JavaScript to Python for Beginners
Why Learn Python?
Python is one of the most popular programming languages in the world, widely used in various fields such as web development, data analysis, artificial intelligence, scientific computing, and more. It is known for its readability and simplicity, making it an excellent choice for beginners and experienced developers alike. Python's extensive libraries and frameworks such as Django, Flask, Pandas, and TensorFlow, enable developers to build complex applications efficiently.
Essential Syntax: A Quick Overview
1. Data Types
In Python, common data types include integers int, floating-point numbers float, strings str, lists, tuples, sets, and dictionaries.
# Integers and floats x = 10 y = 3.14 # Strings name = "John Doe" # Lists fruits = ["apple", "banana", "cherry"] # Tuples coordinates = (10.0, 20.0) # Sets numbers = {1, 2, 3, 4, 4} # Dictionaries person = {"name": "Luke", "age": 19}
2. Variables
Variables in Python are dynamically typed, meaning you don't need to declare their type explicitly.
# Variables a = 5 b = "Hello, World!"
3. Code Blocks
Python uses indentation to define code blocks instead of curly braces {} like in JavaScript.
# Example of a code block if a > 0: print("a is positive") else: print("a is negative")
4. Functions
Defining functions in Python is straightforward with the def keyword.
# Function definition def greet(name): return f"Hello, {name}!" # Function call print(greet("Bo"))
5. Conditionals
Python uses if, elif, and else for conditional statements.
# Conditional statements if x > 0: print("x is positive") elif x == 0: print("x is zero") else: print("x is negative")
6. Arrays and Objects
In Python, lists and dictionaries are the closest equivalents to JavaScript's arrays and objects.
# Lists (arrays in JavaScript) numbers = [1, 2, 3, 4, 5] # Dictionaries (objects in JavaScript) car = { "brand": "Toyota", "model": "Corolla", "year": 2020 }
7. Iteration
Python provides various ways to iterate over sequences, including for loops and while loops.
# For loop for fruit in fruits: print(fruit) # While loop count = 0 while count < 5: print(count) count += 1
Differences and Similarities Between Python and JavaScript
Differences
1. Syntax: Python uses indentation for code blocks, whereas JavaScript uses curly braces.
2. Data Structures: Python has built-in support for lists, tuples, sets, and dictionaries, while JavaScript primarily uses arrays and objects.
3. Functions: Python functions are defined using def, where JavaScript uses the function keyword or arrow functions =>.
Similarities
1. Dynamic Typing: Both languages are dynamically typed, allowing for flexible and concise code.
2. Interpreted Languages: Both are interpreted languages, making them suitable for scripting and rapid development.
3. High-level Language: Both languages are abstracted from low-level details, enabling developers to focus on solving problems.
Tips for Learning Python as a JavaScript Developer
1. Leverage Your JavaScript Knowledge: Many programming concepts such as variables, loops, and conditionals are similar, so you can focus on Python’s specific syntax and conventions.
2. Practice with Projects: Build projects like a web scraper, a simple web app using Flask, or data analysis scripts to get hands-on experience.
3. Use Interactive Python Environments: Tools like Jupyter Notebook and IPython can be helpful for experimenting with Python code.
4. Explore Python Libraries: Familiarize yourself with popular Python libraries relevant to your interests, such as Django for web development or Pandas for data analysis.
Learning Resources
Official Python Documentation
Real Python Tutorials
W3Schools Python Tutorials
Automate the Boring Stuff with Python
Learning Python can significantly broaden your programming skills and open up new opportunities in various fields of software engineering. With its simplicity and readability, you'll find that transitioning from JavaScript to Python can be a smooth and rewarding experience. Happy building and best of luck!
The above is the detailed content of JavaScript to Python for Beginners. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










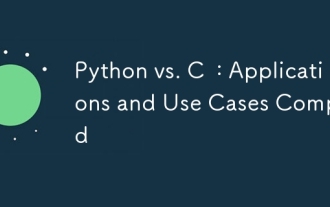
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
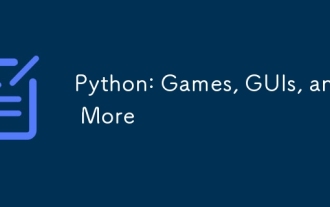
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
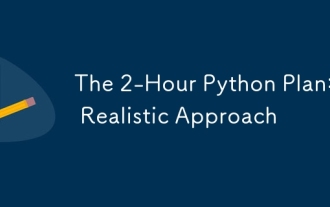
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
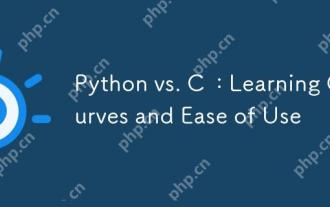
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
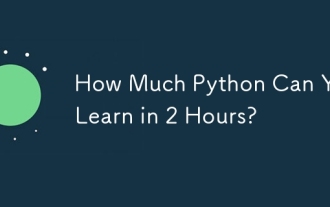
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
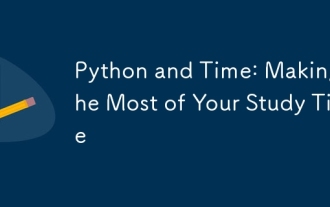
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
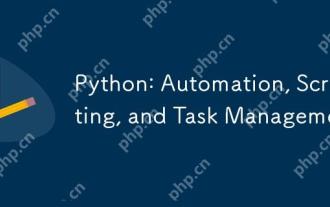
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
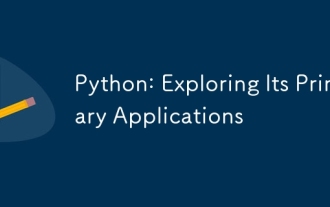
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
