Part 1 - Object-Oriented Analysis and Design
1. Object-Oriented Thinking
Object-oriented thinking is fundamental for object-oriented modelling, which is a core aspect of this post. It involves understanding problems and concepts by decomposing them into component parts and considering these parts as objects.
-
Definition: Object-oriented thinking means viewing various elements as discrete objects. For example, in a software system, a tweet, a user, or a product can be viewed as objects.
-
Attributes and Behaviours:
-
Attributes: Properties or characteristics of an object (e.g., a person's name, age, height).
-
Behaviours: Actions that an object can perform (e.g., a device powering on or off, a user logging in).
-
Benefits:
-
Organization: Objects encapsulate both data and behaviour, keeping related details and functions together.
-
Flexibility: Changes to an object’s attributes or behaviours can be made independently of other objects.
-
Reusability: Objects can be reused across different parts of a program or even in different programs, reducing the amount of code that needs to be written and maintained.
2. Design in the Software Process
The design phase is critical in the software development lifecycle. It ensures that the final product meets user requirements and functions as intended.
-
Software Development Process: The software development process is iterative and involves several key stages:
-
Requirements Gathering: Understanding what the client or user needs from the software.
-
Conceptual Design: Developing high-level design outlines and mock-ups.
-
Technical Design: Creating detailed specifications for each component.
-
Implementation: Writing the actual code based on the designs.
-
Testing: Verifying that the software works correctly and meets requirements.
-
Deployment: Releasing the software for use.
-
Maintenance: Ongoing updates and bug fixes.
-
Importance of Design: Skipping or inadequately addressing design phases can lead to project failure. A solid design foundation ensures that the software development starts on the right track and reduces the risk of costly changes later on.
3. Requirements
Requirements gathering is the foundation of a successful project. It involves understanding what the client or user needs from the software.
-
Definition: Requirements are the conditions or capabilities that the software must satisfy.
-
Elicitation:
-
Client Interviews: Direct discussions with the client to understand their vision and needs.
-
Questionnaires and Surveys: Collecting structured information from potential users or stakeholders.
-
Observation: Watching how users interact with current systems to identify needs and pain points.
-
Workshops: Collaborative sessions with stakeholders to gather and prioritize requirements.
-
Trade-offs: Clients may need to balance different needs and constraints. For instance, they might need to choose between more features or faster delivery.
Example: When designing a house, the architect gathers requirements by asking detailed questions about the homeowner’s preferences for room sizes, placements, and specific features. This helps prevent costly changes during construction.
4. Design
Design in software development involves creating both conceptual and technical blueprints that guide the implementation phase.
-
Conceptual Design:
-
Definition: High-level outline of the software’s major components and their responsibilities.
-
Mock-ups and Wireframes: Visual representations that help stakeholders understand and approve the design before detailed work begins.
-
Responsibilities: Defining what each component of the software is supposed to do.
-
Examples:
-
Mock-ups: Visual layouts of user interfaces showing how screens will look and function.
-
Wireframes: Simple sketches or diagrams showing the layout of components without detailed design elements.
-
Importance: Ensures all stakeholders have a clear understanding and agreement on the high-level structure of the software.
Example: In building a house, the conceptual design outlines the general layout of rooms and their connections but does not yet detail the plumbing or wiring.
-
Technical Design:
-
Definition: Detailed specifications of each component, including how they will be built and interact.
-
Technical Diagrams: Detailed drawings showing how components fit together and how data flows between them.
-
Breakdown of Components: Further decomposing high-level components into smaller, manageable parts until each can be implemented.
-
Examples:
-
Class Diagrams: Show the structure of classes, their attributes, methods, and relationships.
-
Sequence Diagrams: Illustrate how objects interact in a particular sequence of events.
-
Component Diagrams: Depict the organization and dependencies among components.
-
Importance: Provides developers with the detailed information they need to write code effectively and ensures consistency across the development team.
Example: In house construction, the technical design specifies the exact materials for walls, floors, and roofs, as well as the detailed plans for plumbing and electrical systems.
5. Compromise in Requirements and Design
Throughout the design process, compromises are often necessary to balance client needs and project constraints.
-
Communication: Constant feedback loops with clients are essential to ensure the design remains aligned with their vision and constraints.
-
Iterative Reviews: Regularly reviewing and refining designs with client input.
-
Prototyping: Building early versions of components to test and validate ideas with clients.
-
Reworking: Both conceptual and technical designs may need to be revised if they do not meet requirements or prove unfeasible.
-
Flexibility: Being open to changes and adjustments as new information emerges or as requirements evolve.
-
Impact Analysis: Evaluating the potential impact of changes on the overall project to make informed decisions.
