Advanced PHP features: Use Traits to reuse code cleverly
Traits in PHP is a feature that allows code reuse without inheritance. Key benefits include: Code Reuse: Share code between different classes, reducing duplication. Flexibility: Can be added to a class at any time without having to rewrite or extend the class. Avoid multiple inheritance: Provide alternatives to code reuse, eliminating the complexity and risk of multiple inheritance.
Advanced PHP Features: Use Traits to Reuse Code Cleverly
Introduction
## Traits in #PHP are a powerful feature that allow developers to share code and functionality without using inheritance. This article will explore how Traits work and show how to use them skillfully for code reuse through practical cases.Using Traits
To declare a Trait, use thetrait keyword, as follows:
trait ExampleTrait { public function doSomething() { // ... } }
class ExampleClass { use ExampleTrait; }
ExampleClass access to all methods and properties defined in
ExampleTrait.
Practical Case: Logging Objects
Suppose we have a hierarchy of objects, and we want to provide a logging method for each object in it. We can create a Trait to handle logging, as shown below:trait LoggableTrait { protected $logger; public function setLogger(LoggerInterface $logger) { $this->logger = $logger; } public function log(string $message) { if ($this->logger !== null) { $this->logger->log($message); } } }
class ExampleObject { use LoggableTrait; // ... }
Advantages
The advantages of using Traits include:- Code reuse: Traits allow for reuse between different classes Share code among users to reduce duplication.
- Flexibility: Traits can be added to a class at any time without rewriting or extending the class.
- Avoid multiple inheritance: Traits provide an alternative to multiple inheritance for code reuse, thus avoiding the complexity and risks caused by multiple inheritance.
Conclusion
Traits is a powerful feature in PHP that provides a flexible and scalable code reuse mechanism. By using traits, developers can easily share common functionality across multiple objects and avoid code duplication and complexity.The above is the detailed content of Advanced PHP features: Use Traits to reuse code cleverly. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
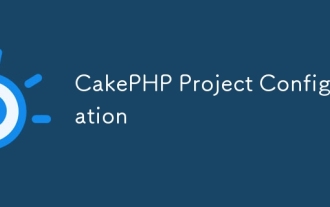
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
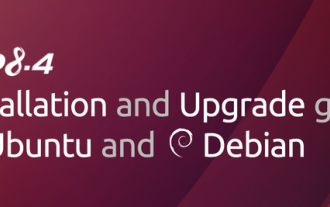
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
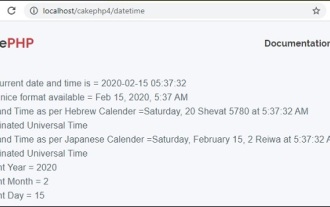
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
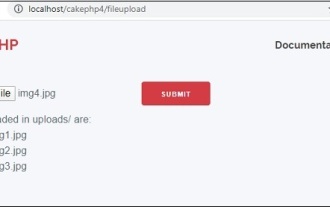
To work on file upload we are going to use the form helper. Here, is an example for file upload.
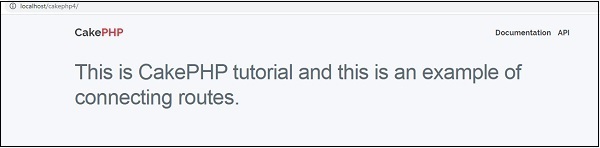
In this chapter, we are going to learn the following topics related to routing ?
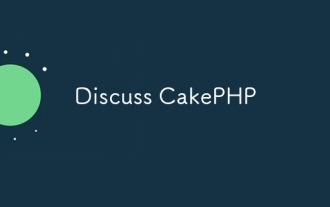
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
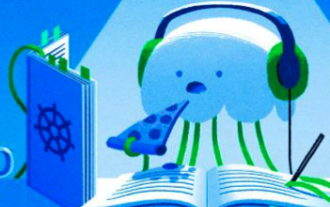
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
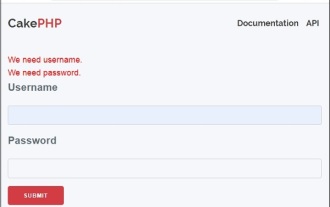
Validator can be created by adding the following two lines in the controller.
