


How to use Golang to build a RESTful API and connect to a MySQL database?
Use Golang to build RESTful API and connect to MySQL database: install Golang, MySQL driver and create project. Define API handlers, including getting all users and specific users. Connect to the MySQL database through the sql.Open function. Use db.Query and db.QueryRow to get data from the database. Use json.NewEncoder to write JSON responses. Optional: Provide code examples for creating new users.
How to use Golang to build a RESTful API and connect to the MySQL database
Introduction
RESTful API is a type of API based on the HTTP protocol that simplifies the interaction between the client and the server. Building RESTful APIs using Golang takes full advantage of its high performance and concurrency. This article will guide you on how to use Golang to build a RESTful API and connect to a MySQL database.
Prerequisites
- Install Golang (version 1.18 or higher)
- MySQL database and MySQL driver (
github. com/go-sql-driver/mysql
) - Text editor or IDE (such as Visual Studio Code)
Build RESTful API
-
Create a new project: Use the
go mod init <project name>
command to create a new project. -
Install the MySQL driver: Use the
go get -u github.com/go-sql-driver/mysql
command to install the MySQL driver. -
Write the API handler: Write the following API handler in the
main.go
file:
package main import ( "database/sql" "log" "net/http" "strconv" "github.com/go-sql-driver/mysql" ) const ( user = "root" password = "" host = "localhost" port = 3306 database = "my_db" ) var db *sql.DB func init() { dsn := fmt.Sprintf("%s:%s@tcp(%s:%d)/%s?parseTime=true", user, password, host, port, database) var err error db, err = sql.Open("mysql", dsn) if err != nil { log.Fatal(err) } } func main() { http.HandleFunc("/users", handleUsers) http.HandleFunc("/users/", handleUser) log.Fatal(http.ListenAndServe(":8080", nil)) }
- Connect to the MySQL database: Use the
sql.Open
function to connect to the MySQL database. - Define route handlers: Use the
http.HandleFunc
function to define two route handlers:/users
and/users/ :id
. - Running the server: Use the
http.ListenAndServe
function to start the server.
Handling user requests
- Get all users: In
/users
handler, Use thedb.Query
function to get all users from the database. - Get a specific user: In the
/users/:id
handler, use thedb.QueryRow
function to get a specific user from the database. - Writing the response: Use the
json.NewEncoder
function to encode user data into JSON and write it into the HTTP response.
Practical case
Assume that your MySQL database has a table named users
, with the following structure:
CREATE TABLE users ( id INT NOT NULL AUTO_INCREMENT, name VARCHAR(255) NOT NULL, email VARCHAR(255) NOT NULL, PRIMARY KEY (id) );
You can use the following code to create a new user and add it to the database:
func handleCreateUser(w http.ResponseWriter, r *http.Request) { var user User if err := json.NewDecoder(r.Body).Decode(&user); err != nil { http.Error(w, "Invalid JSON", http.StatusBadRequest) return } stmt, err := db.Prepare("INSERT INTO users (name, email) VALUES (?, ?);") if err != nil { log.Fatal(err) } defer stmt.Close() res, err := stmt.Exec(user.Name, user.Email) if err != nil { log.Fatal(err) } id, err := res.LastInsertId() if err != nil { log.Fatal(err) } user.ID = int(id) json.NewEncoder(w).Encode(user) }
Note: Don’t forget to update the database connection information and the handling mechanism related to cross-domain requests.
The above is the detailed content of How to use Golang to build a RESTful API and connect to a MySQL database?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
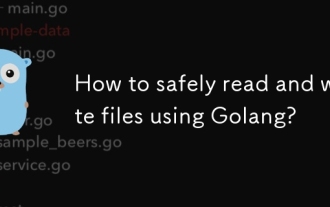
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
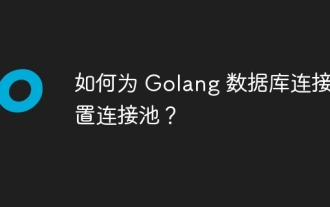
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
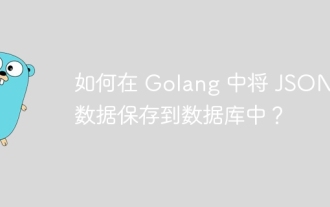
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
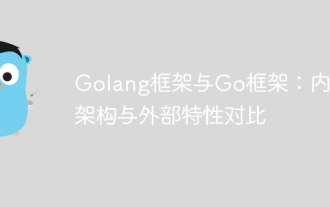
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
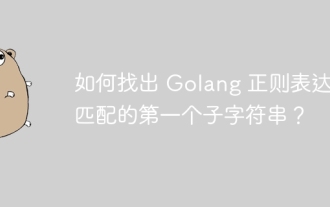
The FindStringSubmatch function finds the first substring matched by a regular expression: the function returns a slice containing the matching substring, with the first element being the entire matched string and subsequent elements being individual substrings. Code example: regexp.FindStringSubmatch(text,pattern) returns a slice of matching substrings. Practical case: It can be used to match the domain name in the email address, for example: email:="user@example.com", pattern:=@([^\s]+)$ to get the domain name match[1].
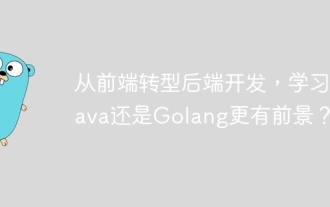
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
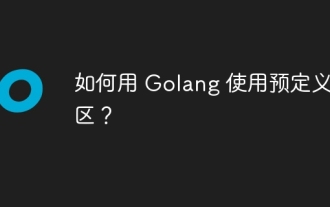
Using predefined time zones in Go includes the following steps: Import the "time" package. Load a specific time zone through the LoadLocation function. Use the loaded time zone in operations such as creating Time objects, parsing time strings, and performing date and time conversions. Compare dates using different time zones to illustrate the application of the predefined time zone feature.
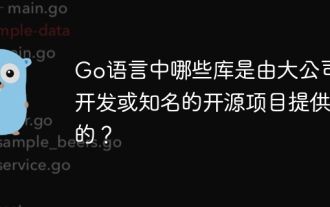
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
