Factory Design Pattern
The Factory design pattern is widely used in object-oriented programming. It provides an interface for creating objects, but allows subclasses to decide which classes to instantiate. In this article, we will explore how to implement the Factory pattern in Golang, understand its benefits and analyze a practical example of use inspired by everyday situations.
What is Factory?
Factory defines an interface for creating objects, but delegates the responsibility for instantiating the concrete class to subclasses. This promotes the creation of objects in a decoupled and flexible way, allowing the code to be more modular and easier to maintain.
Benefits
- Decoupling: Separates the creation of objects from their implementation, promoting cleaner and more modular code.
- Flexibility: Makes it easy to introduce new classes without modifying existing code.
- Maintenance: Makes the code easier to maintain and evolve, as the creation logic is centralized in a single place.
Implementing a Factory
Let's use an everyday example to illustrate the Factory pattern: a system for ordering food, where some different types of meals (Pizza and Salad) can be created.
1 - Creating the interface
First, we need to define an interface that will be implemented by all "concrete classes" of meals.
package main type Food interface { Prepare() }
2 - Creating an ENUM and implementing the interface
To make our lives easier during development and avoid typing something wrong during validation, a good practice is to create an ENUM to have consistency and also make it easier if we want to add new foods in the future
package main type FoodType int const ( PizzaType FoodType = iota SaladType ) type Food interface { Prepare() }
And now let's implement the Food interface. In the example we will just display a message, in real life this is where the object we are working on would be created
package main type FoodType int const ( PizzaType FoodType = iota SaladType ) type Food interface { Prepare() } type Pizza struct{} func (p Pizza) Prepare() { fmt.Println("Preparing a Pizza...") } type Salad struct{} func (s Salad) Prepare() { fmt.Println("Preparing a Salad...") }
3 - Creating the Factory
Now, let's create the factory that will decide which concrete class to instantiate based on the enum it received as a parameter.
package main type FoodFactory struct{} func (f FoodFactory) CreateFood(ft FoodType) Food { switch ft { case PizzaType: return &Pizza{} case SaladType: return &Salad{} default: return nil } }
4 - Using Factory
Finally, we will use the factory to create our food.
package main func main() { kitchen := FoodFactory{} pizza := kitchen.CreateFood(PizzaType) if pizza != nil { pizza.Prepare() } salad := kitchen.CreateFood(SaladType) if salad != nil { salad.Prepare() } }
This will be the result after running our application:
Preparing a Pizza... Preparing a Salad...
Summary of what we did
- Food Interface: Defines the contract that all concrete meals must follow, ensuring that they all implement the Prepare method.
- Enum FoodType: Uses typed constants to represent different types of food, increasing code readability and security.
- Concrete classes (Pizza and Salad): Implement the Food interface and provide their own implementations of the Prepare method.
- FoodFactory: Contains object creation logic. The CreateFood method decides which concrete class to instantiate based on the FoodType enum.
- Main method: Demonstrates the use of the factory to create different objects and call their methods, illustrating the flexibility and decoupling provided by the Factory pattern.
Conclusion
The Factory design pattern is a powerful tool for promoting decoupling and flexibility in object creation. In Golang, the implementation of this pattern is direct and effective, allowing the creation of modular and easy-to-maintain systems. Using interfaces and factories, we can centralize creation logic and simplify code evolution as new requirements emerge.
The above is the detailed content of Factory Design Pattern. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










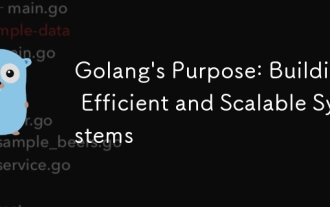
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
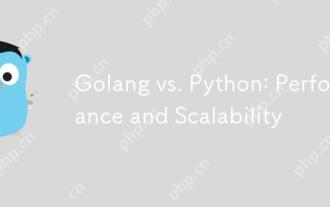
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
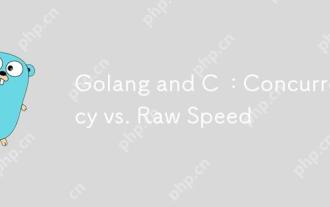
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
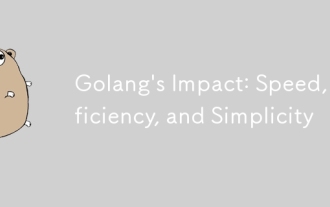
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
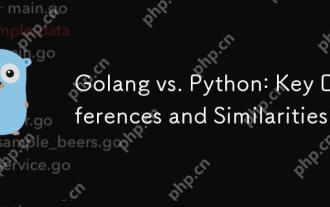
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
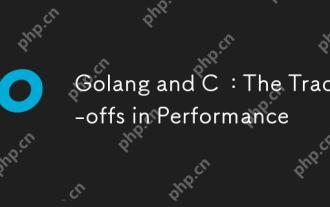
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
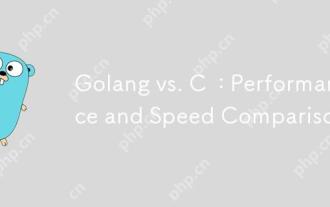
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
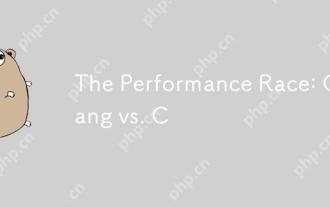
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
