Defense against cross-site scripting attacks in Java framework
XSS defense in the Java framework mainly includes HTML escaping, Content Security Policy (CSP) and X-XSS-Protection headers. Among them, HTML escaping prevents user input from being interpreted as HTML code and executed by converting it into HTML entities.
Cross-site scripting attack defense in Java framework
Cross-site scripting attack (XSS) is a common and dangerous A cybersecurity vulnerability that allows an attacker to inject malicious code into a user's browser. These codes can steal sensitive information, take control of the victim's browser, or redirect to malicious websites.
XSS Defense in Java Framework
The Java ecosystem provides a variety of defense mechanisms against XSS attacks. The most important of these are:
- HTML Escape: HTML escape user input before outputting it to the web page. This means converting special characters (e.g. , &) into HTML entities (e.g. , &).
- Content Security Policy (CSP): This is a set of rules implemented by web browsers to restrict the loading of content from external sources. The execution of malicious scripts can be blocked through CSP.
- X-XSS-Protection Header: This is an HTTP header that instructs the browser to enable or disable XSS filtering. Enabling XSS filtering can block many types of XSS attacks.
Practical case
Let us take a Spring Boot application as an example to demonstrate how to defend against XSS attacks:
import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RequestParam; import org.springframework.web.bind.annotation.RestController; import org.springframework.web.util.HtmlUtils; @RestController public class XSSController { @GetMapping("/xss") public String xss(@RequestParam(required = false) String input) { // HTML转义用户输入 String escapedInput = HtmlUtils.htmlEscape(input); return "<h1 id="输入">输入:</h1><br>" + escapedInput; } }
In this example , HtmlUtils.htmlEscape()
method is used to HTML escape user input, thereby preventing it from being interpreted as HTML code and executed.
By implementing these defenses, Java developers can protect their applications from XSS attacks, thereby enhancing their security.
The above is the detailed content of Defense against cross-site scripting attacks in Java framework. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
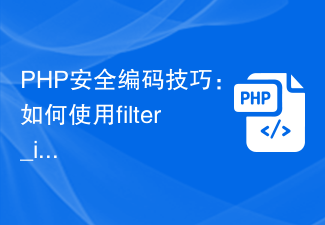
PHP secure coding tips: How to use the filter_input function to prevent cross-site scripting attacks In today's era of rapid Internet development, network security issues have become increasingly serious. Among them, cross-site scripting (XSS) is a common and dangerous attack method. To keep the website and users safe, developers need to take some precautions. This article will introduce how to use the filter_input function in PHP to prevent XSS attacks. learn
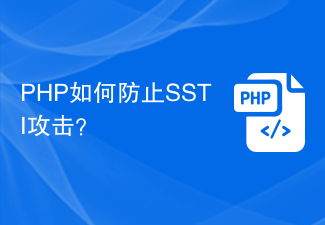
How to use PHP to defend against Server-SideTemplateInjection (SSTI) attacks Introduction: Server-SideTemplateInjection (SSTI) is a common web application security vulnerability. An attacker can cause the server to execute arbitrary code by injecting malicious code into the template engine, thereby causing Serious safety hazard. In PHP applications, SST can be exposed when user input is not handled correctly
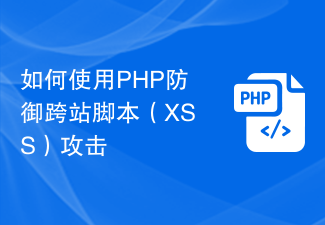
How to Use PHP to Defend Cross-Site Scripting (XSS) Attacks With the rapid development of the Internet, Cross-SiteScripting (XSS) attacks are one of the most common network security threats. XSS attacks mainly achieve the purpose of obtaining user sensitive information and stealing user accounts by injecting malicious scripts into web pages. To protect the security of user data, developers should take appropriate measures to defend against XSS attacks. This article will introduce some commonly used PHP technologies to defend against XSS attacks.
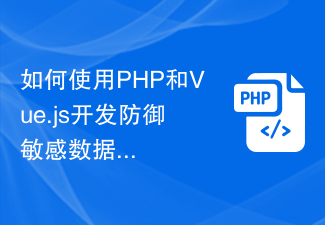
How to use PHP and Vue.js to develop applications that protect against sensitive data leakage In today's information age, the privacy and sensitive data of individuals and institutions face many security threats, one of the most common threats is data leakage. To prevent this risk, we need to pay attention to data security when developing applications. This article will introduce how to use PHP and Vue.js to develop an application that prevents sensitive data leakage, and provide corresponding code examples. Use a secure connection When transmitting data, make sure to use a secure connection.
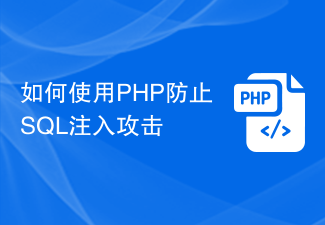
In the field of network security, SQL injection attacks are a common attack method. It exploits malicious code submitted by malicious users to alter the behavior of an application to perform unsafe operations. Common SQL injection attacks include query operations, insert operations, and delete operations. Among them, query operations are the most commonly attacked, and a common method to prevent SQL injection attacks is to use PHP. PHP is a commonly used server-side scripting language that is widely used in web applications. PHP can be related to MySQL etc.
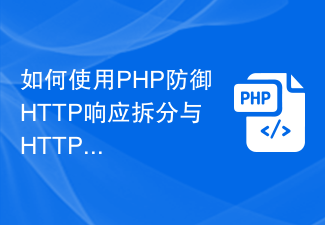
How to use PHP to defend against HTTP response splitting and HTTP parameter pollution attacks. With the continuous development of the Internet, network security issues are becoming more and more important. HTTP response splitting and HTTP parameter pollution attacks are common network security vulnerabilities, which can lead to risks of server attacks and data leakage. This article will introduce how to use PHP to defend against both forms of attacks. 1. HTTP response splitting attack HTTP response splitting attack means that the attacker sends specially crafted requests to cause the server to return multiple independent HTTP responses.
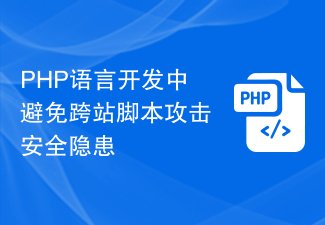
With the development of Internet technology, network security issues have attracted more and more attention. Among them, cross-site scripting (XSS) is a common network security risk. XSS attacks are based on cross-site scripting. Attackers inject malicious scripts into website pages to obtain illegal benefits by deceiving users or implanting malicious code through other methods, causing serious consequences. However, for websites developed in PHP language, avoiding XSS attacks is an extremely important security measure. because
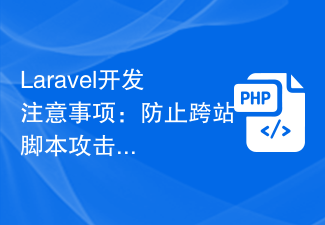
Laravel is a popular PHP framework that provides many useful features and tools that make web application development easier and faster. However, as web applications continue to grow in complexity, security issues become increasingly important. One of the most common and dangerous security vulnerabilities is cross-site scripting (XSS). In this article, we will cover methods and techniques to prevent cross-site scripting attacks to protect your Laravel application from XSS attacks. What is a cross-site scripting attack?
