


Application of debugging technology in C++ algorithm efficiency optimization
Debugging techniques can help optimize the efficiency of C++ algorithms through the use of log statements, breakpoints, single-stepping, and performance analysis tools. Practical examples include optimizing the bubble sort algorithm and improving performance by introducing the isSorted flag to avoid unnecessary loops.
Application of debugging technology in C++ algorithm efficiency optimization
In C++ algorithm development, debugging technology is crucial. It can help identify and solve efficiency bottlenecks to optimize algorithm performance. The following are some commonly used debugging techniques and practical cases:
1. Use log statements
Log statements can output key information during algorithm execution to help locate problems. For example:
// 定义一个日志函数 void log(const std::string& message) { std::cout << "[LOG] " << message << std::endl; } int main() { log("开始算法"); // 算法代码 log("算法结束"); return 0; }
2. Using breakpoints and single-stepping
The breakpoints and single-stepping functions in the debugger can be used to inspect the algorithm execution line by line. For example:
- ##Breakpoint: Set a breakpoint at the line of code that needs to be checked, and the program will pause when it reaches the breakpoint.
- Single-step execution: Execute the algorithm step by step, and you can observe changes in variable values and execution processes.
3. Use performance analysis tools
Performance analysis tools can analyze the execution time and resource usage of the code to identify efficiency bottlenecks. For example:- Visual Studio: You can use the built-in performance analyzer. gprof: A command line tool that can be used to analyze function calls and profiling information of a program.
Practical case: Optimizing sorting algorithm
The following is a practical case of optimizing the bubble sorting algorithm:// 未优化的冒泡排序 void bubbleSort(int* arr, int n) { for (int i = 0; i < n; ++i) { for (int j = 0; j < n - i - 1; ++j) { if (arr[j] > arr[j + 1]) { swap(arr[j], arr[j + 1]); } } } } // 优化的冒泡排序 void bubbleSortOptimized(int* arr, int n) { bool isSorted = false; while (!isSorted) { isSorted = true; for (int j = 0; j < n - 1; ++j) { if (arr[j] > arr[j + 1]) { swap(arr[j], arr[j + 1]); isSorted = false; } } } }
isSorted flag is introduced. When no elements need to be exchanged, the flag becomes true to avoid unnecessary loops.
The above is the detailed content of Application of debugging technology in C++ algorithm efficiency optimization. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


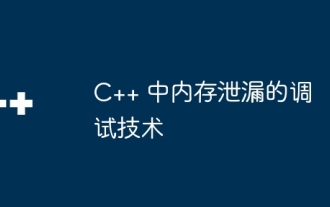
A memory leak in C++ means that the program allocates memory but forgets to release it, causing the memory to not be reused. Debugging techniques include using debuggers (such as Valgrind, GDB), inserting assertions, and using memory leak detector libraries (such as Boost.LeakDetector, MemorySanitizer). It demonstrates the use of Valgrind to detect memory leaks through practical cases, and proposes best practices to avoid memory leaks, including: always releasing allocated memory, using smart pointers, using memory management libraries, and performing regular memory checks.
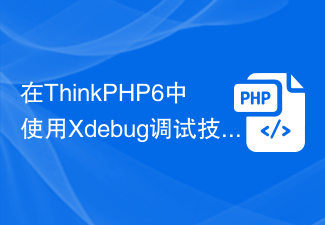
ThinkPHP6 is a popular PHP framework that uses a variety of technologies to make development more convenient. One such technology is debugging tools such as Xdebug. In this article, we will explore how to use Xdebug for debugging in ThinkPHP6. Install and configure Xdebug Before you start using Xdebug, you first need to install and enable it. In the php.ini file, you can add the following configuration: [xdebug]zend_extension=x
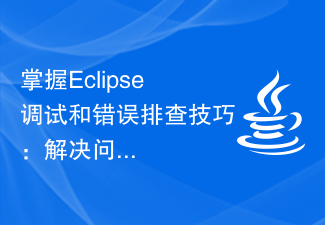
A powerful tool for solving problems: Mastering Eclipse debugging and error troubleshooting techniques requires specific code examples. Introduction: In our daily development process, we often encounter various problems. Some problems are easy to find and solve, but others give us a lot of headaches. In order to effectively solve these problems, it is very important to master Eclipse debugging and error troubleshooting techniques. This article will introduce Eclipse debugging and error troubleshooting technology in detail and give specific code examples. 1. What is Eclipse debugging and errors?
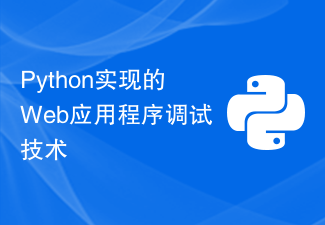
With the development of web applications, the problems encountered during the development process have become increasingly complex and diverse. Debugging is a necessary and common link, which can help developers quickly locate and solve problems and improve development efficiency. As one of the most popular programming languages currently, Python also occupies a very important position in web application development. In this article, we will introduce web application debugging technologies implemented in Python and how to use these technologies to quickly locate and solve problems. 1. Python application tuning
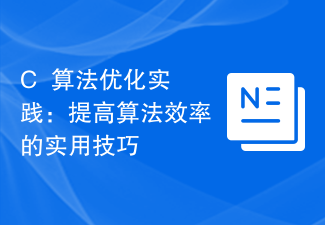
With the increasing popularity of computer applications, algorithm efficiency has become a concern for more and more programmers. For high-level languages like C++, although its compiler can perform certain optimizations, in actual application scenarios, algorithm efficiency optimization still plays a crucial role. This article will introduce some practical techniques for C++ algorithm optimization to help readers improve algorithm efficiency. Algorithm selection: First consider adopting a suitable algorithm, which is the most basic optimization method. For non-routine problems, we should comprehensively consider data size, time complexity, space
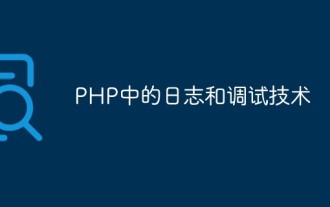
PHP is a widely used programming language that is flexible and easy to learn. Logging and debugging techniques are indispensable when developing and maintaining web applications. In PHP, there are many ways to implement logging and debugging. These techniques can help developers track errors and debug code. 1. Logging technology 1.1 The role of logs Logs are a means of recording events that occur when an application is running. It can help developers understand the decisions made by the application at different points in time. 1.2PHP’s logging system
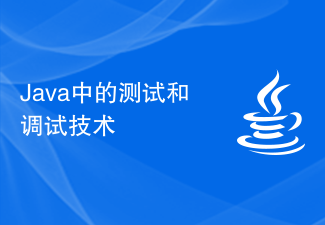
Java is a very popular programming language because it is portable, easy to learn and use, and has a strong community support. Testing and debugging are inevitable steps in writing quality software. In this article, we will explore testing and debugging techniques in Java to help you better understand how to write reliable Java applications. 1. Testing technology Testing refers to evaluating and verifying the correctness, integrity, validity, reliability, security and other quality attributes of the software through various means at different stages of software development.
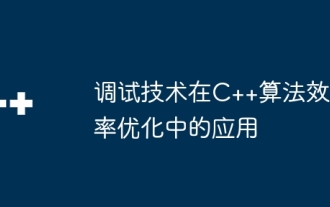
Debugging techniques can help optimize the efficiency of C++ algorithms through the use of logging statements, breakpoints, single-stepping, and performance analysis tools. Practical cases include optimizing the bubble sort algorithm and improving performance by introducing the isSorted flag to avoid unnecessary loops.
