Future trends and emerging technologies of golang framework
Future trends of the Go framework include microservice architecture (practical case: using Gin to build microservices), cloud computing (practical case: using Go Cloud SDK to access Google Cloud Storage), and artificial intelligence and machine learning (practical case: using TensorFlow train machine learning models).
Future Trends and Emerging Technologies of Go Framework
In the ever-changing world of software development, Go Framework is known for its excellent performance , concurrency and type safety. As technology continues to develop, the Go framework is also developing and evolving. This article will explore the future trends and emerging technologies of the Go framework and provide practical cases to demonstrate the application of these technologies.
Trend 1: Microservice architecture
Microservice architecture is gradually becoming the preferred method for building complex systems. The Go framework is ideal for microservice development due to its lightweight and high performance. Microservices built with Go can be deployed, managed, and scaled independently, increasing agility and reliability.
Practical case: Building microservices using Gin
Gin is a popular Go web framework known for its simplicity, ease of use, and high performance. It is ideal for building RESTful APIs and microservices. The following code shows how to use Gin to create a simple microservice:
package main import ( "github.com/gin-gonic/gin" ) func main() { r := gin.Default() r.GET("/ping", func(c *gin.Context) { c.JSON(200, gin.H{ "message": "pong", }) }) r.Run() }
Trend 2: Cloud Computing
Cloud computing is changing the way software is developed, and the Go framework is Ideal for building cloud applications. Go’s native concurrency and high performance make it ideal for handling high loads in cloud environments.
Practical case: Using Go Cloud SDK to access Google Cloud Storage
Go Cloud SDK provides a client library that can easily interact with Google Cloud Storage. The following code shows how to upload a file to a bucket using the Go Cloud SDK:
import ( "context" "fmt" "cloud.google.com/go/storage" ) func main() { ctx := context.Background() client, err := storage.NewClient(ctx) if err != nil { // Handle error. } wc := client.Bucket("my-bucket").Object("my-object").NewWriter(ctx) if _, err := wc.Write([]byte("Hello, Cloud Storage!")); err != nil { // Handle error. } if err := wc.Close(); err != nil { // Handle error. } fmt.Println("File uploaded to Cloud Storage.") }
Trend 3: Artificial Intelligence and Machine Learning
Artificial intelligence and machine learning technologies are growing rapidly With its popularity, the Go framework has also begun to be used in these fields. Go's excellent concurrency and high performance make it ideal for processing large amounts of data and computationally intensive tasks.
Practical case: Using TensorFlow to train a machine learning model
TensorFlow is a popular machine learning library that can be used in the Go language. The following code shows how to train a simple linear regression model using TensorFlow:
import ( "fmt" "github.com/tensorflow/tensorflow/tensorflow/go" "github.com/tensorflow/tensorflow/tensorflow/go/op" ) func main() { // Create a TensorFlow graph. g := tensorflow.NewGraph() // Define the input data. x := op.Placeholder(g, tensorflow.Float, tensorflow.Shape{1}) y := op.Placeholder(g, tensorflow.Float, tensorflow.Shape{1}) // Define the model parameters. w := op.Variable(g, tensorflow.Float, tensorflow.Shape{1, 1}) b := op.Variable(g, tensorflow.Float, tensorflow.Shape{1}) // Define the loss function. loss := op.Mean(g, op.Square(op.Sub(g, op.MatMul(g, w, x), op.Add(g, b, y)))) // Create a session to run the graph. sess, err := tensorflow.NewSession(g, nil) if err != nil { // Handle error. } // Train the model. for i := 0; i < 1000; i++ { // Generate training data. xData := make([]float32, 1) yData := make([]float32, 1) for j := range xData { xData[j] = float32(j) yData[j] = float32(2 * j) } // Train the model. if err := sess.Run(nil, []tensorflow.Tensor{ x.Value(xData), y.Value(yData), }, []tensorflow.Tensor{loss.Op.Output(0)}, nil); err != nil { // Handle error. } } // Get the trained parameters. wVal, err := sess.Run(nil, nil, []tensorflow.Tensor{w.Op.Output(0)}, nil) if err != nil { // Handle error. } bVal, err := sess.Run(nil, nil, []tensorflow.Tensor{b.Op.Output(0)}, nil) if err != nil { // Handle error. } // Print the trained parameters. fmt.Printf("w: %v\n", wVal) fmt.Printf("b: %v\n", bVal) }
Conclusion
The future of the Go framework is bright. As trends such as microservices, cloud computing, and artificial intelligence take hold, the Go framework will continue to be the technology of choice for building high-performance, scalable, and reliable applications. This article shows these trends in action and provides insights into the future development of the Go framework.
The above is the detailed content of Future trends and emerging technologies of golang framework. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










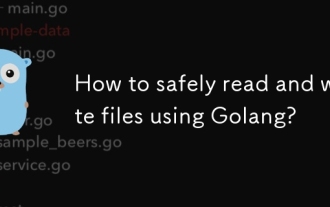
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
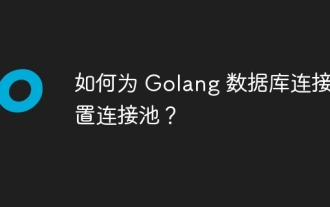
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
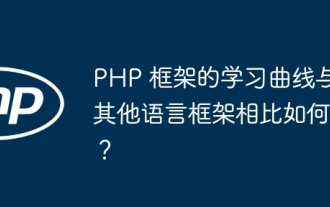
The learning curve of a PHP framework depends on language proficiency, framework complexity, documentation quality, and community support. The learning curve of PHP frameworks is higher when compared to Python frameworks and lower when compared to Ruby frameworks. Compared to Java frameworks, PHP frameworks have a moderate learning curve but a shorter time to get started.
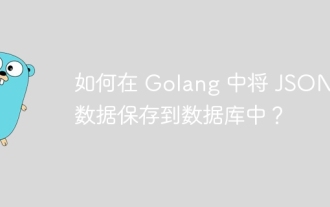
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
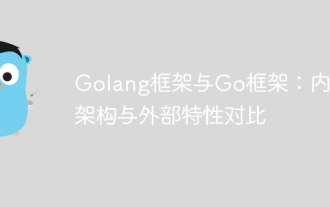
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
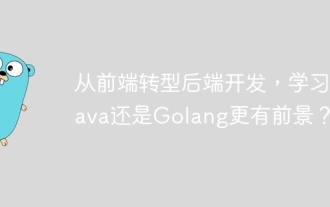
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
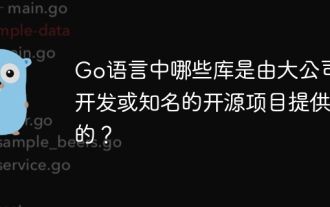
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
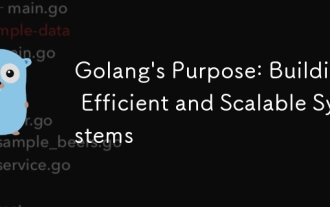
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
