


C# | Building a Command-Line (CLI) App using System.CommandLine Library
Note You can check other posts on my personal website: https://hbolajraf.net
Introduction
In this guide, we will explore how to build a Command-Line Interface (CLI) application using the System.CommandLine library in C# and .NET. System.CommandLine simplifies the process of creating robust and feature-rich command-line interfaces for your applications.
Prerequisites
Before getting started, make sure you have the following installed:
- .NET SDK (version 5.0 or later)
Step 1: Create a new Console Application
dotnet new console -n MyCommandLineApp cd MyCommandLineApp
Step 2: Add System.CommandLine NuGet Package
dotnet add package System.CommandLine --version 2.0.0-beta1.21308.1
Step 3: Define Command-Line Options
In your Program.cs, define the command-line options using System.CommandLine:
using System.CommandLine; using System.CommandLine.Invocation; class Program { static int Main(string[] args) { var rootCommand = new RootCommand { new Option<int>("--number", "An integer option"), new Option<bool>("--flag", "A boolean option"), new Argument<string>("input", "A required input argument") }; rootCommand.Handler = CommandHandler.Create<int, bool, string>((number, flag, input) => { // Your application logic goes here Console.WriteLine($"Number: {number}"); Console.WriteLine($"Flag: {flag}"); Console.WriteLine($"Input: {input}"); }); return rootCommand.Invoke(args); } }
Step 4: Run the CLI App
dotnet run -- --number 42 --flag true "Hello, CLI!"
Replace the values with your own and see the output.
Step 5: Customize Help Text
Add descriptions to your options and arguments for better help text:
var rootCommand = new RootCommand { new Option<int>("--number", "An integer option"), new Option<bool>("--flag", "A boolean option"), new Argument<string>("input", "A required input argument") }; rootCommand.Description = "A simple CLI app"; rootCommand.Handler = CommandHandler.Create<int, bool, string>((number, flag, input) => { Console.WriteLine($"Number: {number}"); Console.WriteLine($"Flag: {flag}"); Console.WriteLine($"Input: {input}"); });
What Next?
You have successfully created a basic Command-Line Interface (CLI) application using the System.CommandLine library in C# and .NET. Customize and extend the application based on your specific requirements.
For more information, refer to the official documentation: System.CommandLine GitHub
The above is the detailed content of C# | Building a Command-Line (CLI) App using System.CommandLine Library. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










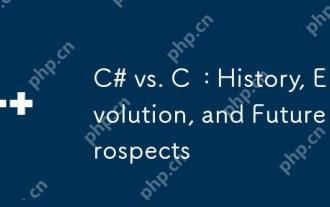
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
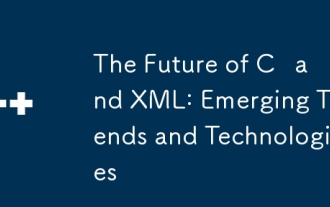
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
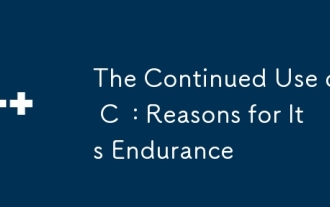
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
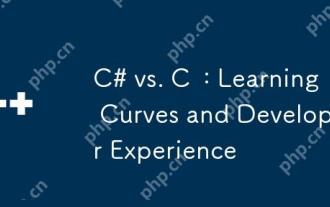
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
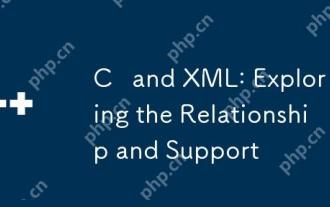
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
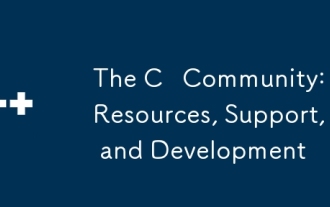
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
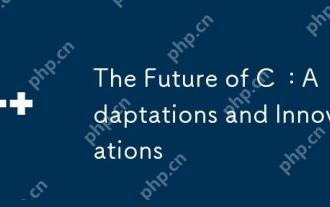
The future of C will focus on parallel computing, security, modularization and AI/machine learning: 1) Parallel computing will be enhanced through features such as coroutines; 2) Security will be improved through stricter type checking and memory management mechanisms; 3) Modulation will simplify code organization and compilation; 4) AI and machine learning will prompt C to adapt to new needs, such as numerical computing and GPU programming support.
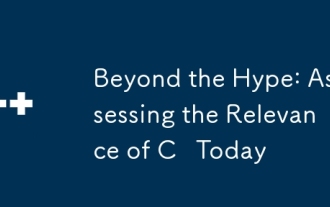
C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
