriter, r *http.Request, _ httprouter.Params) {
data := struct {
Message string
}{
Message: "<script>alert('Hello, XSS!');</script>",
}
// 将数据渲染到模板中
if err := tmpl.Execute(w, data); err != nil {
log.Fatal(err)
}
})
}

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


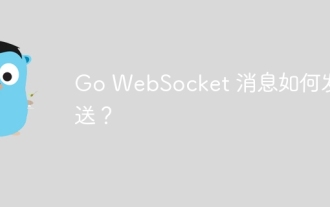
In Go, WebSocket messages can be sent using the gorilla/websocket package. Specific steps: Establish a WebSocket connection. Send a text message: Call WriteMessage(websocket.TextMessage,[]byte("Message")). Send a binary message: call WriteMessage(websocket.BinaryMessage,[]byte{1,2,3}).
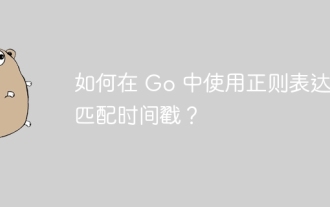
In Go, you can use regular expressions to match timestamps: compile a regular expression string, such as the one used to match ISO8601 timestamps: ^\d{4}-\d{2}-\d{2}T \d{2}:\d{2}:\d{2}(\.\d+)?(Z|[+-][0-9]{2}:[0-9]{2})$ . Use the regexp.MatchString function to check if a string matches a regular expression.
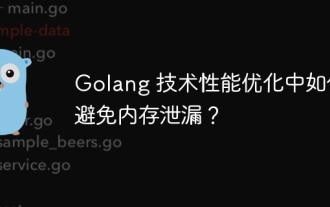
Memory leaks can cause Go program memory to continuously increase by: closing resources that are no longer in use, such as files, network connections, and database connections. Use weak references to prevent memory leaks and target objects for garbage collection when they are no longer strongly referenced. Using go coroutine, the coroutine stack memory will be automatically released when exiting to avoid memory leaks.
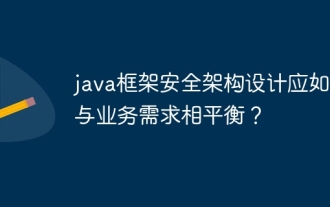
Java framework design enables security by balancing security needs with business needs: identifying key business needs and prioritizing relevant security requirements. Develop flexible security strategies, respond to threats in layers, and make regular adjustments. Consider architectural flexibility, support business evolution, and abstract security functions. Prioritize efficiency and availability, optimize security measures, and improve visibility.
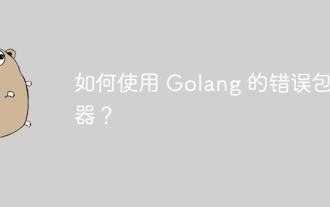
In Golang, error wrappers allow you to create new errors by appending contextual information to the original error. This can be used to unify the types of errors thrown by different libraries or components, simplifying debugging and error handling. The steps are as follows: Use the errors.Wrap function to wrap the original errors into new errors. The new error contains contextual information from the original error. Use fmt.Printf to output wrapped errors, providing more context and actionability. When handling different types of errors, use the errors.Wrap function to unify the error types.
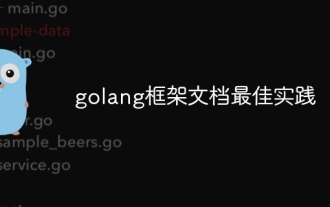
Writing clear and comprehensive documentation is crucial for the Golang framework. Best practices include following an established documentation style, such as Google's Go Coding Style Guide. Use a clear organizational structure, including headings, subheadings, and lists, and provide navigation. Provides comprehensive and accurate information, including getting started guides, API references, and concepts. Use code examples to illustrate concepts and usage. Keep documentation updated, track changes and document new features. Provide support and community resources such as GitHub issues and forums. Create practical examples, such as API documentation.
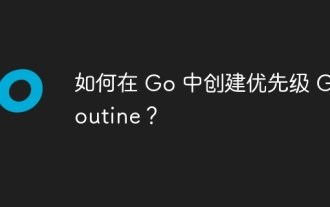
There are two steps to creating a priority Goroutine in the Go language: registering a custom Goroutine creation function (step 1) and specifying a priority value (step 2). In this way, you can create Goroutines with different priorities, optimize resource allocation and improve execution efficiency.
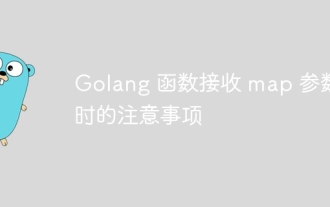
When passing a map to a function in Go, a copy will be created by default, and modifications to the copy will not affect the original map. If you need to modify the original map, you can pass it through a pointer. Empty maps need to be handled with care, because they are technically nil pointers, and passing an empty map to a function that expects a non-empty map will cause an error.