Example: If a client wants an open kitchen but structural needs require a supporting beam, the architect and client must find a compromise that maintains structural integrity while satisfying the client’s aesthetic preferences.
6. Design for Quality Attributes
Designing software involves balancing various quality attributes to meet both functional and non-functional requirements.
-
Quality Attributes: Characteristics that affect the performance, usability, and maintainability of software.
-
Performance: How fast and efficiently the software performs its tasks.
-
Security: Measures taken to protect the software from threats and vulnerabilities.
-
Scalability: The ability of the software to handle increased load or usage.
-
Maintainability: How easily the software can be updated or modified.
-
Usability: The ease with which users can learn and use the software.
-
Trade-offs: Balancing these attributes often involves trade-offs, as optimizing for one attribute can affect others.
-
Performance vs. Security: Enhancing security measures can sometimes slow down performance.
-
Scalability vs. Usability: Adding features to improve scalability might complicate the user interface.
-
Context: The specific context of the software influences how these attributes are balanced.
-
Critical Systems: Prioritize reliability and security over other attributes.
-
Consumer Applications: Emphasize usability and performance to enhance user satisfaction.
Example: When designing a front door, balancing security (sturdy locks) with convenience (ease of access) is crucial. Too many locks make the door secure but inconvenient, while too few locks make it convenient but less secure.
7. Class Responsibility Collaborator (CRC) Cards
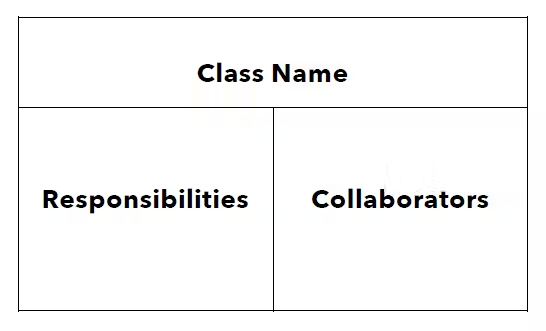
CRC cards are a tool used to identify and organize classes, their responsibilities, and collaborators in the design process.
-
Definition: CRC cards help in visualizing and organizing the responsibilities of different classes and how they interact with each other.
-
Class: Represents an object or concept in the system.
-
Responsibility: Defines what the class knows and does.
-
Collaborator: Other classes with which the class interacts.
-
Usage:
-
Brainstorming: Helps teams brainstorm and identify necessary classes and their roles.
-
Design Sessions: Facilitates discussions about class responsibilities and interactions.
-
Documentation: Serves as a documentation tool to capture design decisions.
-
Process:
-
Identify Classes: List all potential classes involved in the system.
-
Define Responsibilities: Write down the main responsibilities of each class.
-
Identify Collaborators: Determine which classes each class needs to interact with to fulfil its responsibilities.
-
Benefits:
-
Clarity: Provides a clear and concise way to organize and communicate design ideas.
-
Flexibility: Easy to update and modify as the design evolves.
-
Collaboration: Enhances team collaboration by making it easy to discuss and refine design decisions.
Example: In a banking application, a CRC card for the "Account" class might list responsibilities like "manage balance" and "track transactions," with collaborators like "Customer" and "Transaction" classes.
8. Prototyping and Simulation
Prototyping and simulation techniques are used to test and refine designs early in the process, helping to identify and fix issues before full-scale development.
-
Prototyping:
-
Low-Fidelity Prototypes: Simple, rough versions of the software or specific components, often created with paper or basic digital tools.
-
High-Fidelity Prototypes: More detailed and interactive versions that closely resemble the final product.
-
Purpose: Validate design concepts, gather user feedback, and identify usability issues.
-
Methods:
-
Paper Prototyping: Creating hand-drawn sketches of user interfaces and interactions.
-
Digital Prototyping: Using software tools to create interactive mock-ups and simulations.
-
Simulation:
-
Definition: Running models to test the behaviour and performance of a design under various conditions.
-
Use Cases: Evaluating system performance, load testing, and validating design decisions.
-
Benefits:
-
Early Validation: Identifies potential issues before full-scale development.
-
Cost-Effective: Reduces the risk of costly changes by addressing issues early.
-
User Feedback: Allows users to interact with the prototype and provide feedback on functionality and usability.
-
Tools: Various software tools and platforms are available for creating prototypes and running simulations.
Example: Before finalizing the design of a house, an architect might build a small-scale model or use software simulations to visualize the layout and identify potential issues with space utilization and design.
The above is the detailed content of Notes for Object Oriented Design | Part-. For more information, please follow other related articles on the PHP Chinese website!