What are the best practices for error handling in Golang framework?
Best practice: Create custom errors using well-defined error types (errors package) Provide more details Properly log errors Properly propagate errors, avoid hiding or suppressing Wrap errors as needed to add context
Best Practices for Error Handling in the Go Framework
Handling errors in Go applications is critical to writing stable, robust code. The Go standard library provides built-in support for error handling, but different frameworks can have their own best practices. This article describes some guidelines for optimal error handling when using the Go framework.
1. Use error types
It is recommended to use clearly defined error types, which can simplify error handling and improve readability. Error types can be created through the New
or Errorf
functions in the errors
package.
For example:
import "errors" var ErrNotFound = errors.New("not found")
2. Using custom errors
Writing your own error types in the framework can provide more detailed error types than the built-in error types. information. This allows applications to handle errors in a more meaningful way.
For example:
type MyError struct { Message string } func (e MyError) Error() string { return e.Message }
3. Proper logging
An important aspect of error handling is logging. The framework should provide logging functionality to record all errors that occur. This helps debug issues and track application behavior.
For example, use the log
package:
import "log" func main() { log.Fatal(ErrNotFound) }
4. Error propagation
The function should propagate errors correctly, allowing upper-layer functions deal with them. Avoid hiding or suppressing errors as it makes debugging difficult.
func GetResource() (*Resource, error) { db, err := connectToDB() if err != nil { return nil, err } resource, err := db.GetResource() if err != nil { return nil, err } return resource, nil }
5. Error packaging
Sometimes, it is necessary to add contextual information to an existing error. Error wrapping (also called error accumulation) allows adding additional layers of errors on top of the original error.
import "fmt" func GetResource() (*Resource, error) { resource, err := db.GetResource() if err != nil { return nil, fmt.Errorf("failed to get resource: %w", err) } return resource, nil }
Practical Case
Consider a REST API built using the Gin framework that handles errors from the database:
import ( "errors" "github.com/gin-gonic/gin" ) var ErrNotFound = errors.New("not found") func GetResource(c *gin.Context) { db, err := connectToDB() if err != nil { c.JSON(http.StatusInternalServerError, gin.H{"error": err.Error()}) return } resource, err := db.GetResource(c.Param("id")) if err == ErrNotFound { c.JSON(http.StatusNotFound, gin.H{"error": "resource not found"}) return } if err != nil { c.JSON(http.StatusInternalServerError, gin.H{"error": err.Error()}) return } c.JSON(http.StatusOK, resource) }
This example demonstrates :
- Use custom error types
ErrNotFound
- Properly propagate errors, returning them to Gin's response handler
- According to the error type Return the appropriate HTTP status code
The above is the detailed content of What are the best practices for error handling in Golang framework?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


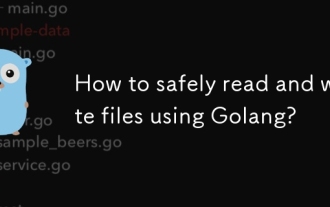
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
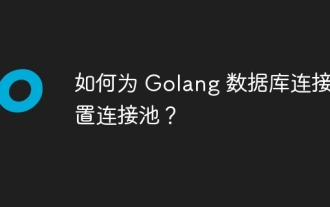
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
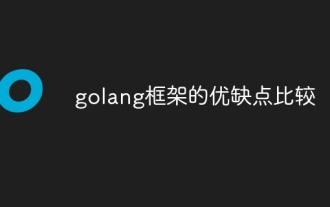
The Go framework stands out due to its high performance and concurrency advantages, but it also has some disadvantages, such as being relatively new, having a small developer ecosystem, and lacking some features. Additionally, rapid changes and learning curves can vary from framework to framework. The Gin framework is a popular choice for building RESTful APIs due to its efficient routing, built-in JSON support, and powerful error handling.
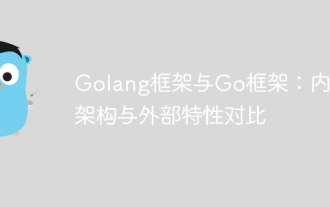
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
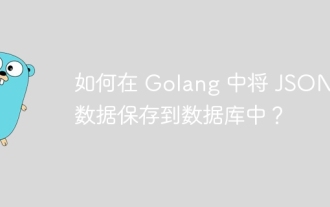
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
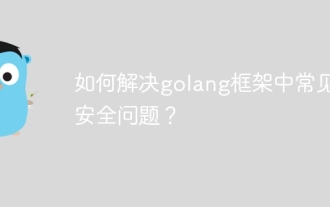
How to address common security issues in the Go framework With the widespread adoption of the Go framework in web development, ensuring its security is crucial. The following is a practical guide to solving common security problems, with sample code: 1. SQL Injection Use prepared statements or parameterized queries to prevent SQL injection attacks. For example: constquery="SELECT*FROMusersWHEREusername=?"stmt,err:=db.Prepare(query)iferr!=nil{//Handleerror}err=stmt.QueryR
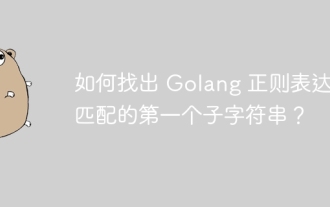
The FindStringSubmatch function finds the first substring matched by a regular expression: the function returns a slice containing the matching substring, with the first element being the entire matched string and subsequent elements being individual substrings. Code example: regexp.FindStringSubmatch(text,pattern) returns a slice of matching substrings. Practical case: It can be used to match the domain name in the email address, for example: email:="user@example.com", pattern:=@([^\s]+)$ to get the domain name match[1].
